guess.py
redux
Consider our class exercise guess.py
from last week.
How can we
-
keep track of whether the user guessed the number or not (outside the loop)?
-
stop asking the user for more numbers after they have guessed correctly?
To keep track of whether the user was successful of not, we need a boolean variable. We have two choices to guard against asking the user for numbers after they guess the right one:
-
if
statement -
break
statement
guess.py
if statement
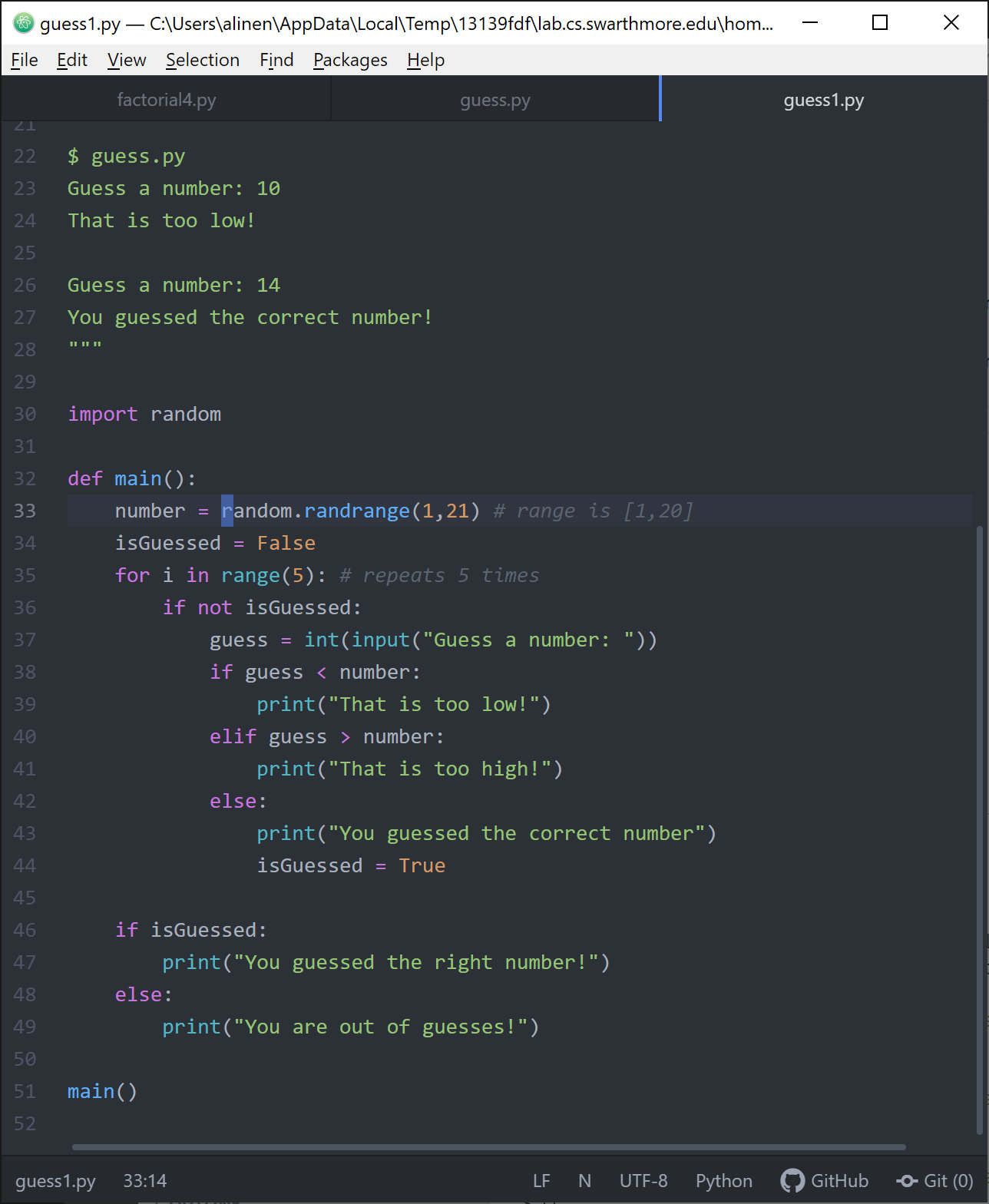
guess.py
break
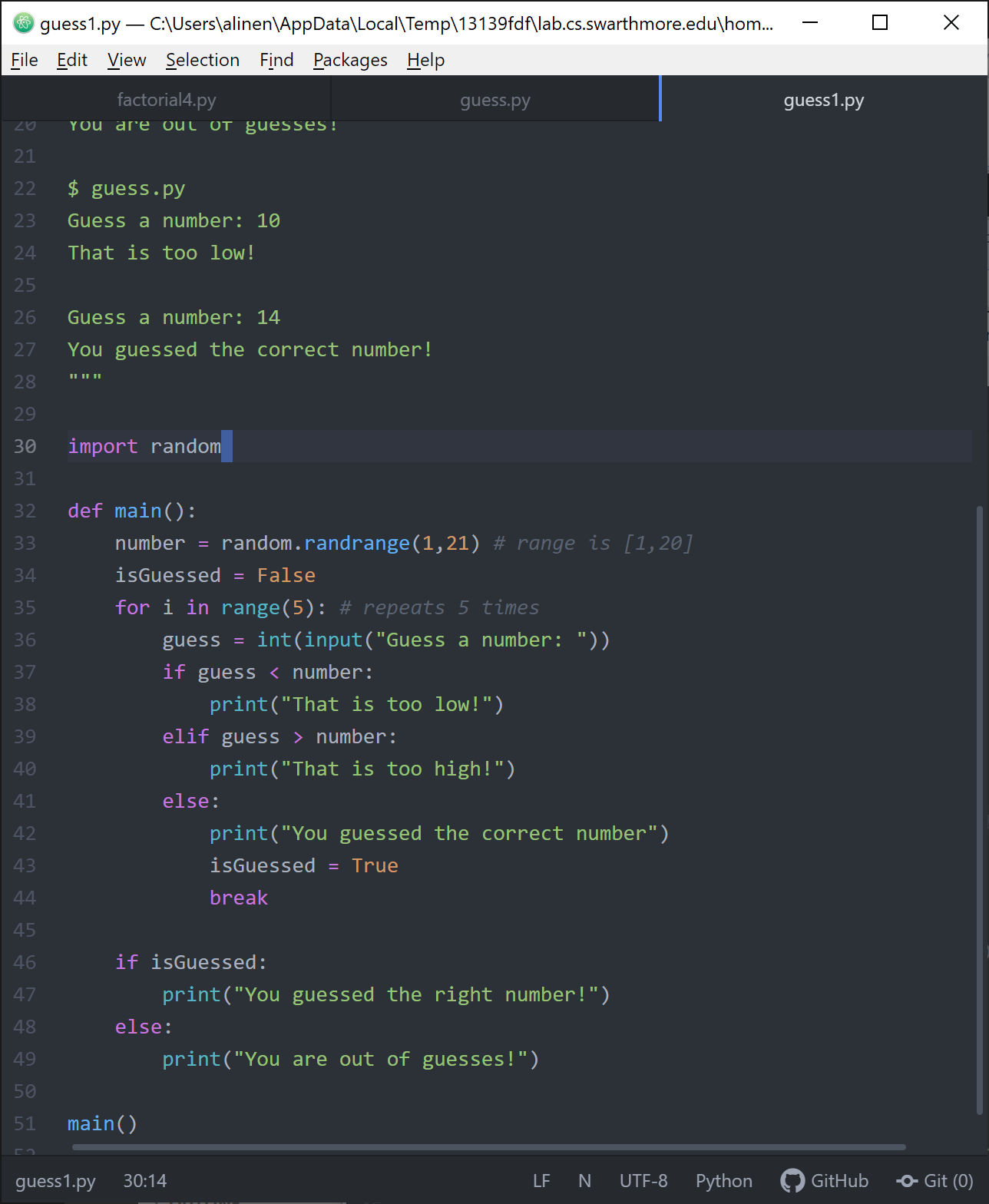
While loop
for
loops are an example of a definite loop. They always loop for a fixed
number of times.
while
loops can loop an undetermined amount of time.
while <condition>:
<body>
where <body> executes for as long as <condition> evaluates to True.
