Branching if/elif/else
Branching statements allow us to express
if it's cold outside
wear a jacket
if you're happy and you know it
clap your hands
Single if statement
if <condition>:
<body>
-
<condition> is an expression that evaluates to either True or False.
-
<body> is one or more statements that execute only when <condition> is true
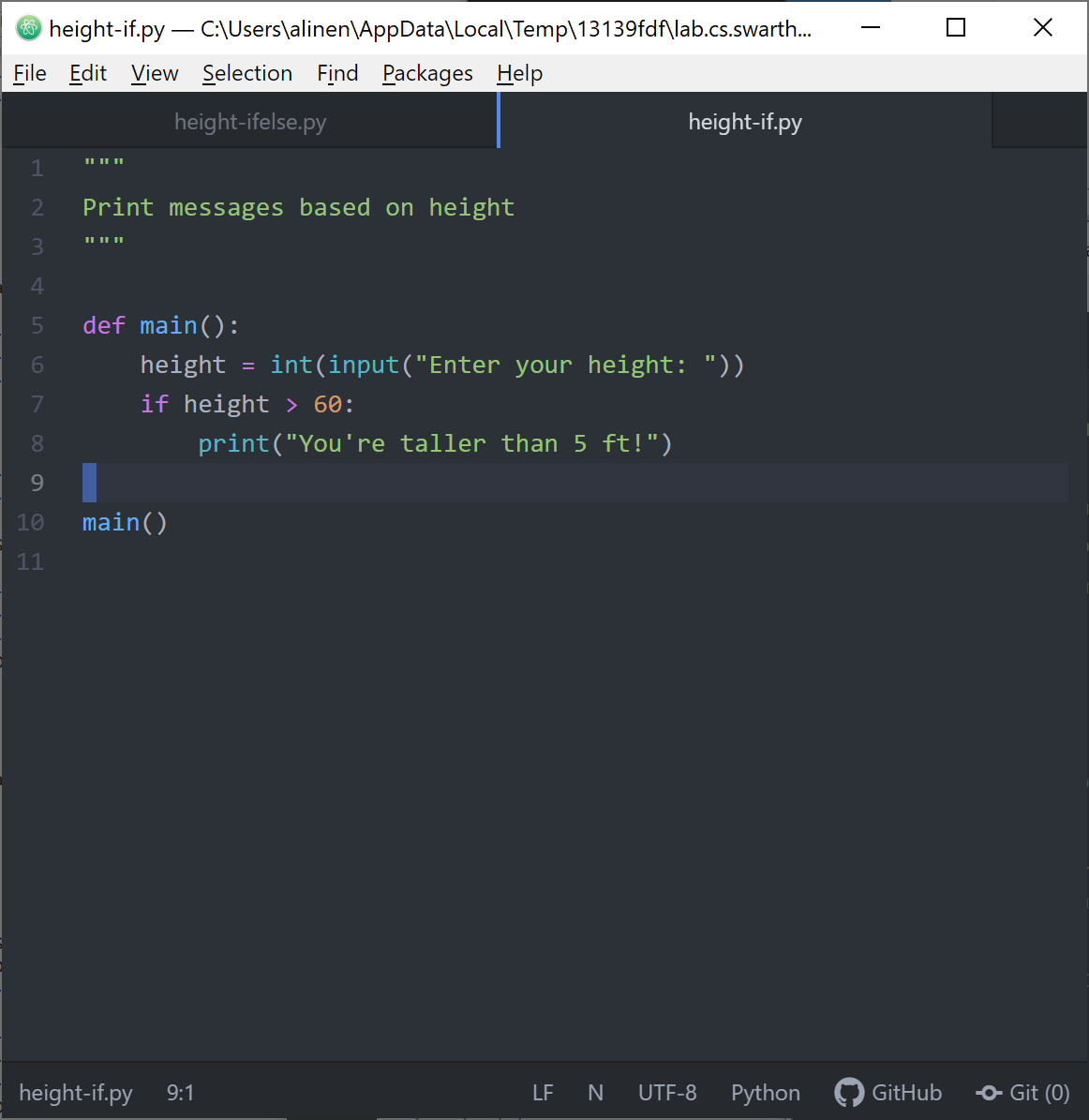
Two-way if/else statement
