You should save your program for this assignment in
cs21/homework/09. A skeleton version of the program will
appear in this directory when you run update21 in a terminal
window. The program handin21 will only submit files in this
directory.
Some of the problems will have optional components that allow you to
further practice your skills in Python. Optional portions will not be
graded, but may be interesting for those wanting some extra challenges.
Turtles
For this assignment you will write a program that implements a class of turtle
objects. These are no ordinary turtles however as these turtles have a penchant
for both the fine arts and computer science. These are turtles that can draw!
Imagine placing a turtle on a canvas with a pen attached to his tail. Our
turtle can move forward, turn clockwise or counter-clockwise, and raise or
lower its tail. If the turtle moves while its tail is down, the pen leaves a
trail behind the path of the turtle. You will write a class that allows you to
create and manipulate drawing turtles.
A Turtle Class
The current state of the turtle can be described by the following variables:
- xPos - The horizontal location of the turtle
- yPos - The vertical location of the turtle
- direction - The direction the turtle is facing, measured in degrees counter-clockwise from East
- tailDown - A Boolean flag that is True if the turtle's tail is lowered
To change or query the turtle's state, you can use a number of turtle methods
- moveAmt(dist) - Move the turtle forward in the direction the turtle is currently facing by an amount dist
- moveTo(x,y) - Move from the current location to the point (x, y) in a straight line
- turn(angle) - Turn the turtle counter-clockwise by angle degrees from the current direction. If the turtle is facing North, then turn(90) would make the turtle face West while turn(-90) from North would make the turtle face East
- up() - Raise the turtle's tail and stop drawing
- down() - Lower the turtle's tail and start drawing
- isDown() - Returns True if the tail of the turtle is currently down.
Turtle objects exist in a graphics window, whose center has the coordinates
(0,0). When the turtle moves with its tail lowered, a line is drawn in the graphics window that tracks the turtle's movement. An example drawing is shown below for a turtle that initially starts facing North in the center of a
200x200 window.
trevor = Turtle(200, 200)
#A triangle
for i in range(3):
trevor.moveAmt(50)
trevor.turn(120)
trevor.up()
trevor.moveTo(-80,-80)
trevor.down()
#A square
for i in range(4):
trevor.moveAmt(40)
trevor.turn(-90)
raw_input("Press enter to quit")
The turtle constructor takes two parameters indicating the height and width of the turtle graphics window. The constructor should first create a new
GraphWin object using the
graphics class. The center of the graphics window should be
(0,0) meaning that the lower left and upper right corners of the window should have coordinates
(-width/2, -height/2) and
(width/2, height/2), respectively. You can use the
setCoords method on
GraphWin objects to set up this coordinate system. The constructor should place the turtle in the center of the window facing North with the turtle's tail down (in drawing mode).
Requirements
Edit the file
turtle.py in your
homework/09 folder to implement the class Turtle as outlined above.
Moving in a Given Direction
There is one small tricky trigonometry part in this assignment. In
moveAmt(dist) you must move the turtle from the current location
(x1,y1) to a new location
(x2, y2) by moving an amount
dist in the current direction the turtle is facing. If the current turtle direction is
t, then the location of
(x2, y2) is given by the following equations:
x2 = x1 + dist*cos(t)
y2 = y1 + dist*sin(t)
where
t is measured in radians. To convert from degrees to radians, use
rad = deg*pi/180
or use the
radians function in the
math library (
from
math import radians).
Optional Components
You may include any additional methods in your class. Some of these methods can make writing other methods easier or extend the feature of you class.
As noted above, these questions are
NOT required to receive full credit. Furthermore, do not attempt to solve these problems until the required portion of the assignment is complete.
There are many extensions you could add to this program. Here are a few
we thought might be interesting.
-
Add methods that can change the color of the turtle's path, change the width of the line drawn, or change the background color of the canvas.
-
Draw the actual turtle on the screen to show its position and orientation.
Rotating the turtle can be rather tricky, but if you use methods similar to the
rotateBug and animateBug from homework
4, it can be done.
- Simulate a random walk by having the turtle repeatedly turning in a random direction and moving a small distance. An example random walk may look like this:
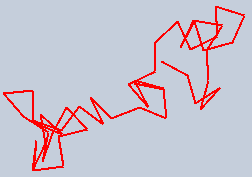
- The textbook gives an example on page 465-466 of a fractal called the "Koch curve" that can be drawn recursively. Write a function that can draw such a curve. By putting together three copies of the curve, you can create the snowflake shown below:
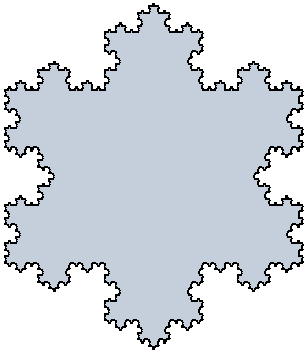
- Similar recursive techniques can be used to draw trees, ferns, and other patterns. The tree below is drawn by drawing the trunk, turning slightly to the left and recursively drawing a tree, then turning slightly right and recursively drawing a tree. Experiment with recursive drawing and come up with your own patterns.
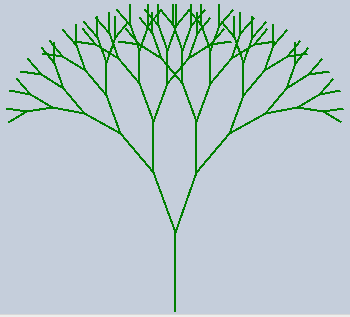
Submit
Once you are satisfied with your program, hand it in by typing
handin21 in a terminal window.