Lecture 3/23/2020
important stuff
-
Make sure you can run ssh and Atom with ftp-remote-edit
-
NOTE: Setting up your development environment to run graphics is optional
-
-
TDD designs are due this week. Submit your design to tdd@cs.swarthmore.edu as soon as possible for feedback
Lecture outline
-
Zoom: chat, raising hands, sharing screens, and brakout rooms
-
Recordings are not private, although they can only be viewed by those with a swarthmore.edu account
-
-
Slack: posting in channels and direct messaging
-
TDD Review
-
What is TDD?
-
What are the steps of creating a TDD?
-
What are the requirements of a good TDD design?
-
-
Review: saucer.py - the solution is available if you run update21
-
Exercise: fitness.py (Part 1) - try to write a TDD for fitness.py (a toy program with only 1 function)
Lecture 3/25/2020
Agenda
-
Exercise: fitness.py
-
Topic: Files and parsing
-
Demo: shopping.py
-
-
Exercise: average.py
-
Exercise: averages.py
fitness.py
Top-down design:
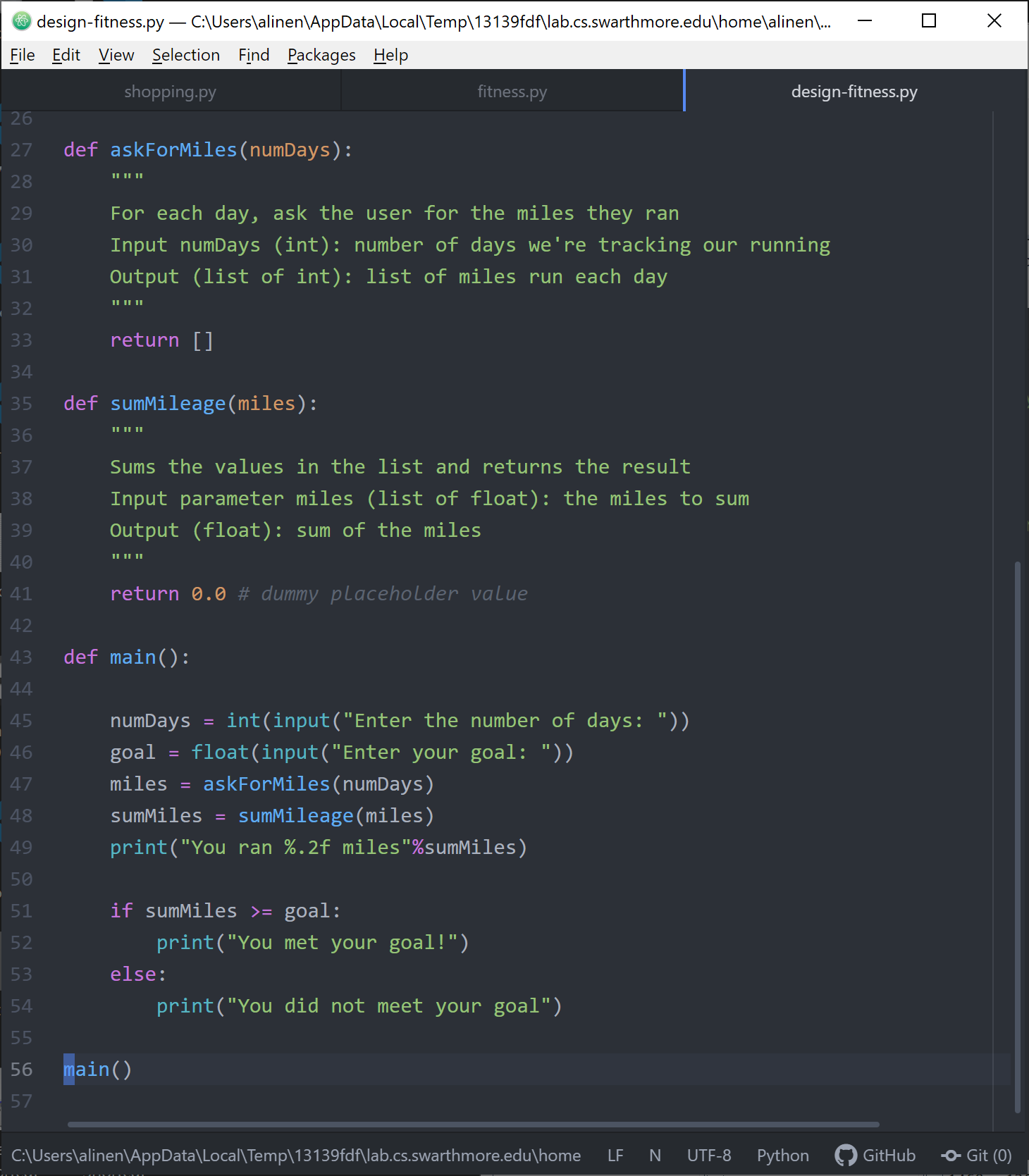
Finished implementation:
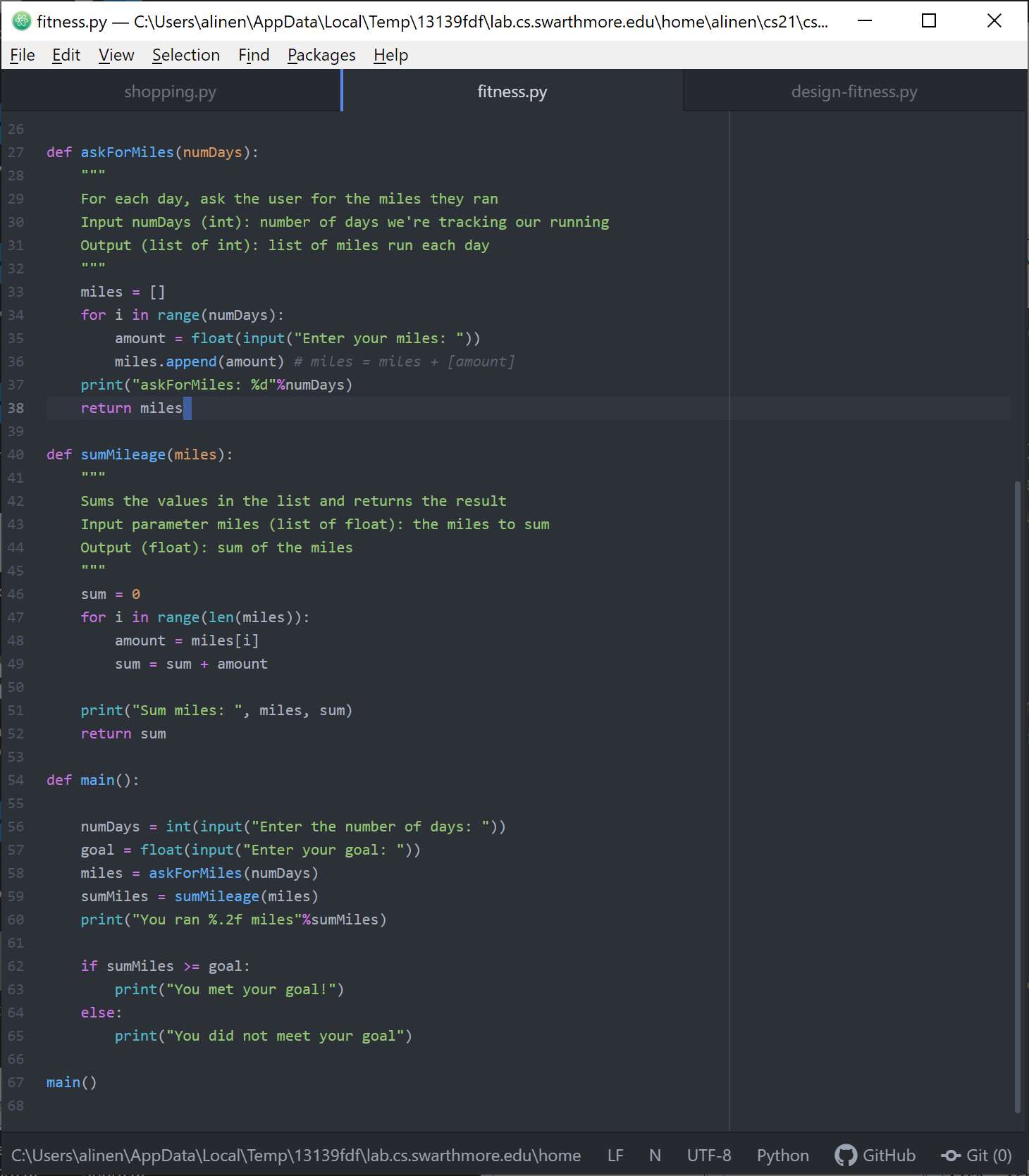
Working with files
A file allows us to store data persistently on a computer. Using files, we can write programs which process large amounts of data without having the enter the data by hand.
