Classes and objects
-
Classes are a generalized definition of an object
-
An instance of a class is called an object. When we create an object, we say we instantiate an object of the class
-
Example: cats have common attributes, like tails, whiskers, and fur. My cat, "Jersey", has specific attributes, such as a fluffy tail and black fur. "Cat" is an example of a class. "Jersey" is an instance of the class "Cat"
-
-
Classes allow use to extend the built-in data types (int, str, etc) with our own types
-
In programming, classes are used to represent nouns whereas functions are used to represent verbs
-
Classes combine data and functions, e.g. "objects know stuff and they can do stuff"
-
Functions declared in a class are called methods
-
Classes bundle together data to create new types. For example, a class which represents different movies might contain each movie’s title, duration, year made, director, and actors. The Movie class might contain functions for printing credits or asking whether someone was an actor in a movie.
Data |
Methods |
|
|
As another example, suppose we have a class for representing x,y points
Data |
Methods |
|
|
Objects can contain other objects. For example, if we defined a class to represent a circle, it might contain a point to represent the center and a float to represent its radius.
Data |
Methods |
|
|
Similarly to functions, we have different syntax for defining classes and for using them.
Defining classes
We will talk in detail about how to implement classes at the end of the semester. For now, it’s most important to know how to use classes. For some people, this is easier if you also know how they are defined. If you are not one of these people, skim this section.
Syntax
class <ClassName>:
# Constructor
# To call this method: obj = <ClassName>(<arg1>,<arg2>,...,<argN>)
# There can be any number of parameters (even zero!)
# All code to initialize the data in our object goes here!
def __init__(self, <param1>, <param2>, ...., <paramN>):
self.<data1> = <param1>
self.<data2> = <param2>
....
self.<dataN> = <paramN>
# any number of methods can be created
# Every method has to have `self` as the first parameter!
# Methods can have any number of additional parameters
# Members can have a return type (or not!)
def <method1>(self, ...):
<body1>
def <method2>(self, ...):
<body2>
...
def <methodN>(self, ...):
<bodyN>
For examples of class definitions, see movie.py
and point.py
in your class examples!
Using classes
At this point of the course, it’s important that you understand how to use classes which have already been defined for you.
Syntax
# Instantiate an object.
# The number of arguments must match the parameters in the constructor defn
obj = <ClassName>(<arg1>,<arg2>,...<argN>)
# Call method on object
obj.<method1>()
# Access data inside object
obj.<data1>
Example: movie.py
Below is an example of using the movie class in a program
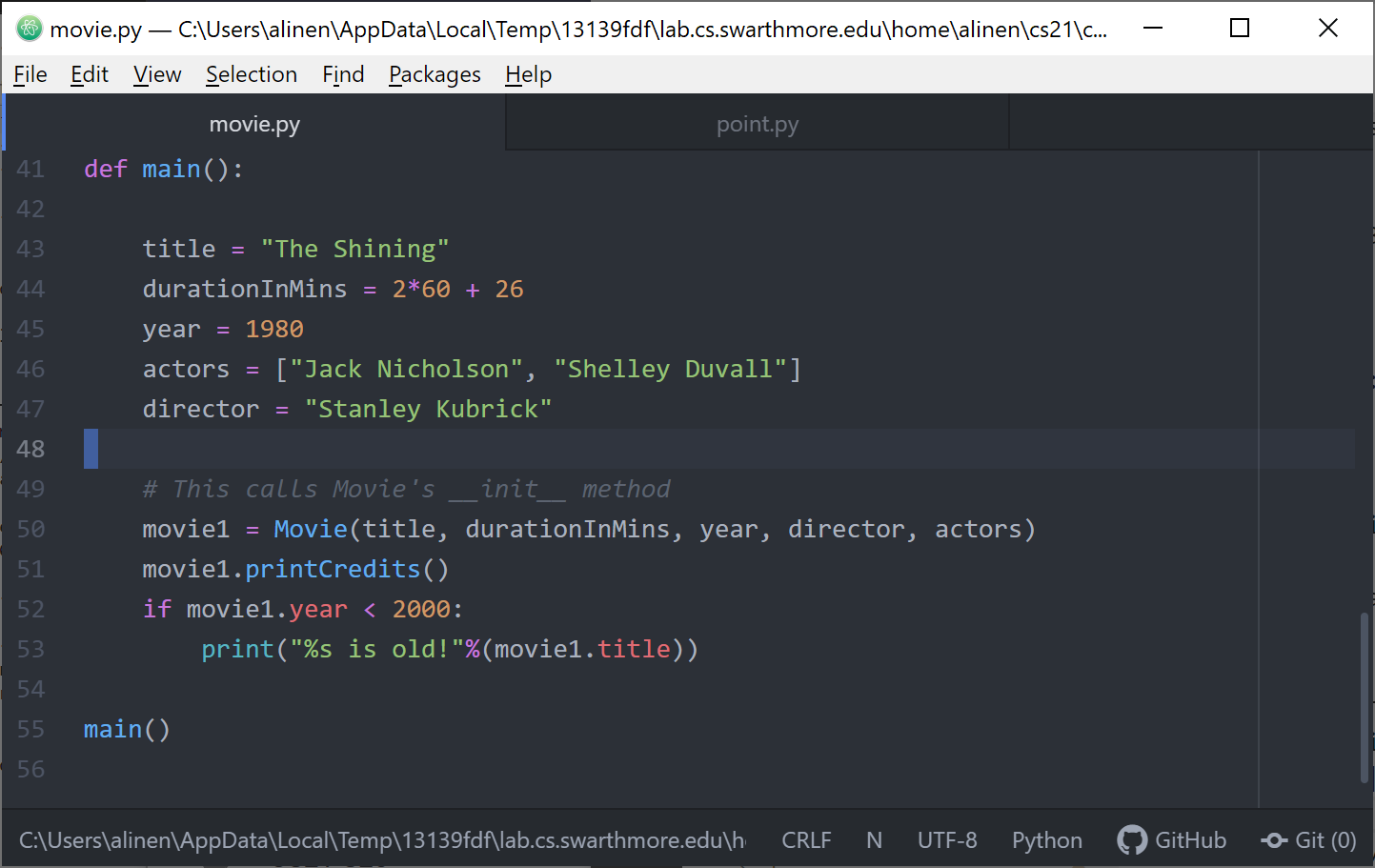
-
What is the output of the program?