if <condition>:
<body1>
else:
<body2>
-
<body1> executes when <condition> is True
-
<body2> executes when <condition> is False
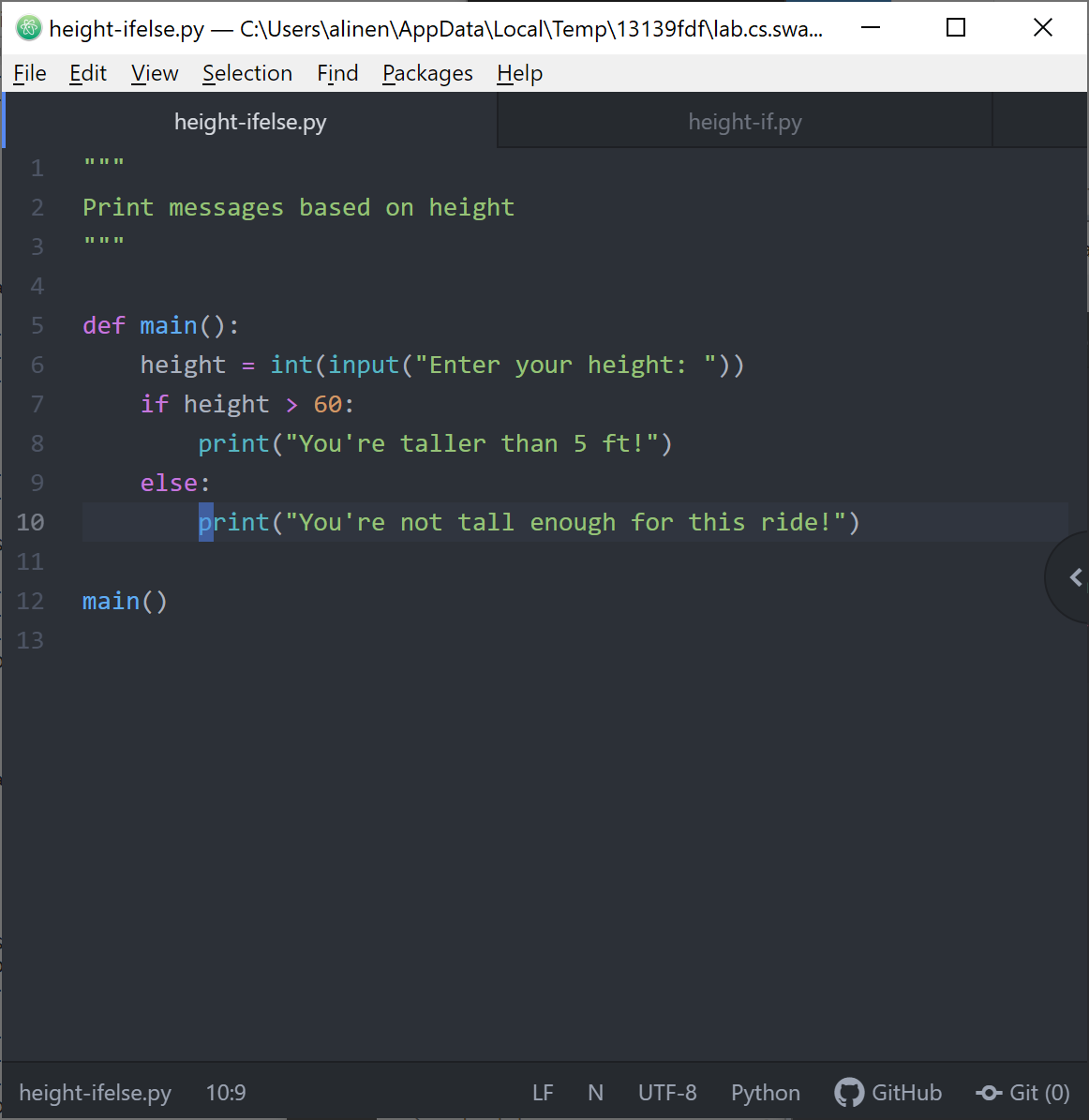
Multi-way if/else statement
if <condition>:
<body1>
elif <condition2>:
<body2>
.
.
.
elif <conditionN>:
<bodyN>
else:
<bodyN+1>
-
All conditions are tested in order, top to bottom. The first condition to be True will have its <body> executed.
