CS21 Lab 2: Numbers and Strings
Due Saturday, February 8, before midnight
Programming Tips
As you write your first programs, start using good programming practices now:
-
Use a comment at the top of the file to describe the purpose of the program (see example).
-
All programs should have a
main()
function (see example). -
Use variable names that describe the contents of the variables.
-
Write your programs incrementally and test them as you go. This is really crucial to success: don’t write lots of code and then test it all at once! Write a little code, make sure it works, then add some more and test it again.
-
Don’t assume that if your program passes the sample tests we provide that it is completely correct. Come up with your own test cases and verify that the program is producing the right output on them.
Are your files in the correct place?
Make sure all programs are saved to your cs21/labs/02
directory! Files
outside that directory will not be graded.
$ update21
$ cd ~/cs21/labs/02
$ pwd
/home/username/cs21/labs/02
$ ls
Questions-02.txt
(should see your program files here)
Goals
The goals for this lab assignment are:
-
Manipulate Python numeric and string data types
-
Learn to apply the accumulator pattern to numeric and string types to solve problems
-
Learn to iterate over data using
for
loops -
Practice string operations
+, len(), []
1. tip jar
Your friend is a barrista at a lively coffee shop. This coffee shop has a unique tip policy: for every sale a barrista makes, a tip of 10% is automatically added to the customer’s total.
Write a program tipjar.py
to keep track of your friend’s tips. Your program should
input the amount customers spend and output the total tips earned. The number of customers
should also be specified.
One example of running the program is shown below. User input is shown in bold.
$ python3 tipjar.py
Enter the number of customers: 5
Customer 1
Enter the amount spent: $8
The barrista gets $ 0.8 for tip
Customer 2
Enter the amount spent: $5
The barrista gets $ 0.5 for tip
Customer 3
Enter the amount spent: $11.50
The barrista gets $ 1.1500000000000001 for tip
Customer 4
Enter the amount spent: $1
The barrista gets $ 0.1 for tip
Customer 5
Enter the amount spent: $3
The barrista gets $ 0.30000000000000004 for tip
The total tip is $ 2.8500000000000005
Your program should have the following features:
-
Ask the user for the number of customers
-
For each customer, ask for the amount of money they spent
-
When all customer information is entered, print the total tip amount
To print a blank line, you can call print() with no arguments. |
2. can’t get a word in entwise
"We must not be hasty." —Treebeard in The Two Towers
The Ents in Lord of the Rings were a noble race of tree herders, whose language was "long and sonorous". Ents live a very long time and so speak very…carefully…and….very…s.l.o.w.l.y.
In slow.py
, implement a program to turn a phrase into ent-speech. Your program
should ask for the pause length and a phrase to change. Your program should
output an ent-phrase. Ent-phrases contain '.' between each letter in the input.
Two examples of the running program are shown below. User input is shown in bold.
$ python3 slow.py
Pause length: 3
Text: Surprise!
S...u...r...p...r...i...s...e...!...
$ python3 slow.py
Pause length: 5
Text: Hmmm?
H.....m.....m.....m.....?.....
Features:
-
The pause length corresponds to the number of '.' that appear between each letter in the input
3. life of the party
You are very popular and you’re planning a party. The only trouble is that it’s hard to estimate the number of guests: you always invite your closest friends, who invite their closest friends, who invite their closest friends, and so on!
For example, suppose 2 levels of invites go out after you invite 2 friends. Those friends will each invite two more, who will each invite 2 more, who will each invite 2 more! The total guests will be 14 people!
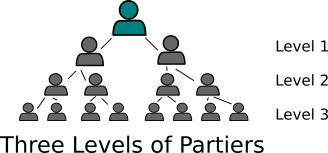
Write a program party.py
to compute the number of guests who will come to your
party given how many friends you tell about it. Assume that there are 6 levels
of invites that will be sent.
Two examples of the running program are shown below. User input is shown in bold.
$ python3 party.py
Enter your number of close friends: 2
You invite 2 friends
Your friends invite 4 more people!
Your friends friends invite 8 more people!
Your friends friends friends invite 16 more people!
Your friends friends friends friends invite 32 more people!
Your friends friends friends friends friends invite 64 more people!
Your friends friends friends friends friends friends invite 128 more people!
You expect 254 people at your party!
$ python3 party.py
Enter your number of close friends: 5
You invite 5 friends
Your friends invite 25 more people!
Your friends friends invite 125 more people!
Your friends friends friends invite 625 more people!
Your friends friends friends friends invite 3125 more people!
