Welcome to CS21!
About the course
This is our introductory course for Computer Science (CS), so no prior knowledge of CS is required. If you have taken AP Computer Science in high school, or have a fair amount of programming experience (e.g., you understand arrays, strings, searching, sorting, recursion, and classes), please come see us during the first week to make sure you are in the correct class. This class is intended for majors and non-majors, but if you have significant programming experience, we may want to move you up to one of the next courses in CS (CS31 or CS35).
For CS21 we have 3 lecture sections and 6 lab sections, so you might have Lisa for lecture and Charlie for lab, or some other combination:
-
Lecture Section 1 — Lisa Meeden
-
Lecture Section 2 — Aline Normoyle
-
Lecture Section 3 — Jeff Knerr
-
Lab Sections A,B — Charlie Kazer
-
Lab Sections C,D — Aline Normoyle
-
Lab Sections E,F — Sara "Scout" Sinclair
We will also have ninjas helping in the lecture, labs, and study sessions. The CS21 ninjas are students that have already taken the course, and are here to help guide you through the process of learning to program. You may also meet Lauri Courtenay this semester, our Academic Support Coordinator. Lauri manages the ninjas and helps us run the classes and labs.
In this class we will introduce you to basic CS concepts such as algorithms, searching and sorting, analysis of algorithms, and object-oriented programming. We will do this by teaching you how to write computer programs, using the python programming language. Python is an excellent language for first-time programmers (as well as one of the top-3 most-used languages in the world today). It is a clean and simple high-level language (i.e., it is easy to read), which often allows you to focus more quickly on the problem you are trying to solve.
Each week we will learn something new, and then write computer programs (lab assignments) to help reinforce what we just learned. In addition to learning to program, and learning about CS, you will practice and learn problem-solving. Designing and writing a program, as well as getting it to run correctly, involves a lot of problem-solving. Hopefully this is a skill that will help you in life, whether you go on in CS or not.
But CS is not programming! Many computer scientists (not all!) do write programs, but CS is more the study of algorithms and what is/is not possible with algorithms.
An example: maze solving
Instead of trying to tell you exactly what CS is or is not, let’s look at an example.
Suppose you have a robot (or a human) in a maze, and you have to give it instructions on how to traverse the maze from the start to the finish:
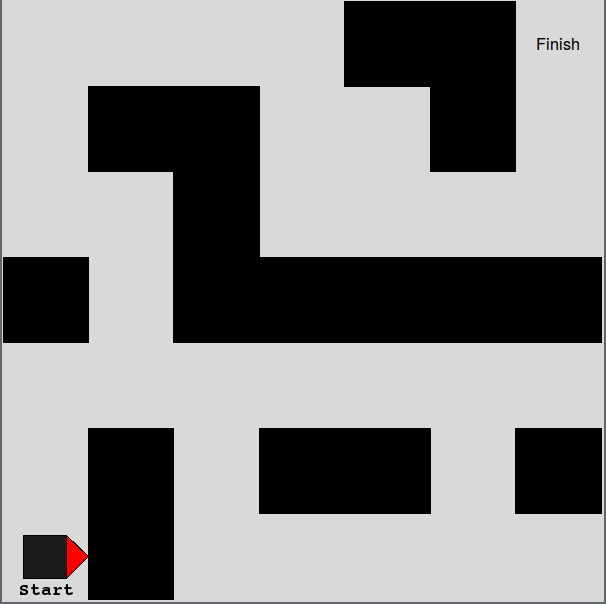
One obvious way, for this particular maze, would be to give it very specific instructions, like this:
-
turn left
-
go forward two steps
-
turn right
-
go forward one step
-
turn left
-
go forward two more steps
-
and so on…
Short aside: one reason we like and are using this maze/robot example is because it is representative of the type of program you should be able to write by the end of this course. During week 6 we will cover graphics, and this animation is made with the same tools we will use in class. Drawing a maze and a simple robot moving through the maze is something you should be able to do by the end of this course.
For this problem, assume that the maze is just an NxN grid (here 7x7), where each grid location is considered one "step" for the robot:
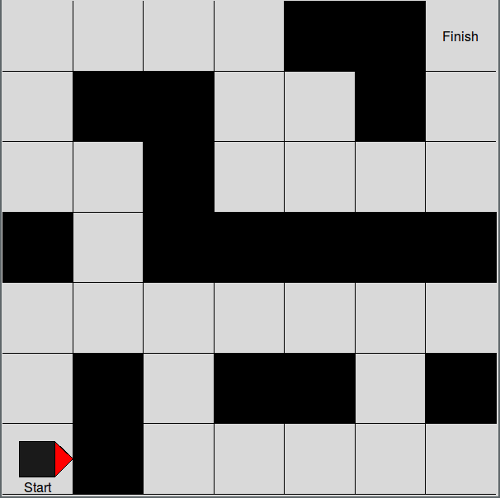
Some grid locations are empty, and the robot can move to/from those locations. Others are walls (the black squares), and the robot can’t move through the walls.
