Lab description
This lab assignment requires you to write two programs in
Python. First, run update21. This will create the
cs21/labs/05 directory and copy
over any starting-point files for your programs.
Next, move into your cs21/labs/05 directory and begin
working on the Python programs for this lab.
The pwd command
helps you verify that you are in the correct sub-directory.
$ update21
$ cd cs21/labs/05
$ pwd
/home/your_user_name/cs21/labs/05
We will only grade files submitted by
handin21 in this
directory, so make sure your programs are in this directory!
Programming tips
For each program you should:
- Use a comment at the top of the file to describe the purpose of
the program.
- Use variable names that describe the contents of the variables.
- Use def main() to combine the steps of your program into
a function.
- Place imports, like those shown below, after
your top comment and before def main()
from graphics import *
from random import *
- Write your programs incrementally and test them
as you go.
Zelle Graphics Reference
1. Draw an animal face
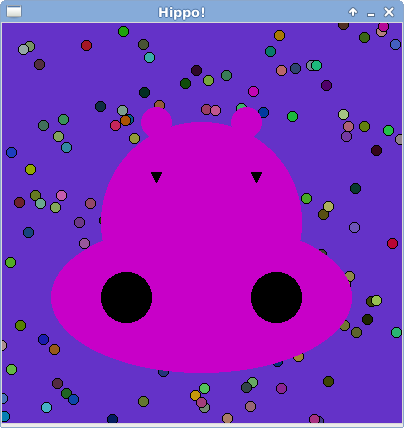
Open the file drawFace.py and create a program that draws
a face using the graphics library. Above are two examples of animal
faces created by previous cs21 students.
You should follow these guidelines when creating this program:
- The face should be centered in the graphics window, and largely
fill the space.
- Your program should keep the drawn face on the graphics window
until the user clicks the mouse. Then the graphics window should close
and your program should end.
- You should base the positions and sizes of the various facial
features on one or more of the following:
- center point of the window
- width and height of the window (you can use the
methods getWidth() and getHeight() on
a GraphWin object)
- size of the head
- Do not hard code locations. Your face should draw correctly in
different sized windows. Be sure to test this. You can assume that the
window will always be square (i.e. that the width will equal the
height).
- Use color and several different types of shapes.
For symmetrical features (such as eyes or ears), you can use
the clone method to make a copy of the original shape. Then
draw the cloned shape in the window and move it to the desired
location.
Have fun! Your animal face doesn't have to be as elaborate as the
above examples.
2. Draw a spring meadow
Spring is just around the corner, and in anticipation we will
create an interactive program to make a meadow of similar, but unique
flowers. Using mouse clicks, your program will allow the user to
specify the location and height of as many flowers as they'd like.
When they are done making flowers they can quit the program by
clicking on the black border at the bottom of the window.
Open the program drawFlowers.py and work incrementally toward
drawing a picture like the one above.
- Begin by importing the graphics library after your opening
comment and before the main program.
- In the main program create a graphics window and set
the background of the window to a sky-like color. Also use
the getMouse() method of the window to wait until the
user clicks before ending the program. Test your program.
- Create a function makeGrass(window) that takes the
graphics window and makes a rectangle that is half the size of the
window, is located in the bottom half of the window, and is
colored green. Call the function in the main program to
test that it works.
- Create a function makeBorder(window) that takes the
graphics window and makes a rectangle that is 5% of the window's
height, is colored black and is positioned at the very bottom of
the window. Call the function in the main program to
test that it works.
- Add a while loop to the main program so that
it continues to accept mouse clicks until the user clicks in the black
border area. You'll know this has happened when the y-component of
the clicked point is greater than 95% of the window's height. Test
that this works.
- Create a function makeFlower(window, click1) that
takes the graphics window and a point generated from a mouse
click. This point represents the location of the center of the
bulb of the flower (click 1 in the picture below). This
function should draw a circle to represent the bulb that has a
radius which is 5% of the window's width. This function should
then get a second mouse click (click 2 in the picture
below), representing the end of the stem. It should draw a line
between click1 and click2. Don't worry about
adding colors now, just be sure you can make this skeleton
of a flower first. Call this function inside the main
program's loop.
- Edit your makeFlower function to make the bulb of the
flower a random color. Add a smaller inner circle to the flower
that is also a random color. Make the stem thicker and add color
if you'd like. Test your program to check that you are getting
random colors each time.
- The graphics library layers objects in the order they are
drawn. While drawing the flower, we want the user to see the bulb
of the flower appear as soon as they do the first click, so we
need to draw this first. Then when we want the stem to appear as
soon as they make the second click. Thus the stem will be drawn
on top of the bulb, which doesn't look good. To make our flower
look nicer, after we've drawn the stem, we can
call undraw() on the bulb, and then
call draw(window) on the bulb again to place it on top of
the stem. Test the program after this change.
- Edit your makeFlower function to add the leaves. Use
the getCenter() method to find the center point of the
stem. Make two symmetrical Oval objects, one above the
center point, and one below. Ovals are defined by a bounding
rectangle based on two points. Each of your leaves will use the
stem's center point as one of these points. For the left leaf,
create another point that is above and to the left of the stem's
center point. For the right leaf, create another point that is
below and to the right of the stem's center point. Test that your
program creates the leaves correctly.
Once you've completed these steps, your program should be drawing a
lovely meadow of flowers. Show it to your friends, and give them a
taste of spring too.
Extra Challenge: Animation
This is completely optional. Only attempt this once you have
completed the required portions of this lab.
Create a file called animation.py. In this file create
some kind of animation using the graphics library. Animations are
created by repeatedly moving objects in the graphics window combined
with a short delay (using sleep from the time
library) between movements.
Tip: If you'd like to make a complicated picture move, it is
helpful to put all of its parts (the various objects) into a single
list. Then you can move the entire picture using a for
loop that moves each individual part the same amount. For example,
you could copy your animal face into this program, putting the head,
eyes, ears, mouth, etc. into a single list. Then you could animate
the face.
Submit
Remember you may run handin21 as many times as you like. Each
time you run it new versions of your files will be submitted. Running
handin21 after you finish a program, after any major changes
are made, and at the end of the day (before you log out) is a good
habit to get into.