Your friends friends friends friends friends invite 15625 more people!
Your friends friends friends friends friends friends invite 78125 more people!
You expect 97655 people at your party!
Features/Hints:
-
Each level multiplies the number of additional guests
-
Use a loop to compute the total and update the message
-
Use string accumulation to change the message
-
If you get stuck, get your program working for 3 levels first.
4. crossword
In crossword.py
, implement a program that inputs text and outputs a word square whose letters
are arranged like a crossword puzzle.
$ python3 crossword.py
Enter a phrase: top
top
o o
pot
$ python3 crossword.py
Enter a phrase: otter
otter
t e
t t
e t
retto
Features:
-
The first row should match the text that was entered
-
The last row is the reverse of the input text
-
The left side spells the input word from top to bottom
-
The right side spells the input word from bottom to top
Although this problem may look daunting, we don’t need to solve it all at once. Let’s break this problem into simpler subproblems and work our way up to the final solution.
4.1. Subproblem 1: Top row
The top row prints the input string. Write a program that outputs the user’s input.
$ python3 crossword.py
Enter a phrase: otter
otter
4.2. Subproblem 2: Bottom row
Using string accumulation, compute the reverse of the input string. Then print both the original and reversed string.
$ python3 crossword.py
Enter a phrase: otter
otter
retto
4.3. Subproblem 3: Left column
The left column prints each character on its own line, excluding the first and last character. To start, simply print all the characters on their own line.
$ python3 crossword.py
Enter a phrase: otter
otter
o
t
t
e
r
retto
Now, exclude the first and last characters.
$ python3 crossword.py
Enter a phrase: otter
otter
t
t
e
retto
4.4. Subproblem 4: Right column
Excluding the first and last character, print each character of the reversed string on its own line. Don’t worry about spacing to start.
$ python3 crossword.py
Enter a phrase: otter
otter
t e
t t
e t
retto
Now, add spaces between the left and right columns.
$ python3 crossword.py
Enter a phrase: otter
otter
t e
t t
e t
retto
5. Extra Challenge — string formatting
This part does not affect your grade so please only attempt this after completing the rest of your lab. It is simply an extra challenge, if you want to try it.
Similarly to assignment 1, the output for tip jar is not realistic since money does not come in smaller denominations than 1 cent (i.e., we do not need more than two digits after the decimal point). Use string formatting to clean up the output like this:
$ python3 tipjar.py
Enter the number of customers: 3
Customer 1
Enter the amount spent: 11.50
The barrista gets $1.15 for tip
Customer 2
Enter the amount spent: 3
The barrista gets $0.30 for tip
Customer 3
Enter the amount spent: 10
The barrista gets $1.00 for tip
The total tip is $ 2.45
6. Extra Challenge — checking input
This part does not affect your grade so please only attempt this after completing the rest of your lab. It is simply an extra challenge, if you want to try it.
Consider our program tipjar.py
. What should the program do if
the user enters $0? What should we do if the user enters a negative value? What if the
user accidentally enters a string that isn’t a number?
We can handle these cases by checking the user’s inputs before we use it. For this extra
challenge, add if
statements to ensure the user inputs a non-negative number. If the number is
negative, print an error and ignore the input.
$ python3 tipjar.py
Enter the number of customers: 0
The total tip is $ 0
$ python3 tipjar.py
Enter the number of customers: -2
Number of customers can't be negative. Quitting...
The total tip is $ 0
$ python3 tipjar.py
Enter the number of customers: 3
Customer 1
Enter an amount spent: 5
The barrista gets $ 0.5 for tip
Customer 2
Enter an amount spent: -4
Amount can't be negative. Skipping...
Customer 3
Enter an amount spent: 1
The barrista gets $ 0.1 for tip
The total tip is $ 0.6
7. Answer the Questionnaire
Each lab will have a short questionnaire at the end. Please edit
the Questions-02.txt
file in your cs21/labs/02
directory
and answer the questions in that file.
Once you’re done with that, run handin21
again.
Turning in your labs….
Remember to run handin21
to turn in your lab files!
You may run handin21
as many times as you want. Each time it will
turn in any new work. We recommend running handin21
after
you complete each program or after you complete significant
work on any one program.
Logging out
When you are all done working in the lab, you should log out of the computer
you are using. First quit any applications you are running, like the browser and
the Terminal. Then click on the logout icon
and choose "log out".
If you plan to leave the lab for just a few minutes, and then come right
back to work, you do not need to log out. It is, however, a good idea to lock
your machine while you are gone. You can lock your screen by clicking on the
lock icon.