-
The else statement is optional
-
<bodyN+1> when all conditions are False
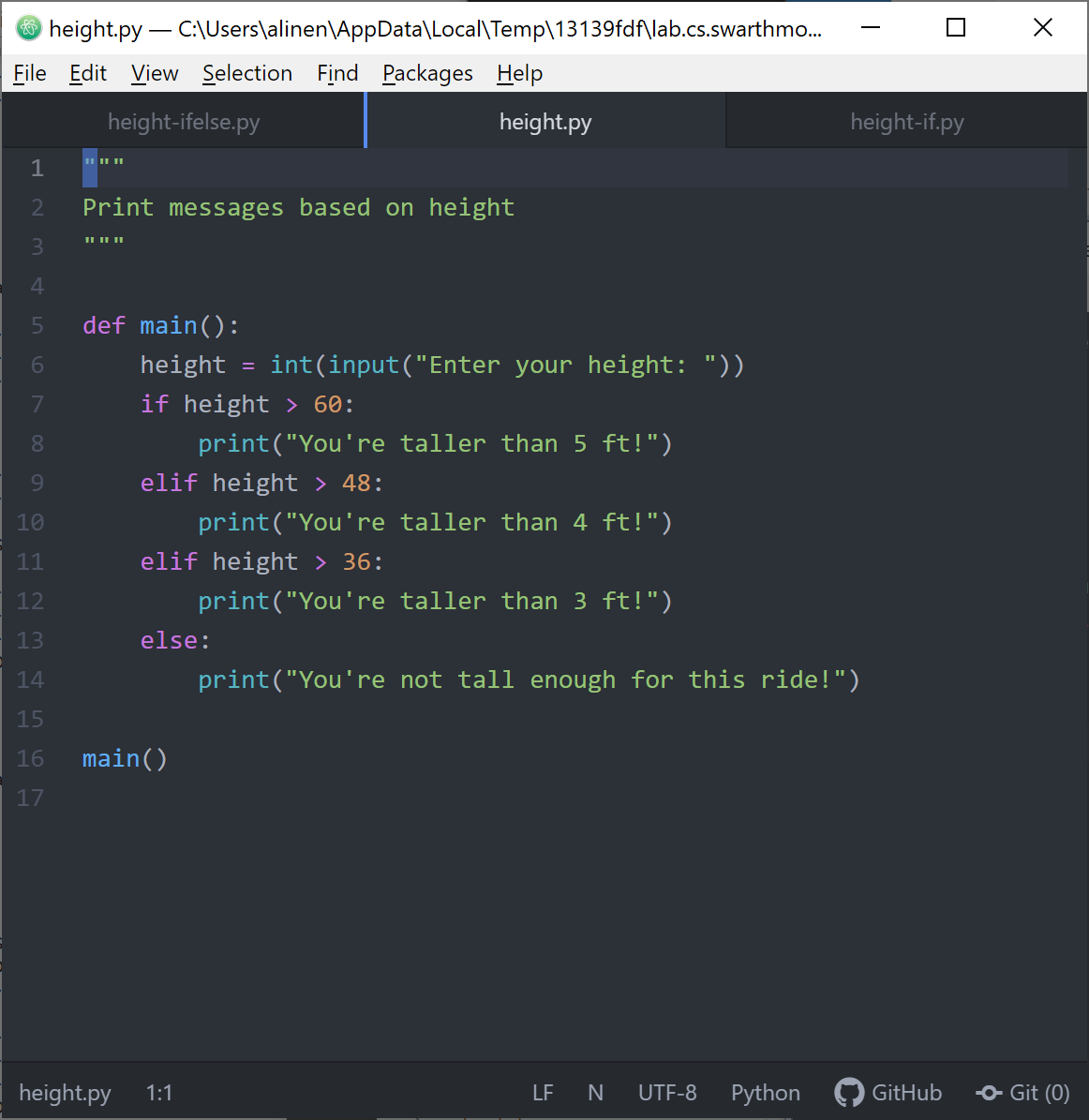
Exercise: Bug1
The following code doesn’t execute correctly. What’s wrong?