The robot also has a "heading", such as "east" or "south", so it knows which direction it is going.
A more general solution
So far we only have a specific solution for one particular maze.
Can you write a more general solution that would work for all 3 of these mazes?
For this more general solution, let’s use phrases like these:
-
keep going until you hit a wall
-
turn left (or right)
-
if there’s a wall in front of you, do this, otherwise, do that
-
if there’s a wall on your right, do this
-
repeat these steps until ….
Take a few minutes and see if you can write down a solution that works for all three mazes above. We’re serious….get out a pencil and some scratch paper and jot down a solution, then try it out (on paper or just in your head, looking at the 3 mazes), and see if the robot actually would make it to the end of the maze in all cases.
[ pause here for 10 minutes… ]
Algorithms
Even if you didn’t succeed in writing a general solution to the maze problem, we hope you at least tried, and experienced the process of designing an algorithm. An algorithm is just a series of steps to follow, like a recipe.
One algorithm that works for many mazes is called the left-hand rule. If you’re in a maze, at the start, put your left hand on the wall and just move forward, always keeping your left hand on the wall. If you have to turn right or left to keep your left hand on the wall, do that.
Here’s how we might write the left-hand algorithm, using instructions that the robot can understand:
as long as you are NOT at the Finish, repeat these (do one of these): - if there's a wall on your left and it's clear ahead, move forward one step - but if there's a wall on your left and it's not clear ahead, turn right - otherwise, turn left and move forward one step
And here’s where the computer science part comes in. Does this algorithm work for all 3 mazes? Will it work for any maze? Is it the only algorithm that works? If not, is it the best algorithm?
Here’s a video of the left-hand algorithm solving one of the example mazes:
Another working algorithm for mazes is called random: every time you get to a location in the maze that has more than one option, randomly choose a direction and go. For example, if you get to a location where you could keep going forward, or turn right, flip a coin. If the coins ends up heads, keep going forward, otherwise, turn right and go. It can be shown that the random algorithm works. Do you think it will be better or worse than the left-hand rule? And what do we even mean by "better or worse"?
A bit about computer programming
Most computer programs contain the following elements:
-
input and output
-
branching
-
looping
-
data structures
Your algorithm from above probably has some of these elements in it, and the full maze solution program has all of them. For example, the inputs to the program are the maze data (which locations are walls, which locations are open, where is the start, where is the finish). The output of the maze program is the graphical display that shows the robot moving through the maze. As you decide what the robot should do at each point in the maze, you are using a branch statement (e.g., if there’s a wall ahead, turn, otherwise go forward). And we are looping (i.e., repeating some sequence of steps) as long as the robot is not at the Finish. All of the maze data (which locations are walls, which are open), as well as the robot data (where it is in the maze, which direction it is facing) must be kept/stored in some form to be used throughout the program. How and where this data is stored is called the data structure. Finally, to complete the program, all of this is tied together with the above algorithm.
What We Will Do In This Class
The above maze solver is a good example of what we will do in this class. Each week we will have lab assignments, where you must develop an algorithm and implement it in the python programming language. This includes testing your program, to make sure it works, and analyzing your algorithm, to make sure it is efficient.
Your tasks for this week
For this week, you need to do the following:
-
attend your assigned lab session on either Tuesday or Wednesday. Note the E and F lab sections are in Clothier 16 (the basement of Clothier). All the rest (and the lectures) are in SciCenter 256.
-
start working on Lab 0 during your lab session. You are welcome and encouraged to do some of the lab readings ahead of time.
-
please read the entire class web page!
-
set your CS password: see our password self-service site
If you have a Tuesday lab session, you will get your CS account and password during lab. If not, we will hand out the remaining accounts during Wednesday’s class. Even without your CS account and password, you can still start reading the lab and class web page. And feel free to use our password self-service site to reset your CS password before you come to class or lab (click on the Forgotten Password button, enter your username (like hpotter1), click Search, check your ITS email for the Code, then enter the Code and change your CS password). Note: this CS password will only be for the CS computers — it will not affect your ITS account.
Let us know if you have any questions!