This is an individual lab assignment. The next four labs and the midterm will be partner assignments. In this lab you will practice working wit git, CMake, Qt, and C++ to implement some basic image processing filters. After this lab, you'll just be a few steps away from the next Snapchat/Instagram.
Lab 1 goals
- Gain proficiency using the development tools for this course
- Manipulate images at the pixel level
- Understand the RGB color space
- Learn to navigate the Qt5 Documentation
- Make ponies look fancy
Useful references
Getting started
Initializing git repos
Clone your personal copy of the starter code for lab 01.
$ cd
[~]$ ssh-add
Enter passphrase for /home/ghopper1/.ssh/id_rsa:
Identity added: /home/ghopper1/.ssh/id_rsa (/home/ghopper1/.ssh/id_rsa)
[~]$ cd cs40/
[cs40]$ mkdir labs
[cs40]$ cd labs/
[labs]$ git clone git@github.swarthmore.edu:CS40-F16/lab01-YOURUSERNAME.git 01
Making and building code
Make a build directory in your projects directory and run cmake, and make. You can also do this in QtCreator by selecting new project and opening the file
~/cs40/projects/CMakeLists.txt
[01]$ cd ~/cs40/labs/01
[01]$ mkdir build
[01]$ cd build
[build]$ cmake ..
[build]$ make -j8
Working with RGB components
OK, you are now ready to modify the code to complete the lab.
Your primary goal is to write code to split an image into four sub-images showing the red, green, blue, and grey components separately. The grey component is computed by taking the average of the
r,g,b components for a given pixel. An example is shown below
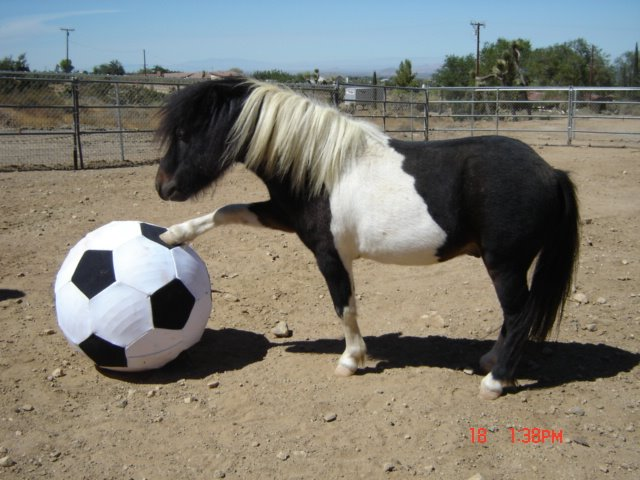
img found here.
Nothing too tricky here, but note that each sub-image is 1/4 the size of the original. You can decide how to sample pixels in the original image to create the sub-image. One possibility is to take the upper left pixel of each 2x2 block of four pixels in the original image.
Modifying Code
Implement your functionality as a QT application. Start with the code in
lab/01, and design at least the UI part in
qtcreator. The project should build in either qtcreator or standard CMake. Much, but not all of the UI design has been completed already.
If you run ./lab1 you'll see the load and quit buttons work. The Split button has signal and slots connected and will pop up a window saying this feature is not yet implemented. The New Effect button, which can be a new image effect of your own choosing is not connected to a MyImageBox slot. You will need to edit slots/signals for this button in the UI designer and then implement the desired behavior in the MyImageBox class
Implementing Split
You should implement split in
myimagebox.cpp. Look for the function stub
void MyImageBox::split() {
/* http://doc.qt.io/qt-5/qmessagebox.html#information */
QMessageBox::information((QWidget*)parent(),"Oops!", "This is not implemented ");
}
Remove the stub code and write code to create a new
QImage object,
newImage that splits the current image
m_current. At the end of processing, set the current image to be your new image, and update the widget to show the new image.
m_current=outImg;
m_imageLabel->setPixmap(QPixmap::fromImage(m_current));
To help implement your split method, you may want to look at the following
QImage and
QColor methods. Note that the file
testQImage.cpp shows how to use some of these methods to copy a sample image file. You may want to edit this file to do some simple tests on the
QColor class before integrating your solution into
myimagebox.cpp. Nothing in
testQImage.cpp will be graded.
QImage
QColor
Note that QImage mentions methods
pixelColor() and
setPixelColor(), but "
[These] function[s] [were] introduced in Qt 5.6". We have Qt5.5 installed, so you'll have to use
pixel() and
setPixel(),
When testing your code, be sure to consider the following cases
- What if the user does not load an image before clicking Split? You should detect this case and display a QMessageBox
- What happens if you click split twice?
Adding a new effect
To add a new effect, edit the
mainwindow.ui file in the Designer window of QtCreator.
-
Change the name of the button label to something more descriptive.
- Create an event that connects the clicked() signal on the new button to a new slot in the MyImageBox widget. You may need to add a slot in the Designer window and give the new slot a descriptive name for your effect.
- Add the new slot method name to the MyImageBox header
- Implement your new effect similar to the way you implemented split()
- If no image is loaded when the button is clicked, display an informational QMessageBox.
If you would like to add more effects, you will need to add more buttons and slots.
Hints and Tips
- Start early, proceed incrementally
- Commit and push when something new is working
- You may want to make a QString imgName private member variable to remember the name of the last opened image.
- Use the QT Documentation. Remember, some methods might be defined in a base class.
- The only source files you really need to change are myimagebox.*
- Aside from QColor and perhaps QString, all other QT classes you need are used at least once in myimagebox.cpp
Submit
Prior to submitting your code, edit the
README.md file and answer the Concept Questions and the Quick Lab Survey. Add, commit, and push your answers to these questions along with your source
To submit your code, simply commit your changes locally using
git add and
git commit. Then run
git push while in the
labs/01 directory.
Summary of Requirements
Your project will be graded on the following components
- A working split function plus one additional image processing effect
- Suitable error handling for when a user clicks a button and an image is not loaded
- Easy to read code. New methods should have a descriptive comment.
- Answers to the concept questions in the README.md. You will not be graded on the Lab Survey questions in that file.
- A small percentage of your grade will be based on style, creativity, and your new effect. A simple convert to grey scale image or a snarky make blank effect meets the letter of the requirements, but not the spirit. Have fun and try something a little more elaborate.
Optional Extensions
These extensions are entirely optional and should not be attempted until the required components are complete.
- Add more image effects for manipulating RGBImages. Examples might include blur or posterize.
- You can add images to your data folder and commit them to git if you have a favorite sample image. Don't go crazy though. Two to three examples max, please.
- Use a QVector<QImage> of QImage objects and implement an undo button that can undo multiple filters