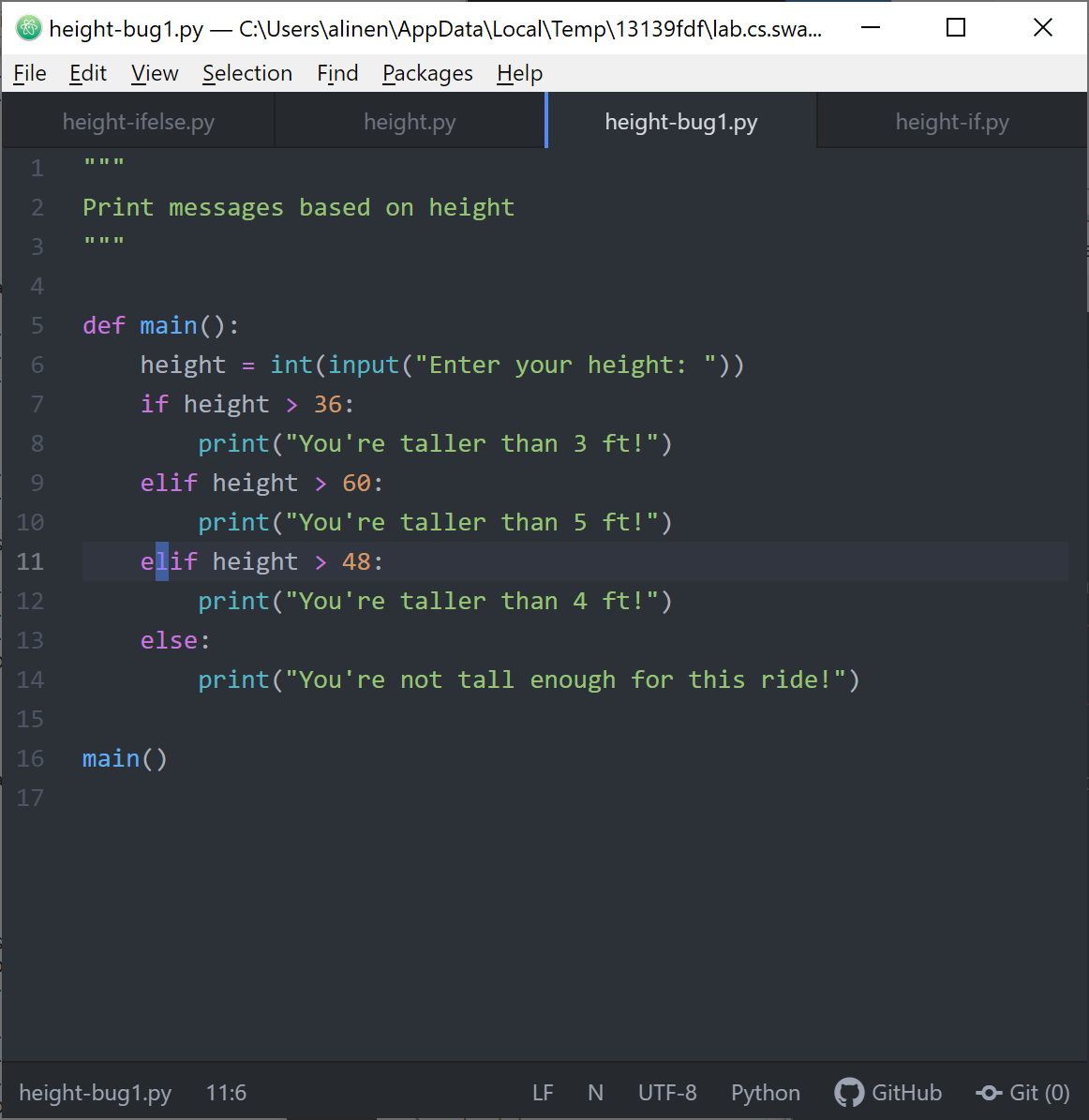
Exercise: Bug2
The following code doesn’t execute correctly. What’s wrong?