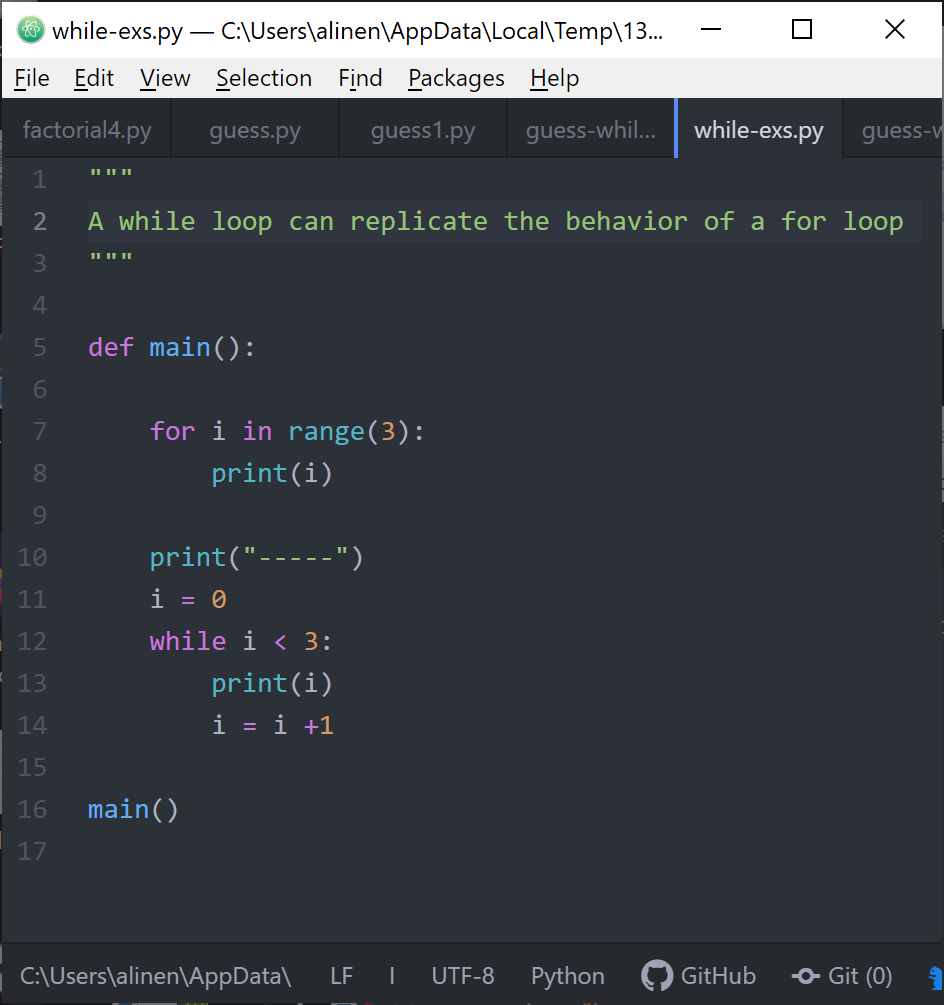
iteration |
i |
i < 3 |
i + 1 |
1 |
0 |
T |
1 |
2 |
1 |
T |
2 |
3 |
2 |
T |
3 |
4 |
3 |
F |
exits |
Infinite loops
A while loop can repeat forever if its <condition> never becomes False! This is called an infinite loop. If your program infinite loops, it will become stuck at the console.
-
Type Ctrl-C to forcibly exit your program when it infinite loops!