Title: The Shining Duration (minutes): 146 Year: 1980 Director: Stanley Kubrick Actors: Jack Nicholson Shelley Duvall The Shining is old!
-
What variables are in scope on line 51? What are their types?
title |
str |
duration |
int |
year |
int |
actors |
list (of str) |
director |
str |
movie1 |
Movie |
-
How do we call the method printCredits() which belongs to movie1?
movie1.printCredits()
-
What is the stack diagram on line 51? Note that object in memory have both data and methods!
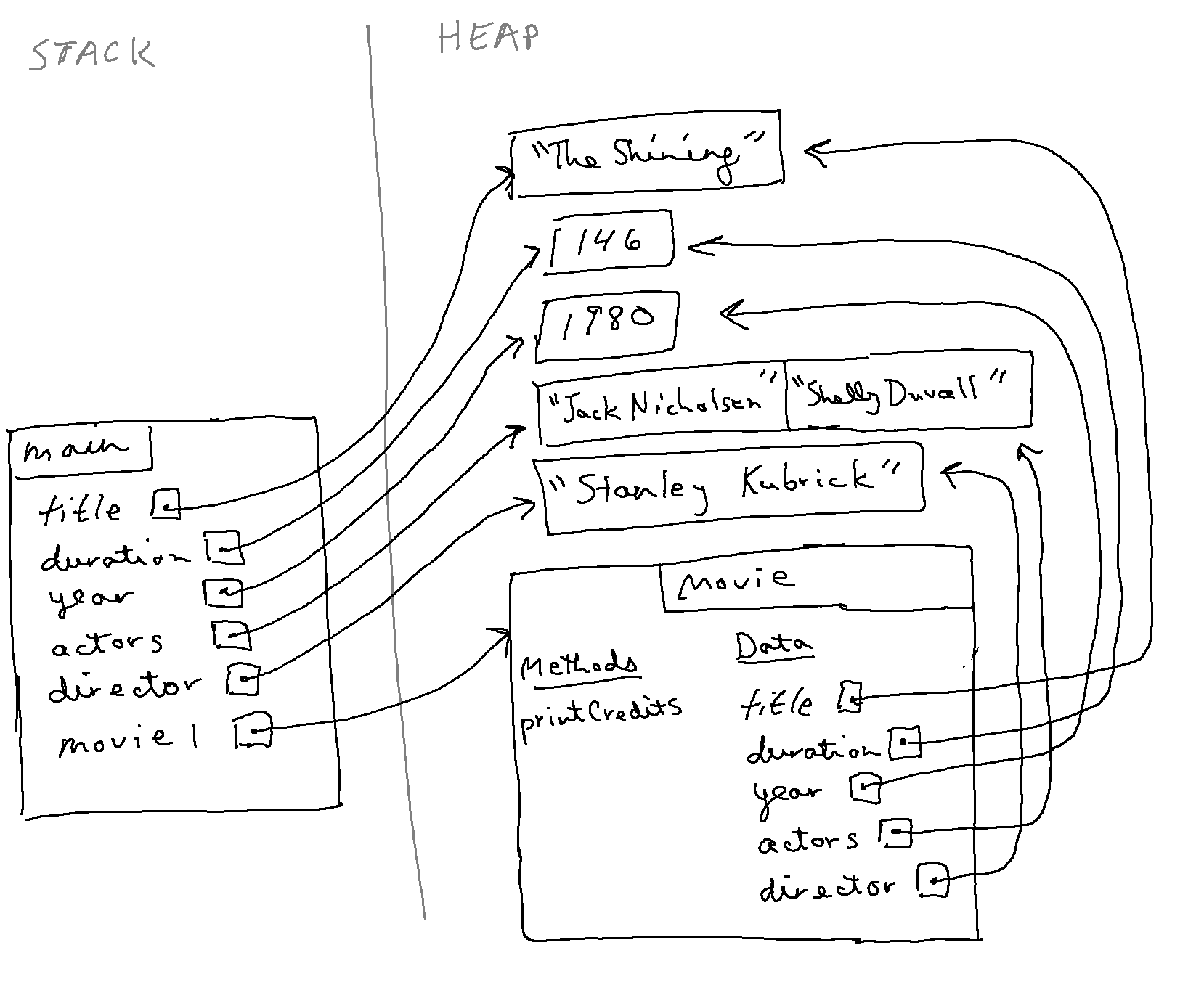
Example: point.py
Below is an example of using our own point class in a program
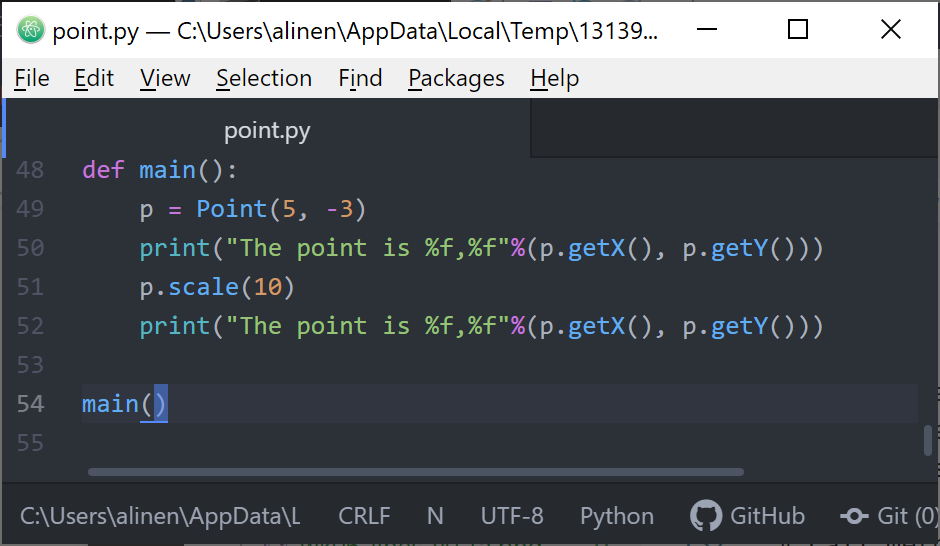
-
What is the output of the program?
