CS21 Lab 4: functions and while loops
Due Saturday, October 5, before midnight
Goals
-
Write programs with multiple functions.
-
Solve problems using indefinite
while
loops. -
Learn to use pseudo-random numbers.
1. Workout Of the Day (WOD)
Note: no while
loop in this one — just a function and main()
to
write.
Don’t know what to do when you get to the matchbox? Tired of thinking up new and different workouts? My kids gave me these cool dice to help with this problem.
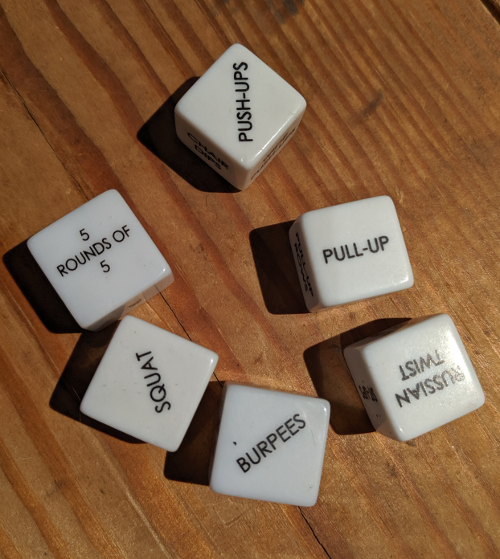
Let’s write a computer program (instead of using the dice) to plan our workouts for the whole week!
1.1. write the wod()
function
In a file called wod.py
, start by writing a wod()
function.
For now, just write a simple main()
that calls wod()
, like this:
def main():
wod()
Your wod()
function is going to pick workout exercises from
a list of options. For example, suppose you have
this list (which you can copy) in your function:
exercises = ["burpees","pushups","box jumps","deadlifts",
"pullups","situps","squats","curls","toes to bar"]
Your function will pick 4 exercises, and for each exercise, it will also pick how many of that exercise to do.
Here’s a quick look at how this function should print the workout (and
it just prints — the function doesn’t return anything to main()
):
1. 14 situps
2. 11 burpees
3. 12 curls
4. 11 box jumps
Use the functions in the random
library (see below) to pick
the exercise from the list and to pick how many of that exercise.
For how many, pick a number from 8-15 (including 15).
1.1.1. some random
hints
If you haven’t seen all of these in class, here are some functions
from random
that might be useful:
>>> from random import *
>>> randrange(1,100)
95
>>> randrange(1,100)
7
>>> randrange(1,100)
78
>>> random()
0.4443630748051812
>>> random()
0.7370533782998238
>>> random()
0.42552976284970034
>>> L = list("ABCDEFG")
>>> print(L)
['A', 'B', 'C', 'D', 'E', 'F', 'G']
>>> choice(L)
'D'
>>> choice(L)
'A'
>>> choice(L)
'A'
If you run your program multiple times, you should see different workouts each time:
$ python3 wod.py
1. 13 situps
2. 11 deadlifts
3. 9 box jumps
4. 11 box jumps
$ python3 wod.py
1. 8 squats
2. 10 toes to bar
3. 13 box jumps
4. 15 situps
$ python3 wod.py
1. 14 pushups
2. 11 box jumps
3. 15 pullups
4. 10 deadlifts
And it’s OK (but maybe not fun) if the program chooses the same exercise over and over!
Finally, make sure your wod()
function has a comment at the top, like this:
def wod():
"""comment here should say what the function does..."""
1.2. finish main()
Once you have the wod()
function working, write a main()
function
that calls wod()
multiple times and generates workouts for the entire
week, starting on October 6. Your program should include a 50-50
chance of either being a workout day or a REST DAY. If it is a workout
day, we’ll do 5 rounds of whatever the wod()
function prints.
Here’s a sample run of the final program (and if you run it again, you will get different workouts and different REST DAYs):
Here's your training week:
= = = = = = = = = = = = = = = = = = = =
October 6, 2019: workout day...5 rounds of:
1. 13 deadlifts
2. 12 pullups
3. 8 squats
4. 11 curls
= = = = = = = = = = = = = = = = = = = =
October 7, 2019: REST DAY!!