Exercise: guess.py
while
The code below replicates the program with the for loop. Neither the
if-statement
or a break
is needed.
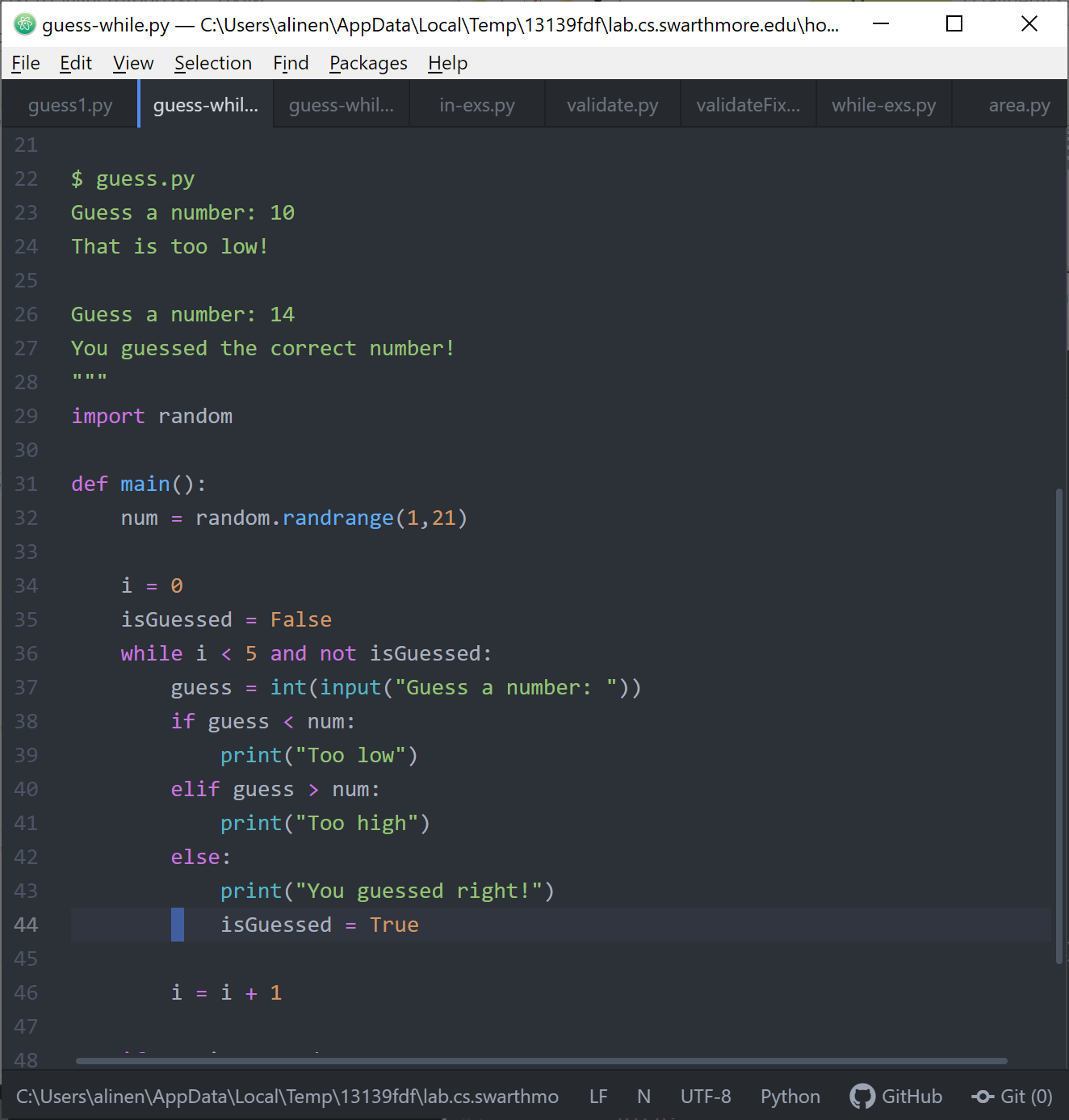
This example lets the user guess as many times as they wish!
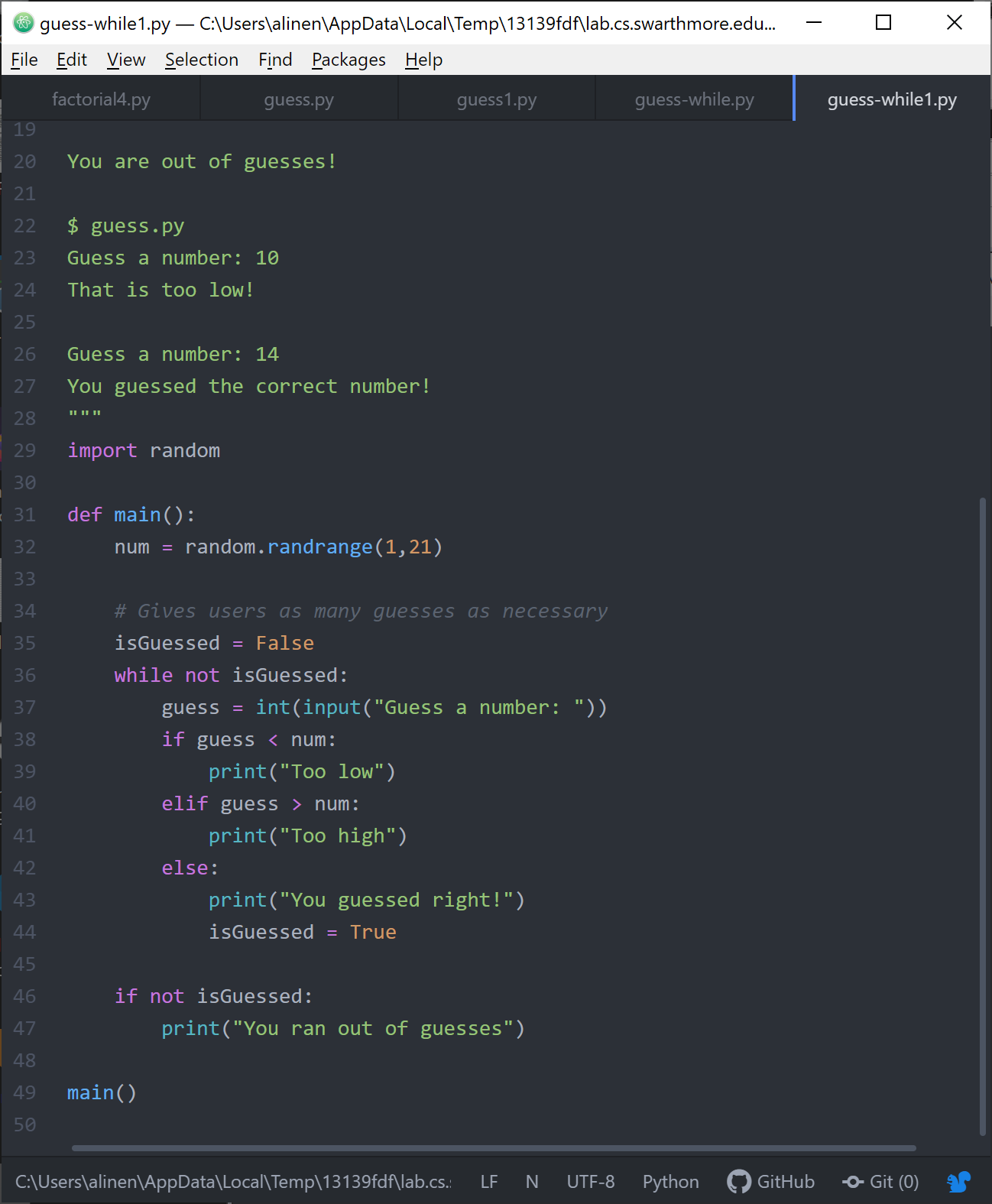
String formatting
Python3 offers several methods for string formatting.