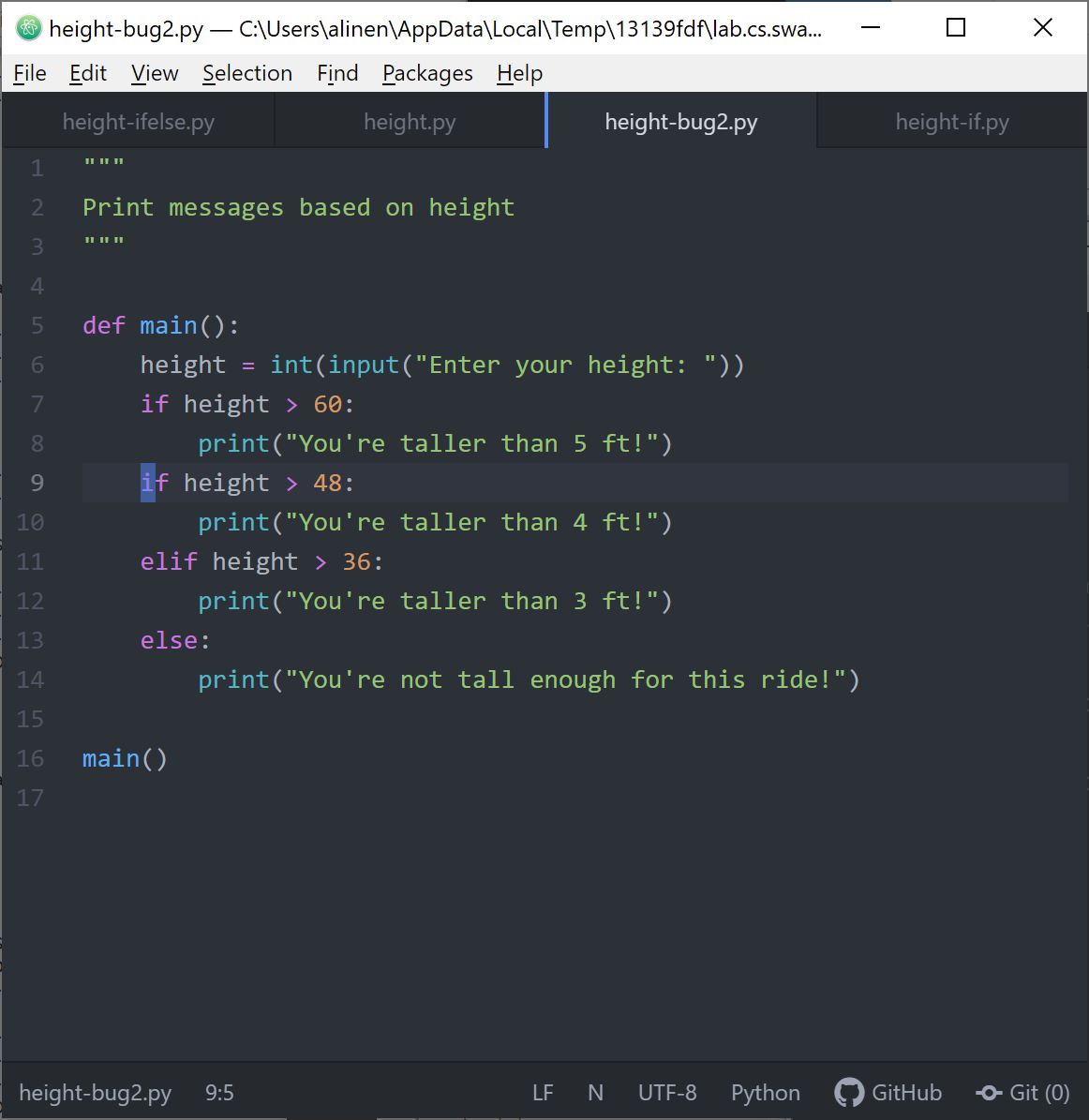
Conditionals
Booleans
Boolean data types can have one of two possible values
-
True
-
False
Relational operators
>, >=, ⇐, == (don’t confuse with assignment!!), !=
Relational operators for strings
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> "apple" < "bananas"
True
>>> "apple" < "apricots"
True
>>> "apple" < "kiwi"
True
>>> "apple" > "kiwi"
False
>>> ord("a")
97
>>> ord("b")
98
>>> ord("c")
99
>>> ord("d")
100
>>> chr(100)
'd'
>>> chr(10000)
'✐'
>>> chr(20000)
'丠'
>>> chr(2000000)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: chr() arg not in range(0x110000)
>>> "apple" < "apples"
True
link::https://www.asciitable.com/[ASCII Table]
Logical operators
Syntax:
<bool expr1> and <bool expr2>
<bool expr1> or <bool expr2>
not <bool expr>
-
and
is True only when <exp1> and <expr2 are True. "If you are over 16 and have a license, you can drive" -
or
is True if either <expr1> and <expr2> is True. "Either cats or dogs are allowed on the train." -
not
flips the boolean of the expression (e.g. if <expr> is True, not <expr> is False) "I am the tallest! Not!"