The point is 5,-3 The point is 50,-30
-
What variables are in scope on line 51?
p
(only one) -
What type is
p
? It has typePoint
. It is an object. Therefore, its type is its class.
The stack diagram for this program looks like the following on line 52. Note that objects contain both data and methods in memory!
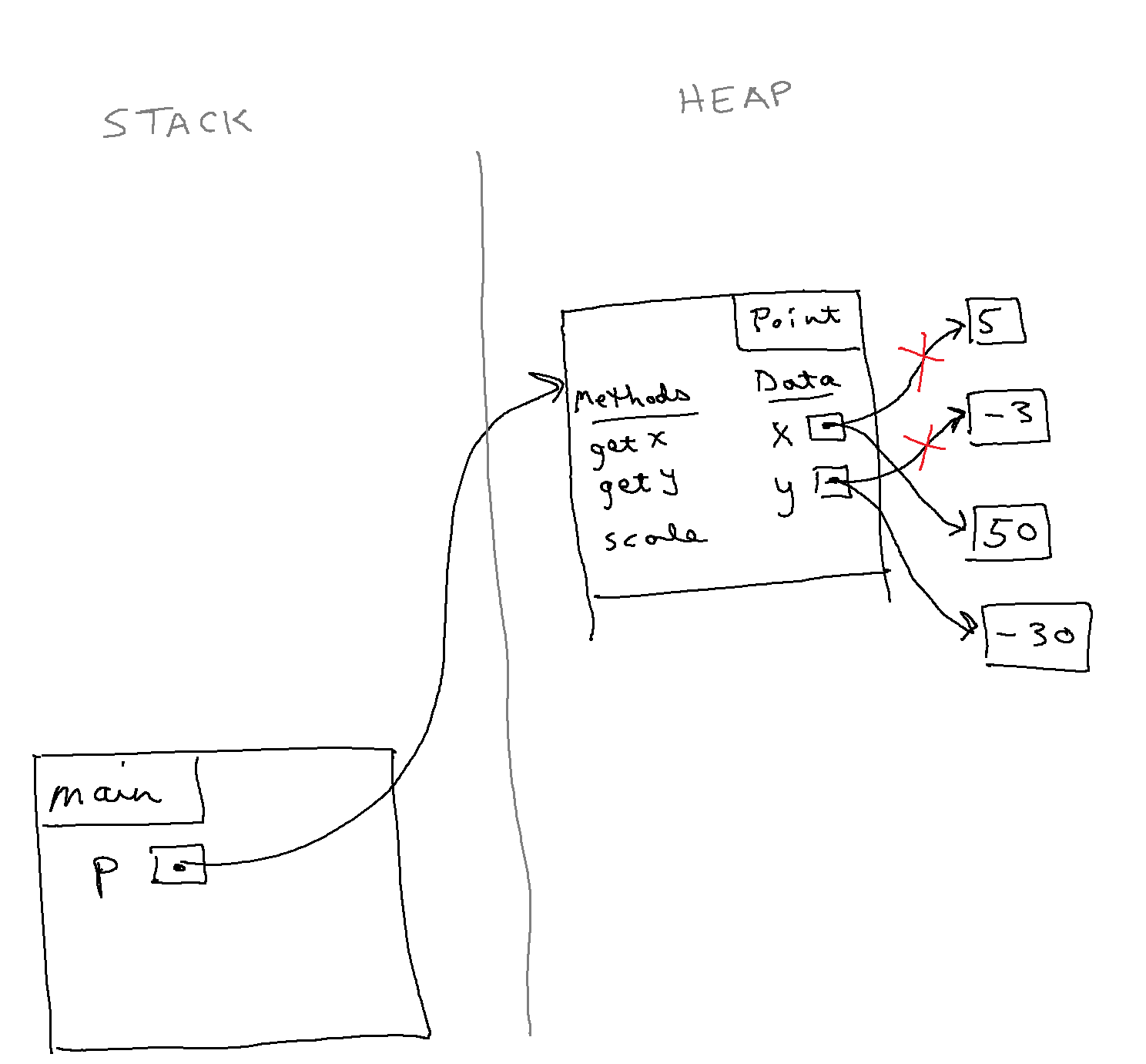
Graphics
We will use the Zelle graphics library to practice with classes. Zelle defines classes for circles, points, lines, rectangles, and more!
The best way to learn how to use the objects in Zelle is by (reading the documentation.