Method 1:
"string containing <token1> <token2> ... <token N>"%(<value1>, <value2>, ..., <valueN>)
where tokens can be
-
%s
for strings -
%f
for floats -
%d
for integers
Tokens can take additional arguments.
-
%<NUM>s
pads NUM spaces before the string -
%<NUM>d
pads NUM spaces before the integer -
%<NUM1>.<NUM2>f
pads NUM1 spaces before the float and NUM2 digits after the decimal
Demo
rosemary[~]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> text = "apples"
>>> val = 3.14567
>>> quantity = 10
>>> "The string format: %s %f %f"%(text, val, quantity)
'The string format: apples 3.145670 10.000000'
>>> "The string format: %20s %f %f"%(text, val, quantity)
'The string format: apples 3.145670 10.000000'
>>> "The string format: %2s %2.2f %2d"%(text, val, quantity)
'The string format: apples 3.15 10'
>>> "The string format: %5s %5.2f %5d"%(text, val, quantity)
'The string format: apples 3.15 10'
>>> "The string format: %10s %10.2f %10d"%(text, val, quantity)
'The string format: apples 3.15 10'
>>> "The string format: %s %.3f %d"%(text, val, quantity)
'The string format: apples 3.146 10'
Method 2:
"string containing {<token1>} {<token2>} ... {<token N>}".format(<value1>, <value2>, ..., <valueN>)
f"string containing {<value1>:<token1>} {<value2>:<token2>} ... {<valueN>:<token N>}"
where tokens can be
-
:s
for strings -
:f
for floats -
:d
for integers
Tokens can take additional arguments similarly to the old method.
However, you can also include the values to print along with their format
if you pre-pend the string with f
.