Example: happy.py
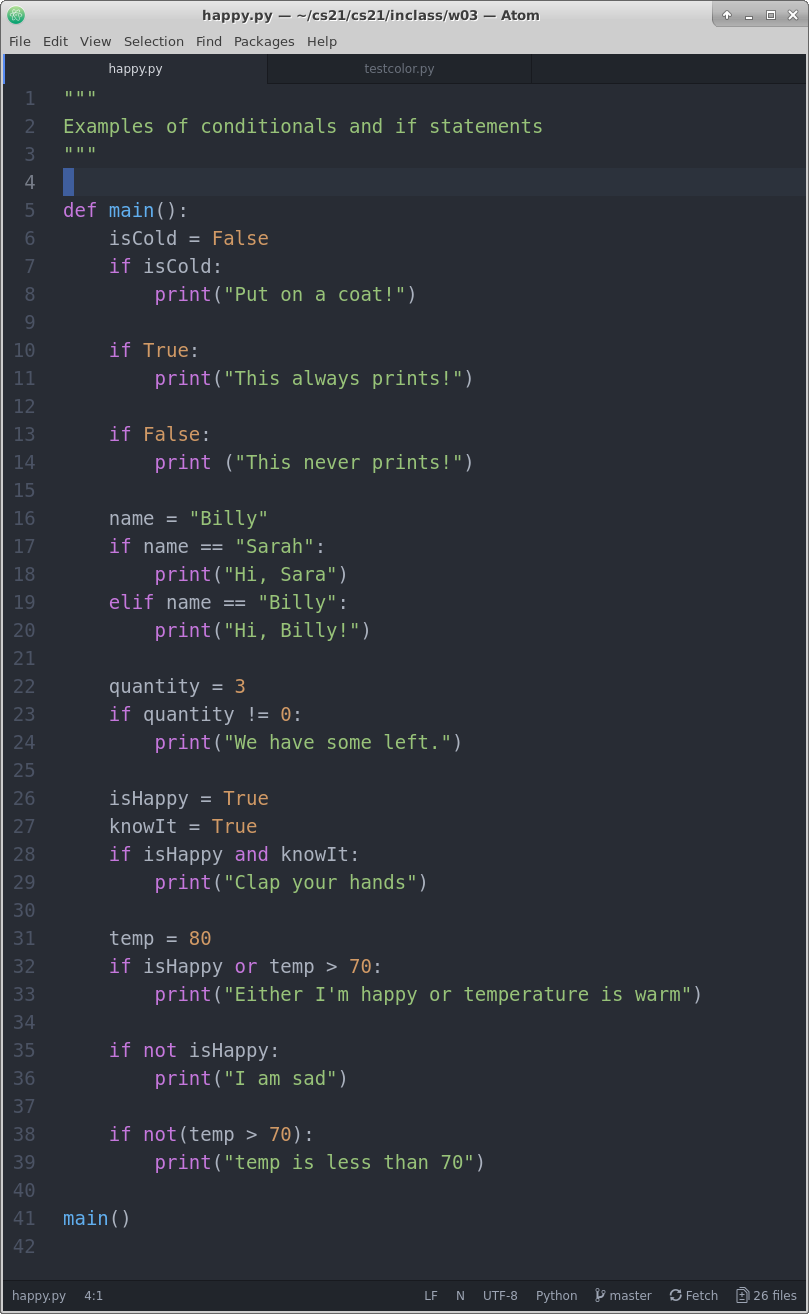
Exercise: testcolor.py
Here are several ways to write this program!
