Socket Programming
For this reference page, we refer to the |
As we’ll see in class, a protocol defines both the message & header format and transfer procedure. With HTTP, your lab code must first establish a connection before it starts sending and receiving data.
To establish this connection, the application layer (your lab1 web client), relies on a transport layer protocol known as TCP. TCP offers in-order, reliable delivery, making it a common transport protocol choice. To start a TCP connection, your client will create a socket. You can think of a socket like a mailbox to send and receive messages.
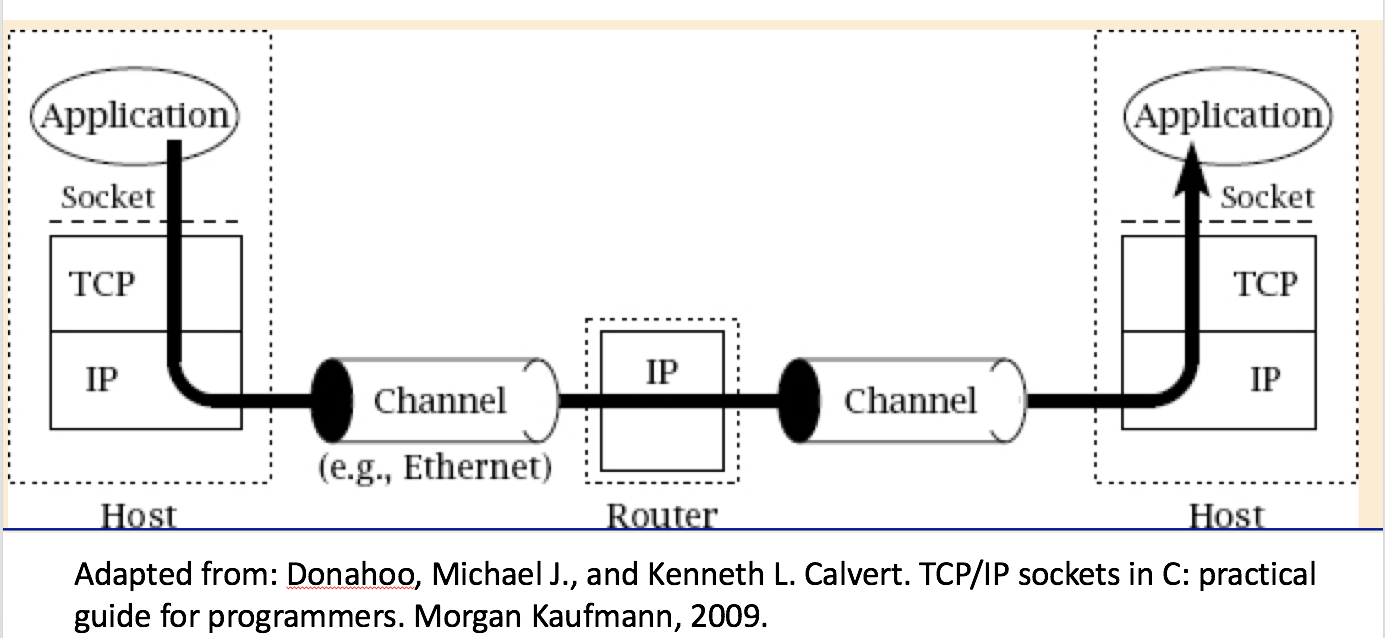
Socket system-calls
First you need to create a socket on the client side. Once you have a socket,
you’ll need to connect it to the server, which requires you to specify the
server’s address using a struct sockaddr_in
(details in the next section).
After your socket is connected, you can send and receive data. Eventually,
you’ll need to close the socket, after you’re done using it.
Look through the system calls provided in lab1.c and their comments.
|
Resolving a hostname to an IP address
Before you can send an HTTP GET message to the server, you need to translate
the destination’s hostname (demo.cs.swarthmore.edu
) to its corresponding IP
address. Fortunately, there’s a system that will do this for you: the domain
name system (DNS). We’ll study DNS in much more detail later, so we’re going
to treat it like a black box that magically converts hostnames to IP address in
lab 1. For now, you need to know that there are two functions that will
translate hostnames to IP address:
-
The
gethostbyname
function is the old interface. You shouldn’t use it for this lab, but you should know of its existence, as you may encounter it some day — it’ll probably never die out for good. I’ve provided an example ingethostbyname.c
. You may want to look over it briefly, but don’t spend too much time on it. -
The
getaddrinfo
function is the preferred function for new code. To use it, you’ll pass in the hostname, and it will fill in a pointer to astruct addrinfo
struct. On failure, that pointer will be NULL, and you shouldn’t attempt to use it. On success, it’ll point to a struct that contains the answer you’re looking for. After you’re done using it, you should free the struct withfreeaddrinfo
. See thegetaddrinfo.c
example for details.