>>> "The string format: {:s} {:.3f} {:d}".format(text, val, quantity)
'The string format: apples 3.146 10'
>>> "The string format: {:s} {:f} {:d}".format(text, val, quantity)
'The string format: apples 3.145670 10'
>>> "The string format: {:10s} {:10.2f} {:10d}".format(text, val, quantity)
'The string format: apples 3.15 10'
>>> f"The string format: {text} {val} {quantity}"
'The string format: apples 3.14567 10'
>>> "The string format: {text} {val} {quantity}"
'The string format: {text} {val} {quantity}'
>>> f"The string format: {text} {val:0.2f} {quantity}"
'The string format: apples 3.15 10'
in
keyword
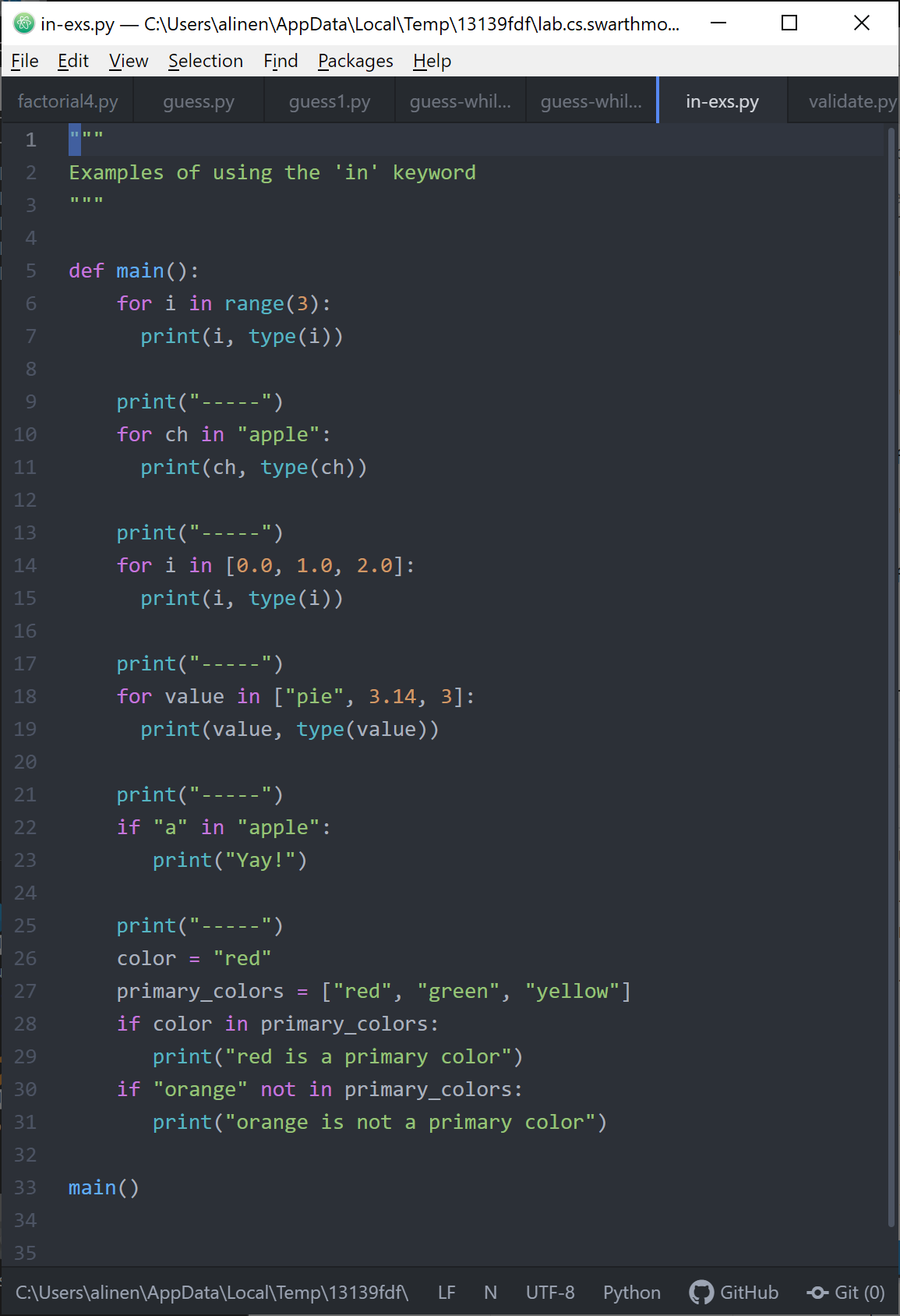
Exercise: Boolean types practice
Functions
Function definition syntax:
def <name>(<param1>, <param2>, ...., <paramN>):
<body>
[return <value>]
-