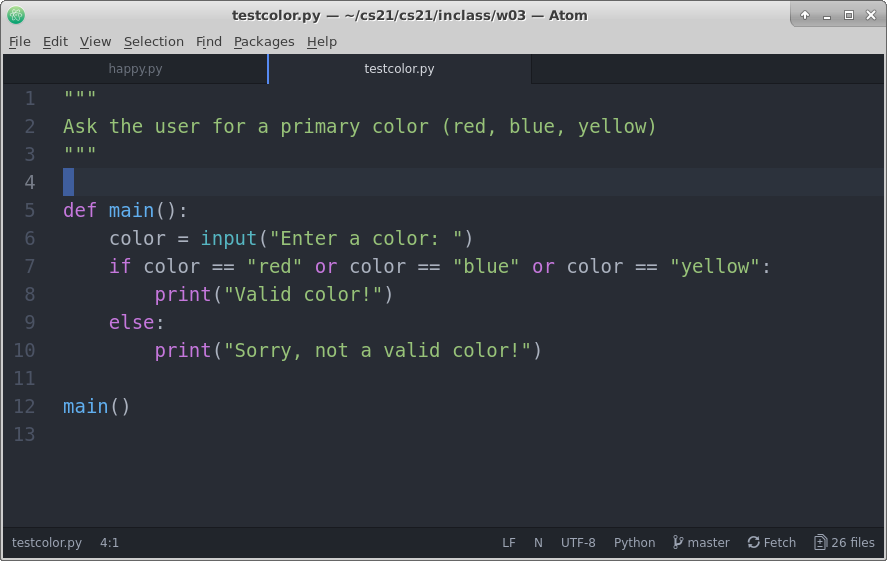
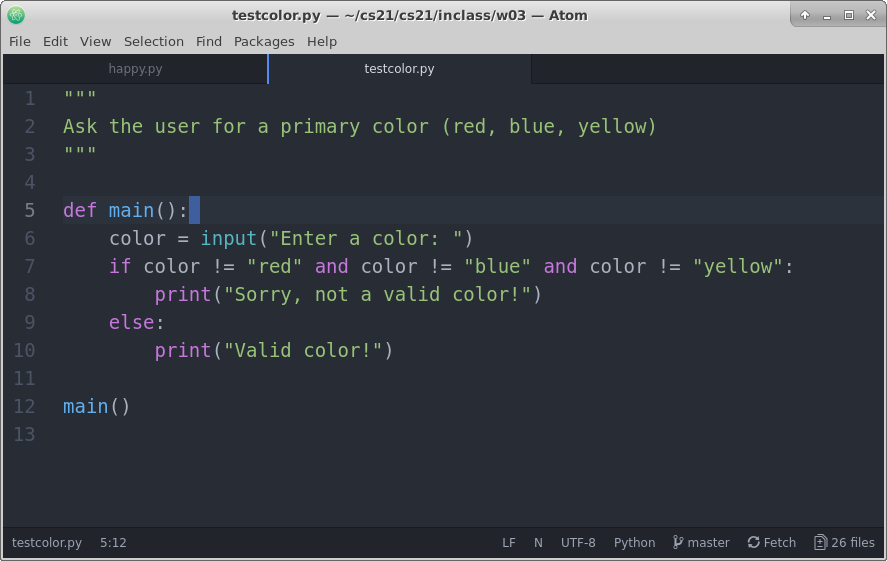
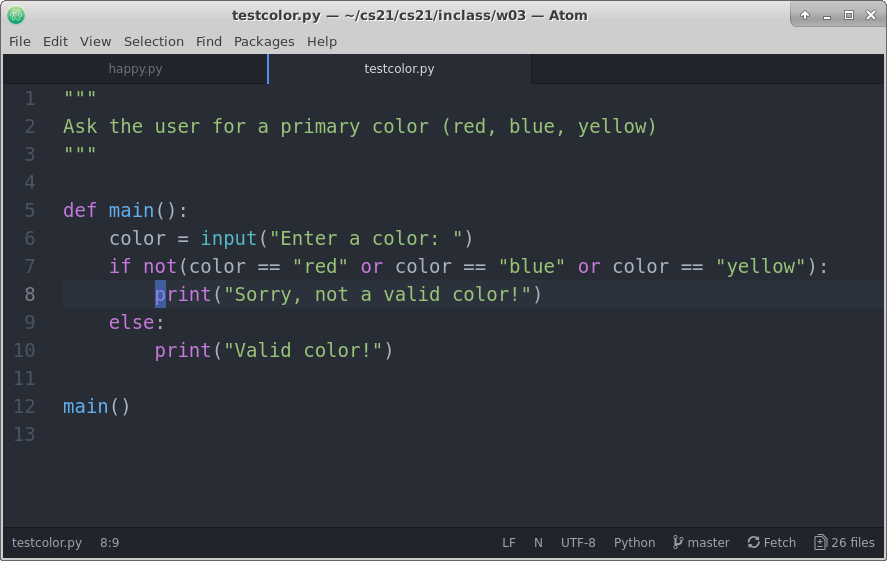
Exercise: Bug 1
The following code doesn’t execute correctly. Why?