Exercise: helloGraphics.py
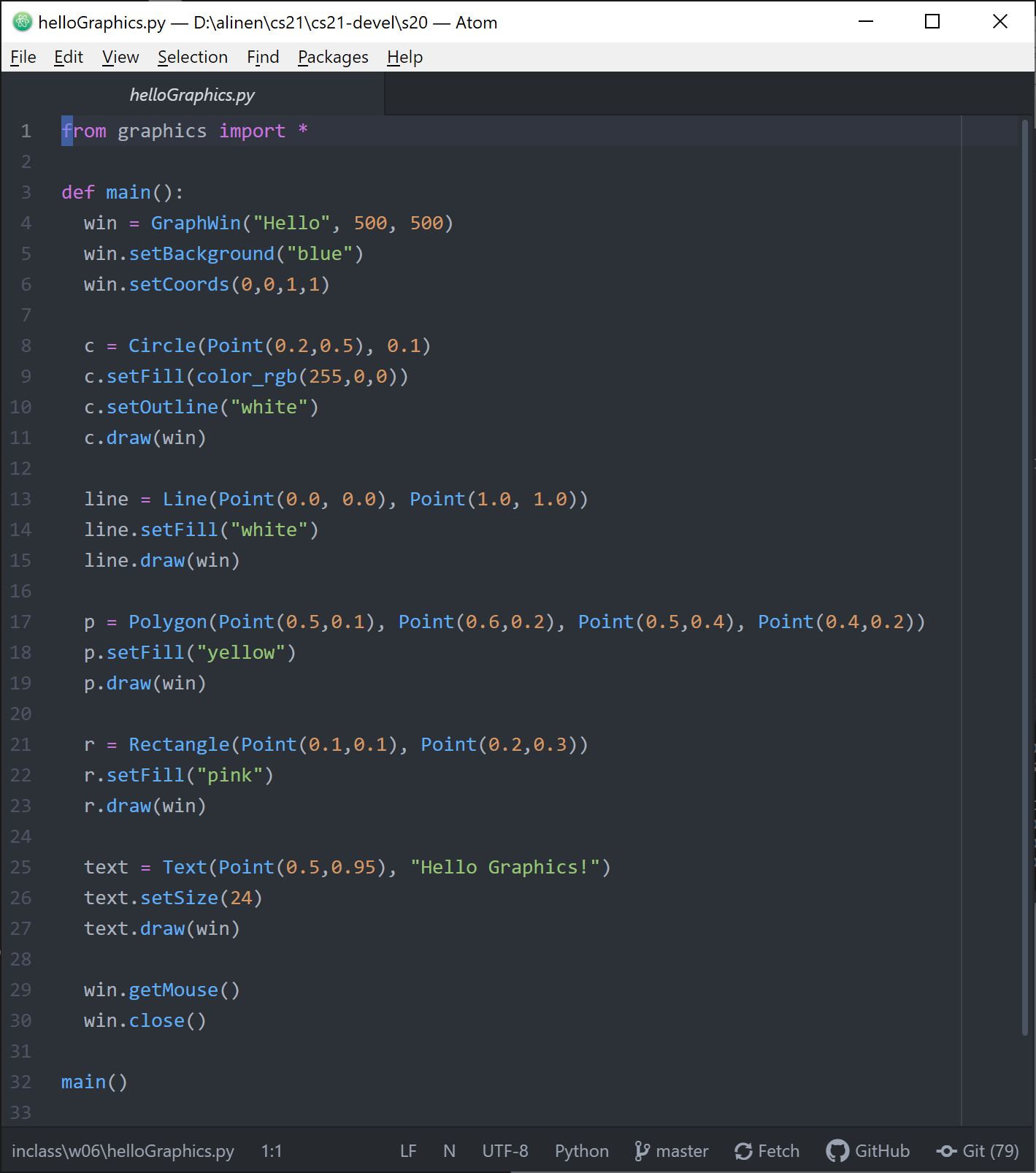
Exercise: lines.py
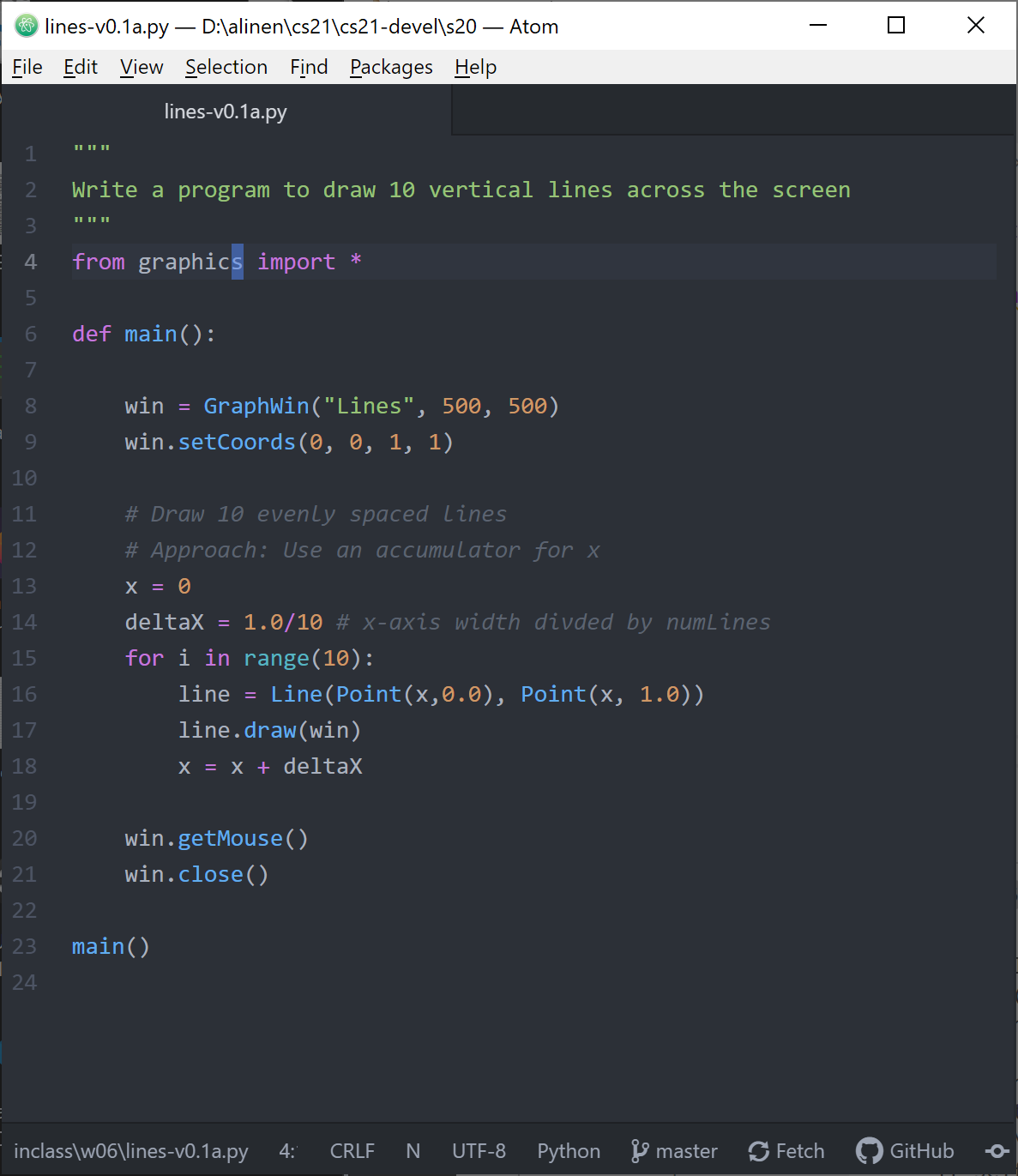
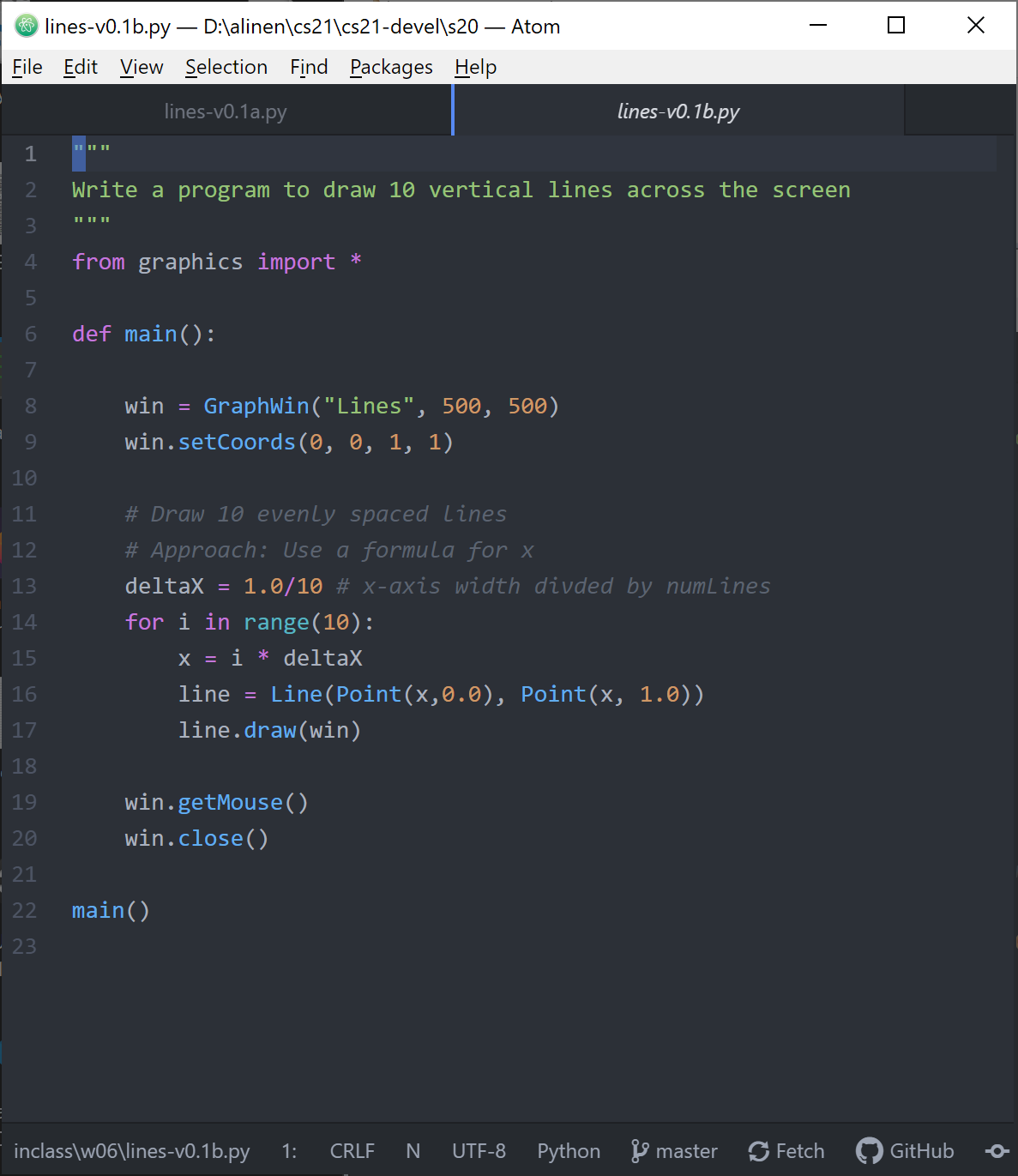
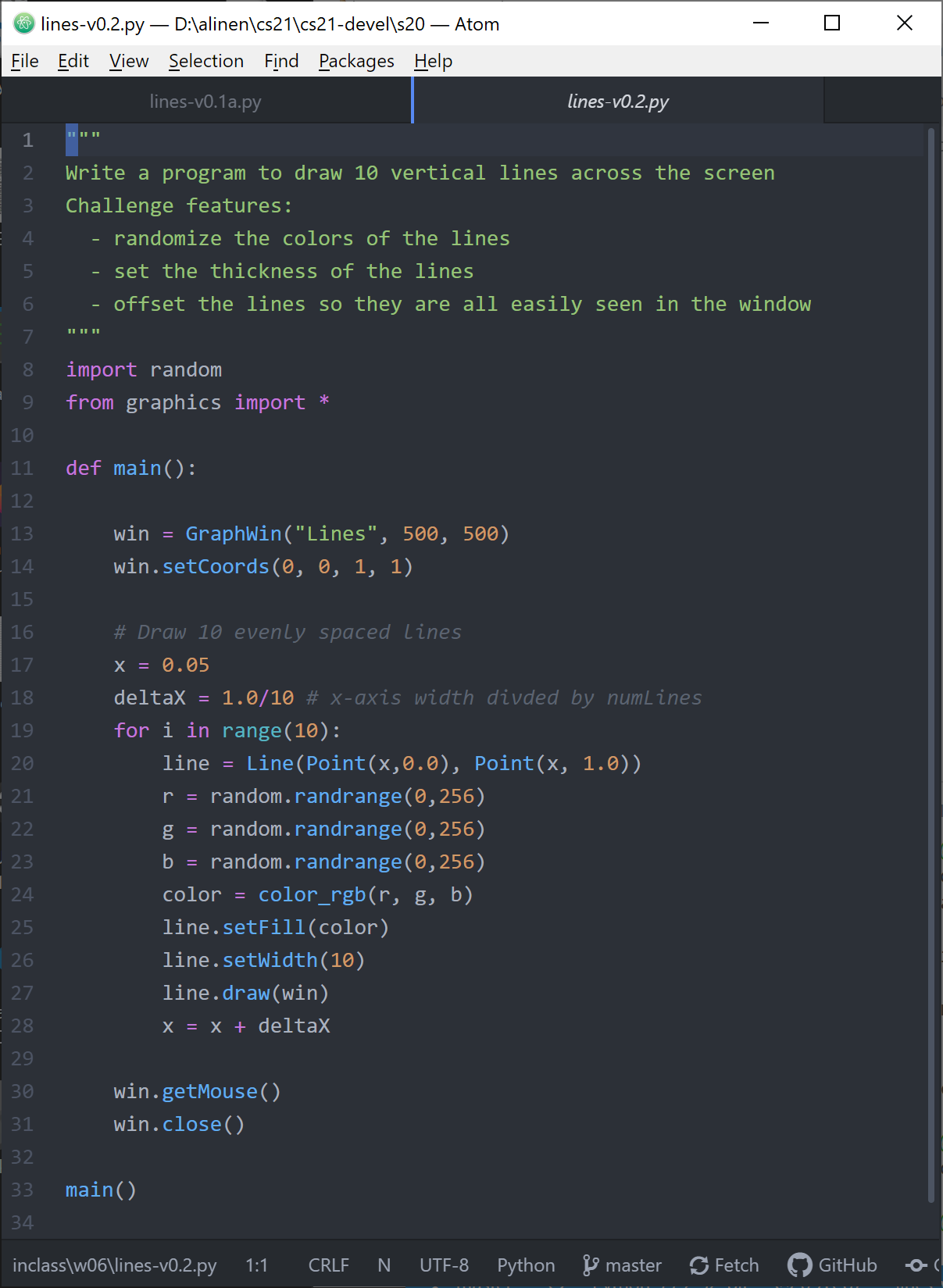
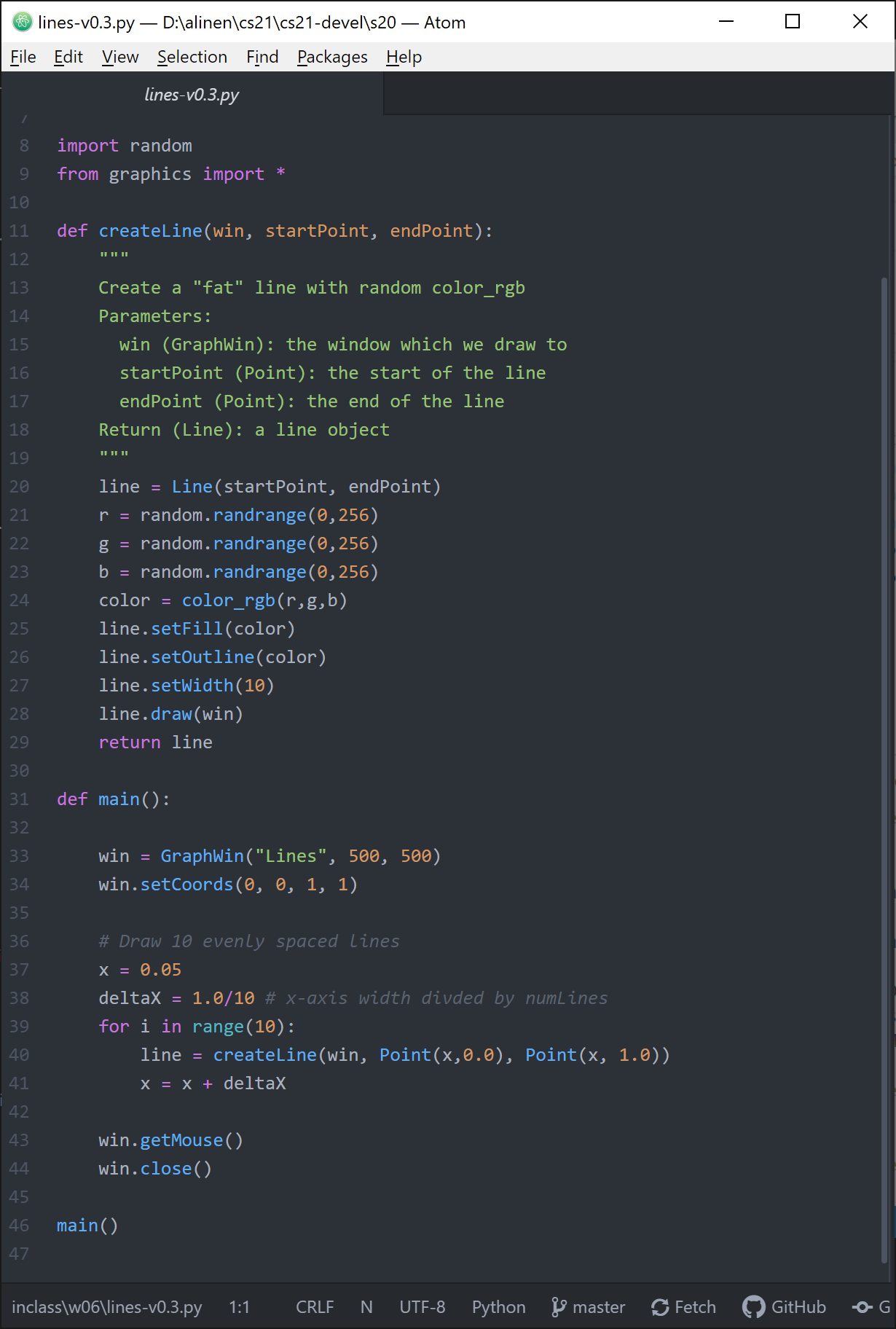
Questions:
-
Verify that the values for x are the same when we use either an accumulator or the formula based on
i
-
Why do we need to pass
win
as an argument tocreateLine
? -
What data type is
win
? How is the data type of objects determined?
Exercise: helloMouse.py
The example below uses mouse input to create a rectangle.