A text file contains a sequence of characters. Examples are our Python programs, html files, or notes we might save with Atom.
A binary file contains raw bytes of data. These data can represent anything. Examples of binary files are wav files (music and sounds), png files (images), or mpg files (videos). Binary files can store information more compactly, e.g. using less space, than text files.
To read a file in Python:
-
Open the file
-
Read through each line
-
Close the file. If we do not close the file, Python will close it after the program exits.
Example
def main():
# Passing "r" opens the file for reading
file = open("shoppingList.txt", "r")
for line in file:
print(line)
file.close()
main()
To write a file in Python:
-
Open the file
-
Write lines
-
Close the file
Example
def main():
# Passing "w" opens the file for writing
# Watch out! This will overwrite the file's contents!
file = open("test.txt", "w")
for i in range(10):
line = f"Hello {i}\n" # Need \n for newline!
file.writelines(line)
file.close()
main()
Parsing
When we read in a text file, we receive each line as a string. Often this string must be converted into values that we can use in our program. This process is called parsing.
When parsing, we need to watch out for whitespace characters.
The \n
character represents a new line. The \t
represents a tab.
Use strip()
to remove whitespace from the beginning and end of a
string.
When parsing, we often need to split a string into sub-strings based
on a deliminator character. Use split(<character>)
to split a
string based on <character>. The sub-strings we acquire from splitting
are often called tokens. The process of splitting strings is
called tokenization.
shopping.py
This example loads a list of items from a file and strips whitespace
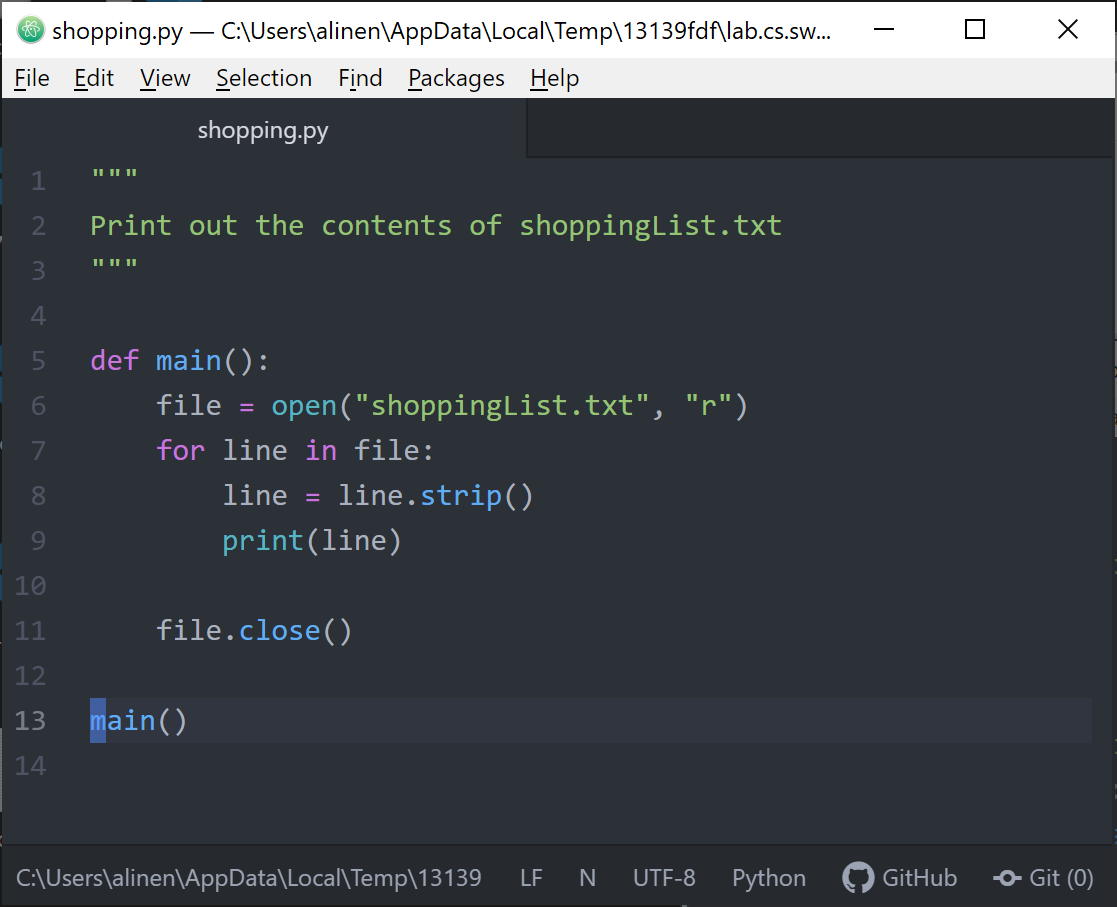
This example loads a list of items and quantities from a file and converts the input to string and integer values.