You can pass as many arguments (aka parameters, aka inputs) as you like to a function
-
the return value is optional. If there is no return value, the function doesn’t return anything.
-
Above is a function definition. To actually call the function, you use the name and pass in parameters
validate.py
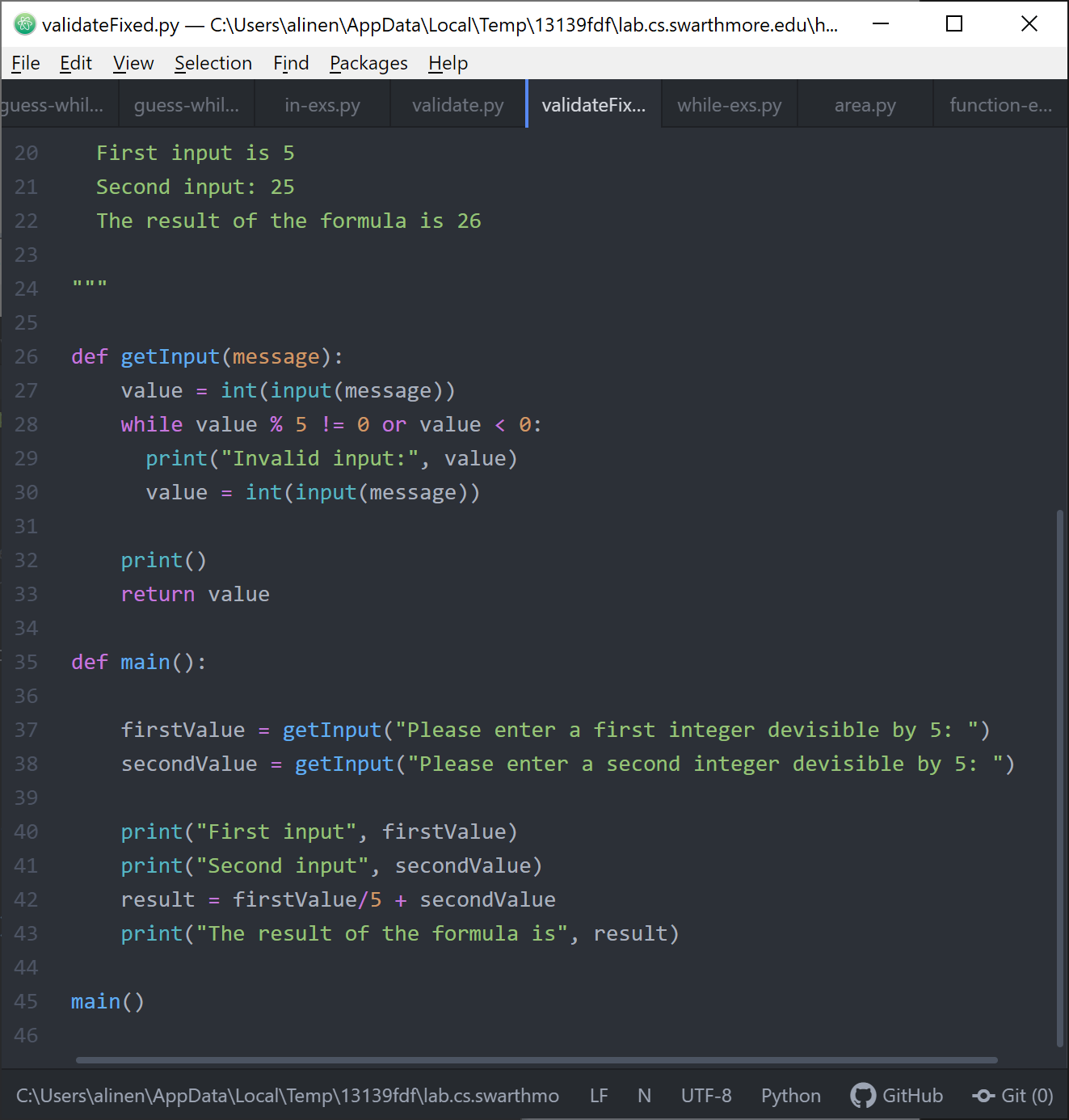
area.py
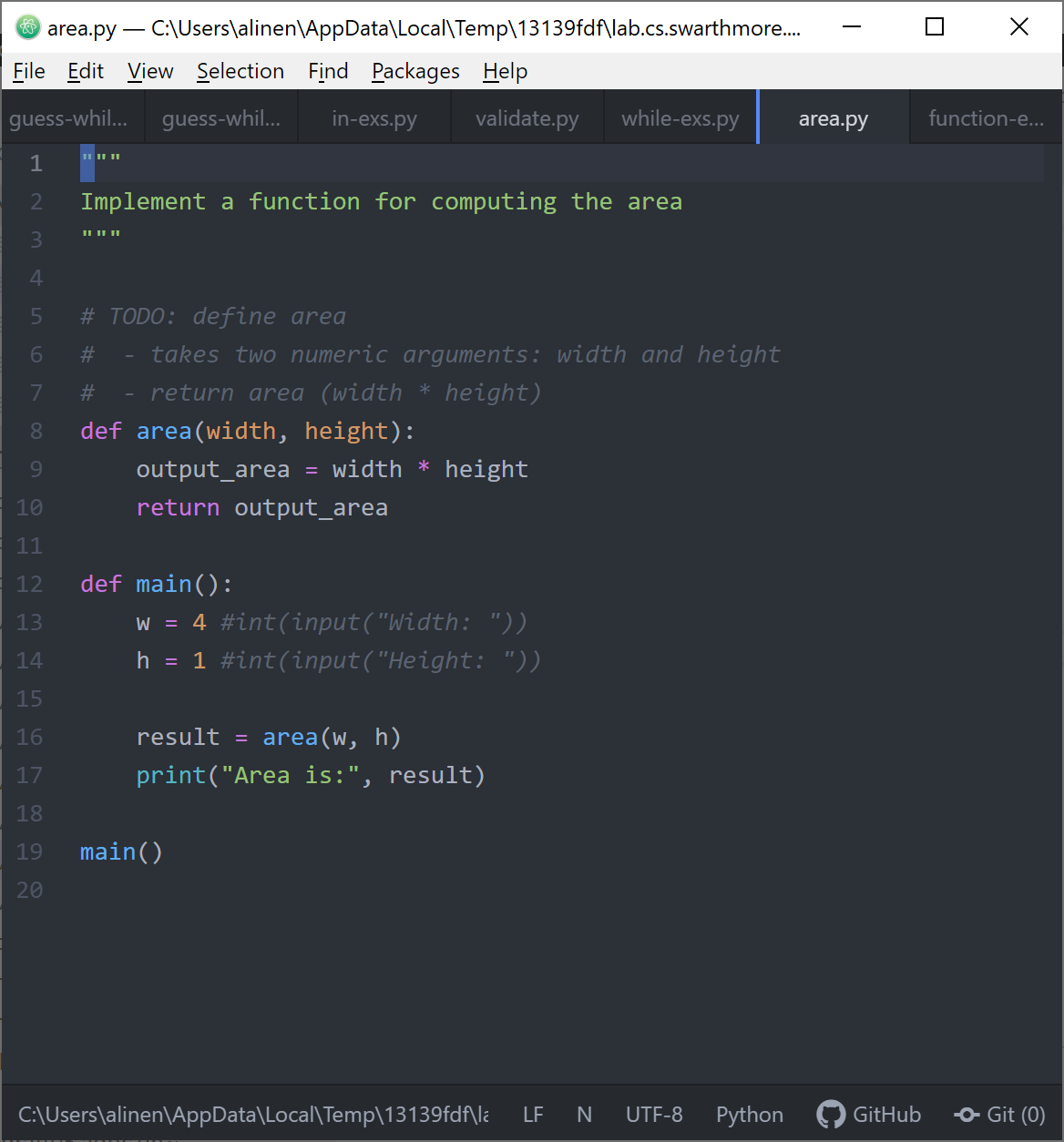
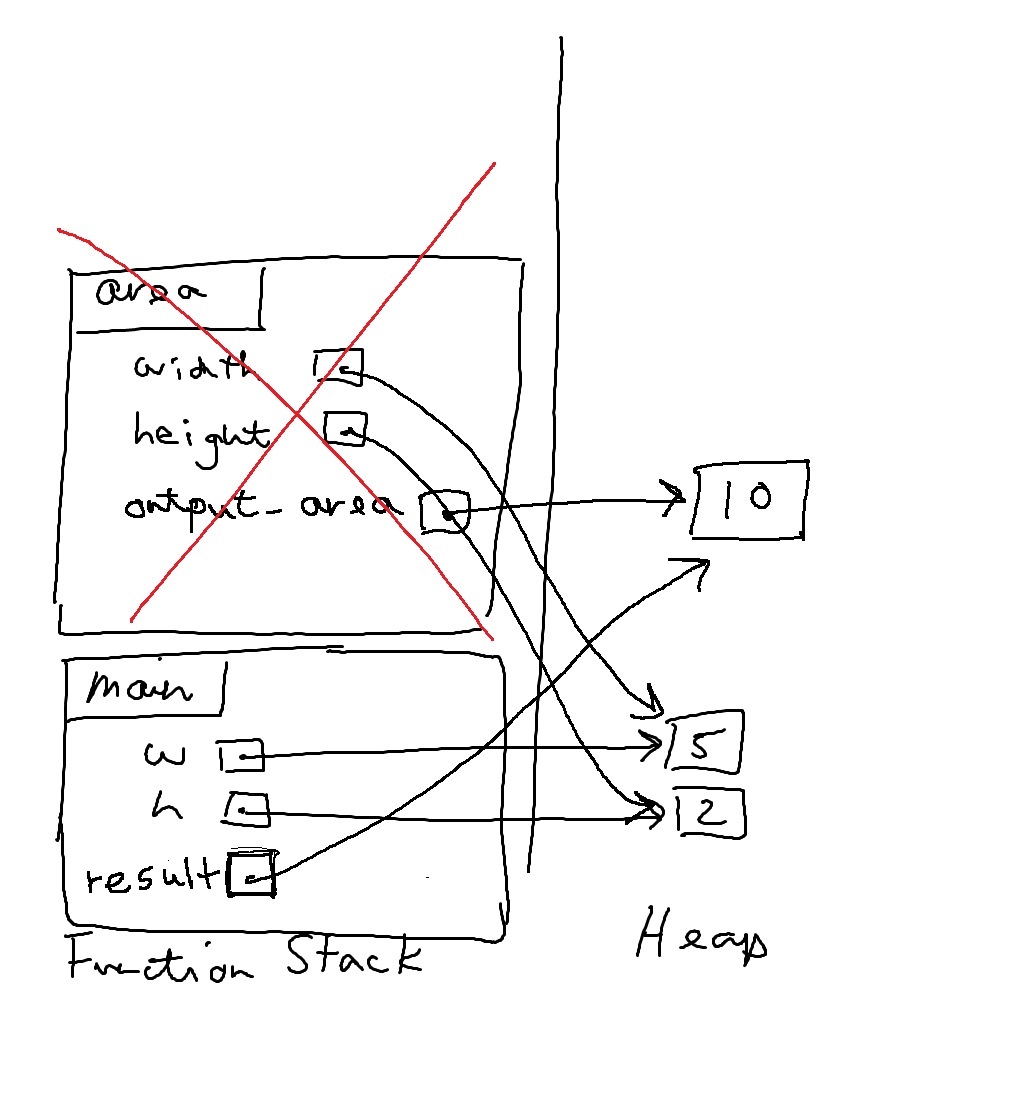
Function execution summary
-
Pause when you see a function call
-
Create a stack frame for the function and push it onto the stack
-
allocate parameters
-
-
Copy argument values to parameters
-
Execute the function, line by line, until return
-
Send back the return value (if there is one)
-
Pop the function off the stack. Destroy the frame
-
Resume the calling function