= = = = = = = = = = = = = = = = = = = =
October 8, 2019: workout day...5 rounds of:
1. 15 squats
2. 9 situps
3. 9 situps
4. 14 burpees
= = = = = = = = = = = = = = = = = = = =
October 9, 2019: REST DAY!!
= = = = = = = = = = = = = = = = = = = =
October 10, 2019: REST DAY!!
= = = = = = = = = = = = = = = = = = = =
October 11, 2019: workout day...5 rounds of:
1. 8 curls
2. 11 burpees
3. 11 burpees
4. 13 squats
= = = = = = = = = = = = = = = = = = = =
October 12, 2019: workout day...5 rounds of:
1. 14 situps
2. 11 burpees
3. 12 curls
4. 11 box jumps
= = = = = = = = = = = = = = = = = = = =
2. three-card monte
The typical three-card monte game has the user trying to guess where the Queen of Hearts is. And after each guess, the cards are shuffled and the user then makes another guess.
For this program, we’ll just have the user guess based on position, either A, B, or C (where the cards would normally be facing down):
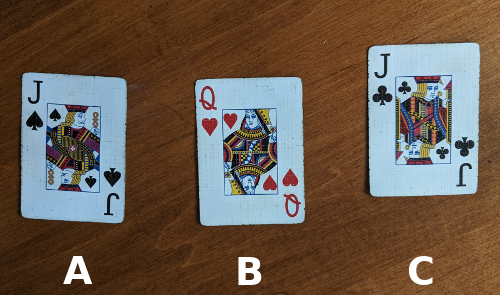
Here’s what our game will eventually look like (but please read the sections below and write the functions we want!):
$ python3 threecard.py
Round 1...
Where is the Queen of Hearts? [A,B,C]: A
Nope....it was in position C!
==============================
Round 2...
Where is the Queen of Hearts? [A,B,C]: A
Nope....it was in position B!
==============================
Round 3...
Where is the Queen of Hearts? [A,B,C]: C
Nope....it was in position B!
==============================
Round 4...
Where is the Queen of Hearts? [A,B,C]: B
Nope....it was in position C!
==============================
Round 5...
Where is the Queen of Hearts? [A,B,C]: A
Correct!
2.1. write the getPick()
function
In a file called threecard.py
, start by writing a getPick()
function
that asks the user to make their pick (the "Where is the Queen…"
line). This function should only return one of three strings: "A", "B",
or "C". This function should only return one of those three strings if
it receives valid input from the user. If the user types something
invalid, the function should ask again (and again, and again) until it
gets a valid pick.
While writing and testing this function, just put a simple call to
getPick()
in main()
, like this:
def main():
pick = getPick()
print("You chose: %s" % (pick))
Here’s an example of how the function handles invalid input (prints informative message, asks again):
Where is the Queen of Hearts? [A,B,C]: aaaa
Please enter A, B, or C!!
Where is the Queen of Hearts? [A,B,C]: zebra
Please enter A, B, or C!!
Where is the Queen of Hearts? [A,B,C]: 1
Please enter A, B, or C!!
Where is the Queen of Hearts? [A,B,C]: A
2.2. finish main()
Once you have getPick()
written and working, go ahead and write the
rest of the game in main()
.
Requirements for the game include:
-
keeps going until the user guesses correctly
-
should keep track of and show how many rounds it takes
-
if the user guesses incorrectly, program should tell the user what the correct position was for that round
Hint: for this game, you don’t need to keep track of all three cards.
We only care about where the Queen of Hearts is, so you can use the
random
library’s choice()
function to pick the Queen’s location
(A, B, or C) for each round.
3. simplified blackjack
The card game blackjack involves dealing cards and trying to get as close as possible to 21 points, without going over.
The initial deal is just two cards, where each card has a numerical point value. Face cards (Jack, Queen, and King) are worth 10 points, all other cards are worth their rank (e.g., 8 of anything is worth 8 points). So, for example, if your first two cards are a 6 and a 10, you’ve got 16 points.
At this point in the game you decide if you want another card, and keep going until you either stop (don’t want any more cards), or go over 21 (you lose).
Here are some sample "games":
First two cards | other cards | result |
---|---|---|
6 10 |
no |
16 |
2 3 |
5 10 |
20 |
8 7 |
10 |
25 (lose) |
8 7 |
6 |
21 |
3.1. write the oneHand(limit)
function
In a file called blackjack.py
, start by writing a oneHand(limit)
function that does a very simple simulation
of a blackjack deal: just one player and just one hand.