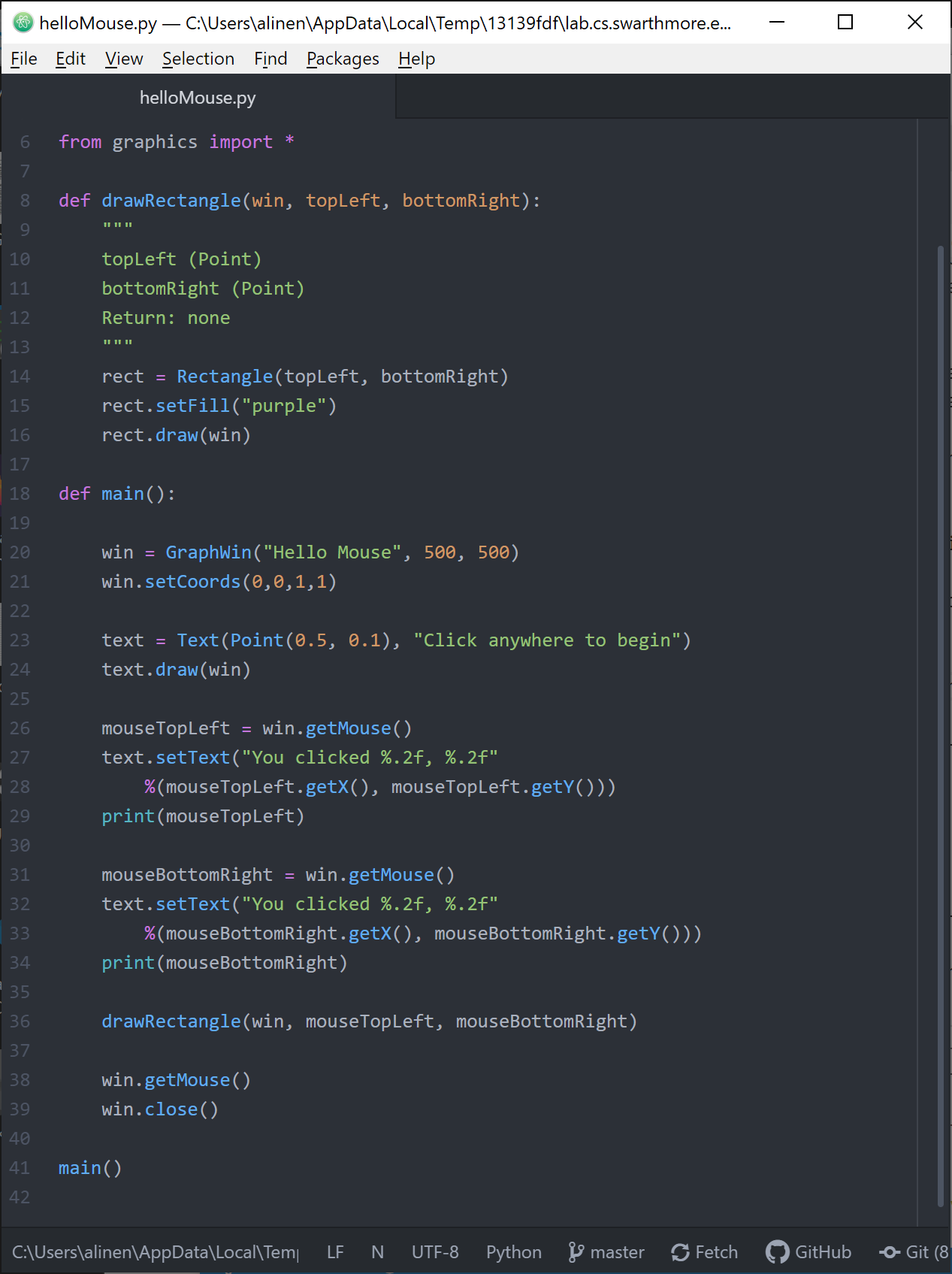
Exercise: helloAnimation.py
The example below moves a circle from one side of the screen to the other
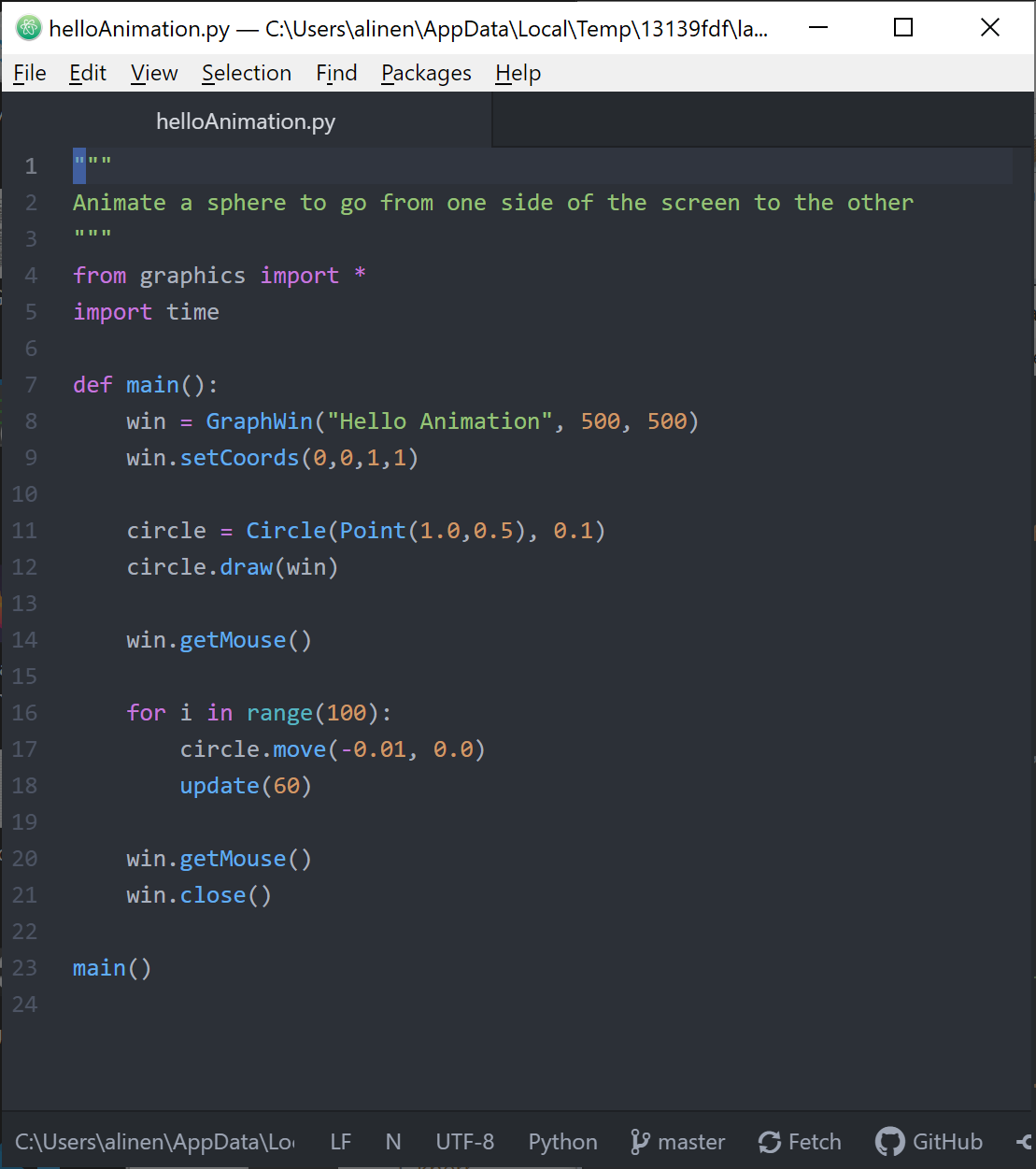
Importing our own code
So far, we have been cutting and pasting code when we want to reuse it. There is a much
better way. We can use import
for our own code just like we use it for random
and
graphics
. We call a unit of re-useable code a library or a module.