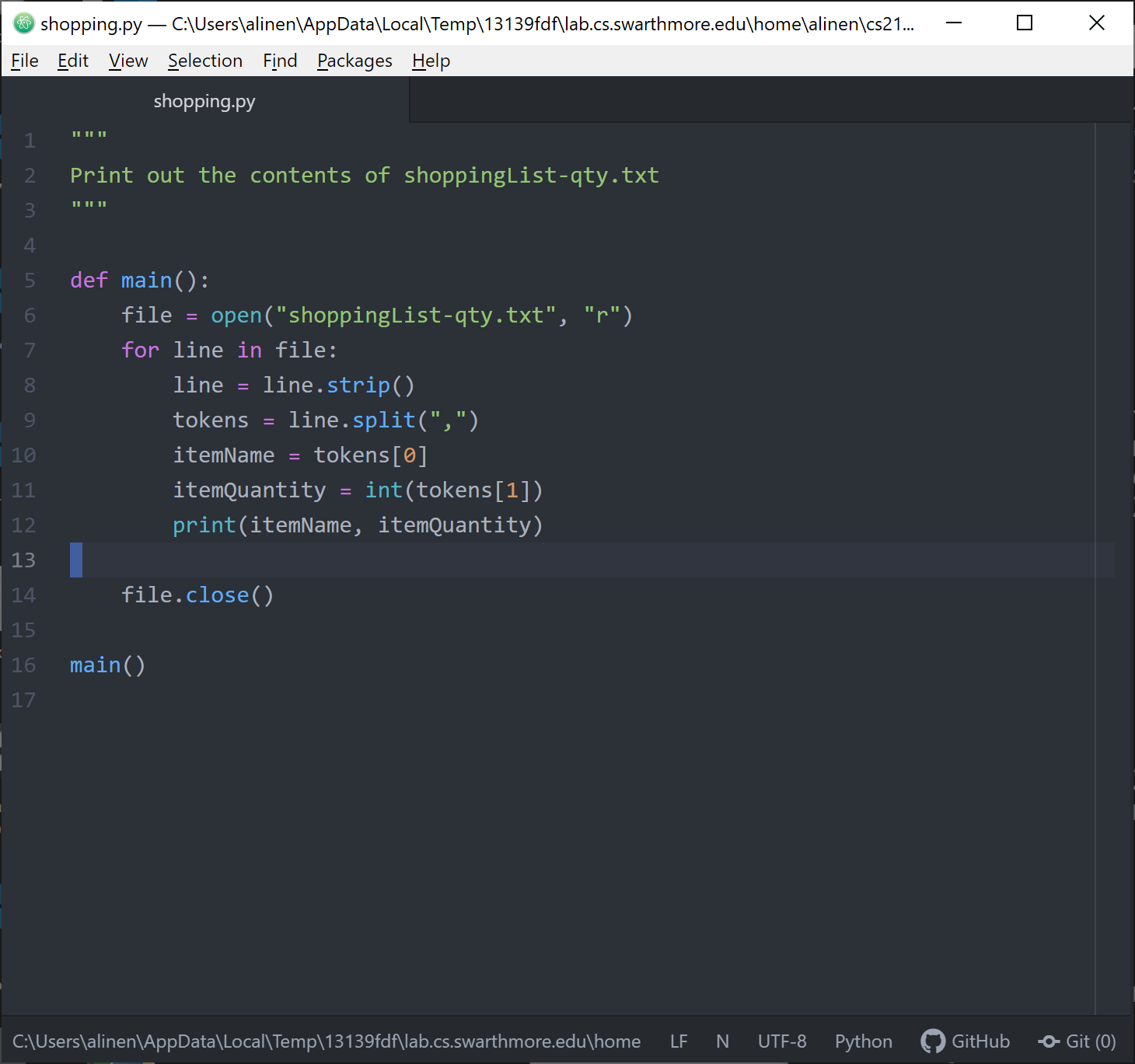
average.py
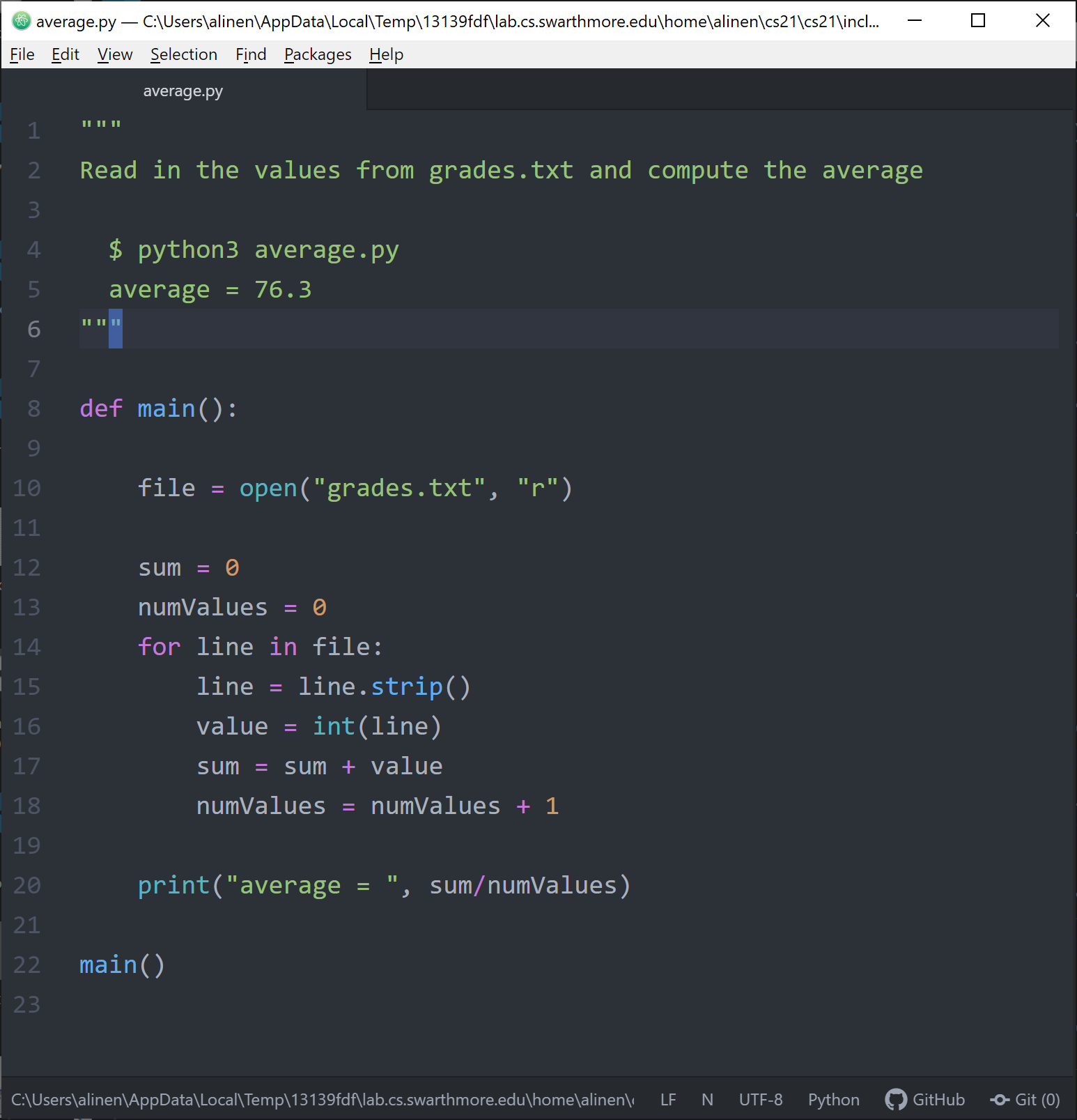
averages.py
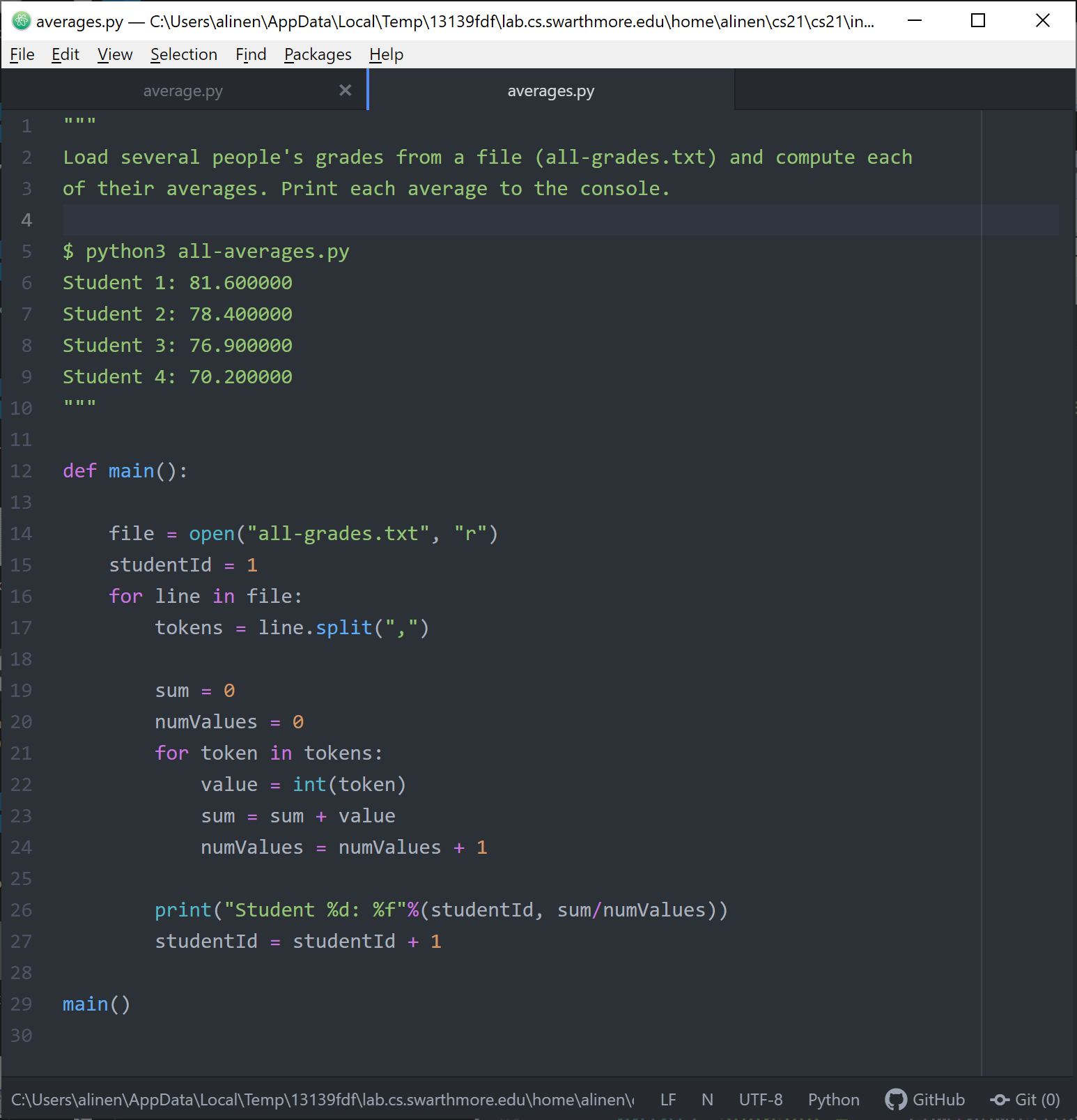
3/27/2020
Agenda - Review: files and parsing - Exercise: flashcards.py — design: from feature 'verbs' to functions — design: implementing main() and choosing function parameters and return values
flashcards
You should have two designs in your /inclass directory:
-
design-flashcards.py
is the solution from lecture. This solution was modified so that our stub for menu() contains placeholder code to ask the user for an option. This allows us to thatlistCards
andpracticeCards
are called. -
design-flashcards-soln2.py
is an alternate design which defines a function calledaskCard
. Both designs are equally valid.