In the real game Aces can be either 1 or 11 points, but for
this lab we will make them just 1 point.
Note: you don’t need to simulate actual playing cards for this (Jack, Queen, King, etc) — simple numbers (1-10) are good enough.
Your program should deal the first two cards, then keep dealing more
cards as long as the total points are less than or equal to the
limit
, and they haven’t gone over 21.
For testing purposes, write a simple main()
function that calls this
oneHand(limit)
function, like this:
def main():
limit = 15
points = oneHand(limit)
print(points)
As you can see, oneHand(..)
should return the result (points) of
the simulated one hand of blackjack.
Here are a few examples of running the program so far:
$ python3 blackjack.py
Card1: 1, Card2: 1 -- total = 2
Next Card: 10 total = 12
Next Card: 7 total = 19
19
$ python3 blackjack.py
Card1: 8, Card2: 7 -- total = 15
Next Card: 8 total = 23
23
$ python3 blackjack.py
Card1: 10, Card2: 1 -- total = 11
Next Card: 2 total = 13
Next Card: 1 total = 14
Next Card: 1 total = 15
Next Card: 1 total = 16
16
In the first example above, the first two cards were Aces (one point each), so the total was 2, which is less than the limit (15) so it went on to deal another card (and another).
In the second example, the first two cards (8 and 7) are still less than or equal to the limit (15), so it dealt another card (an 8) and went over 21.
3.2. finish main()
Once you have oneHand(..)
working, add some code to main()
to
play 10 games and output the results.
Requirements for this program:
-
play 10 games, each with
limit=15
-
keep track of and display at the end how many games went over 21
-
output a smiley-face when we get 21 :)
-
output a sad-face when we go over!
-
obviously, in
oneHand(..)
, show the cards being dealt and total points
Here is an example of the finished program (no user input is required):
$ python3 blackjack.py
Card1: 4, Card2: 7 -- total = 11
Next Card: 7 total = 18
Game 1 Final total = 18
= = = = = = = = = = = = = = = = = = = =
Card1: 6, Card2: 9 -- total = 15
Next Card: 1 total = 16
Game 2 Final total = 16
= = = = = = = = = = = = = = = = = = = =
Card1: 8, Card2: 9 -- total = 17
Game 3 Final total = 17
= = = = = = = = = = = = = = = = = = = =
Card1: 6, Card2: 1 -- total = 7
Next Card: 7 total = 14
Next Card: 10 total = 24
Game 4 Final total = 24 :(
= = = = = = = = = = = = = = = = = = = =
Card1: 1, Card2: 4 -- total = 5
Next Card: 2 total = 7
Next Card: 8 total = 15
Next Card: 7 total = 22
Game 5 Final total = 22 :(
= = = = = = = = = = = = = = = = = = = =
Card1: 5, Card2: 6 -- total = 11
Next Card: 10 total = 21
Game 6 Final total = 21 :)
= = = = = = = = = = = = = = = = = = = =
Card1: 5, Card2: 6 -- total = 11
Next Card: 3 total = 14
Next Card: 10 total = 24
Game 7 Final total = 24 :(
= = = = = = = = = = = = = = = = = = = =
Card1: 6, Card2: 5 -- total = 11
Next Card: 8 total = 19
Game 8 Final total = 19
= = = = = = = = = = = = = = = = = = = =
Card1: 4, Card2: 5 -- total = 9
Next Card: 7 total = 16
Game 9 Final total = 16
= = = = = = = = = = = = = = = = = = = =
Card1: 4, Card2: 7 -- total = 11
Next Card: 8 total = 19
Game 10 Final total = 19
= = = = = = = = = = = = = = = = = = = =
For 10 games and limit=15: 3 went over.
4. Answer the Questionnaire
Each lab will have a short questionnaire at the end. Please edit
the Questions-04.txt
file in your cs21/labs/04
directory
and answer the questions in that file.
Once you’re done with that, run handin21
again.
Turning in your labs….
Remember to run handin21
to turn in your lab files!
You may run handin21
as many times as you want. Each time it will
turn in any new work. We recommend running handin21
after
you complete each program or after you complete significant
work on any one program.