Example: createLine.py
By using import
, we can make our createLine
function re-useable in different programs.
In this example, createLine
is in a module/library defined in createLine.py
.
image::wk06-createLine.png
Next, we modify our application like so:
image::wk06-lines-v0.4.png
Example: add.py
There is one gotcha to watch out for when creating modules. We demonstrate it below. Consider the following program. What is its output?
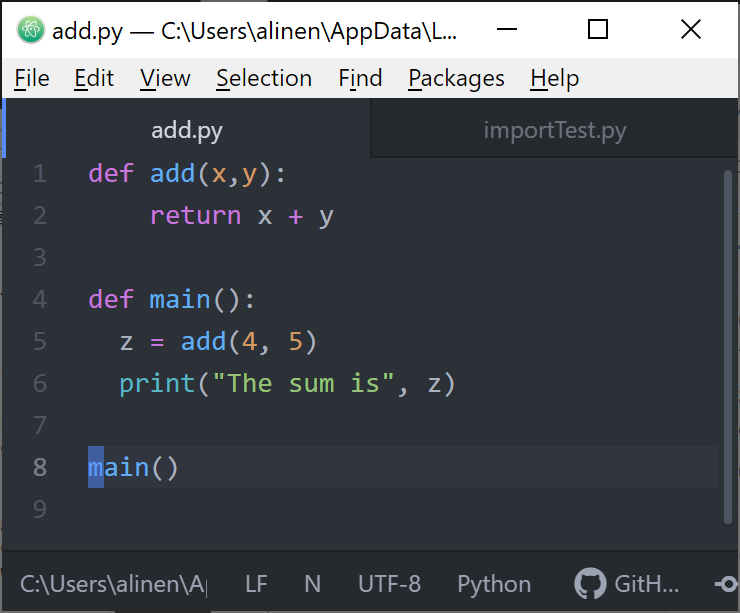
The sum is 9
Consider the following program. What is its output?
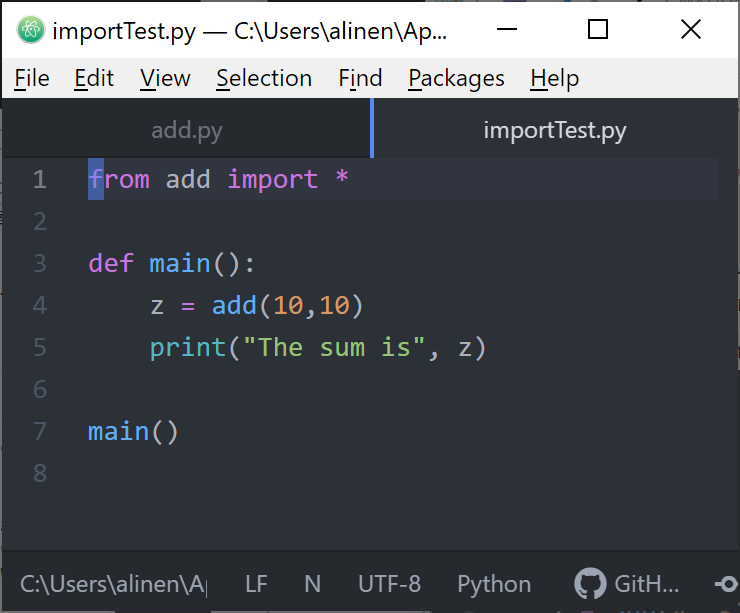
The sum is 9
The sum is 20
Why do we print two statements? Because both add.py
and importTest.py
call their own
main()
functions. Do the following to fix it:
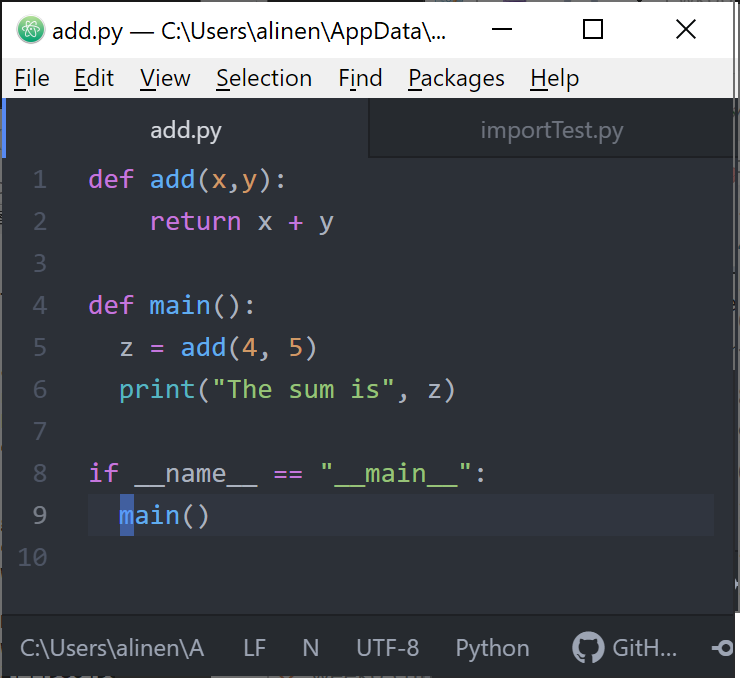
Now the output of python3 importTest.py
will be
The sum is 20
And the output of python3 add.py
will be
The sum is 9
When we run python3 add.py
, Python will define the variable name
to have
the value main
. However, when we do from add import *
, Python will set name
to something else.