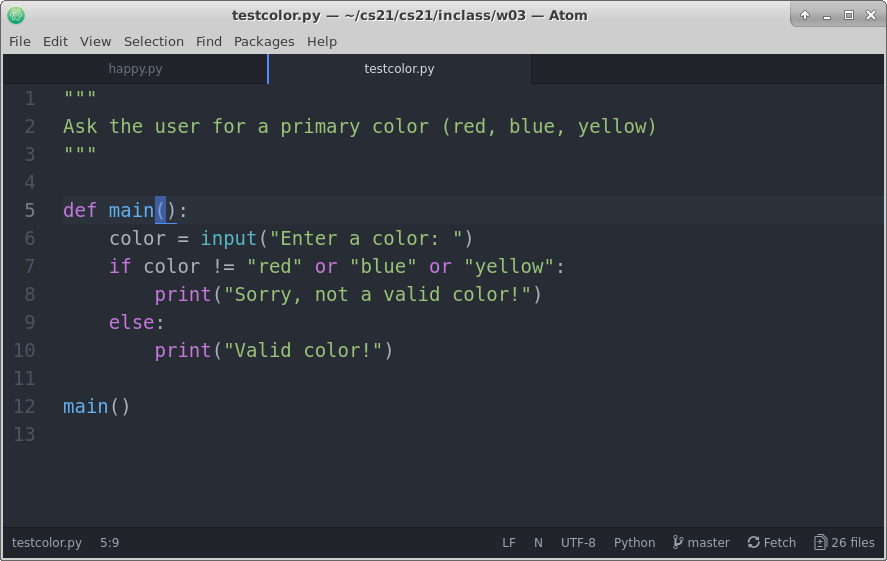
Exercise: Bug 2
The following code doesn’t execute correctly. Why?
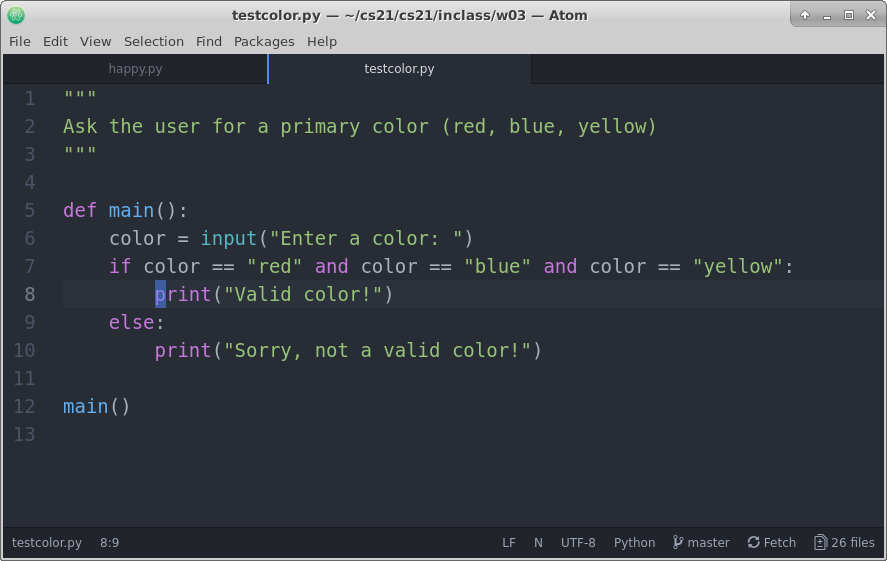
Exercise: Bug 3
The following code doesn’t execute correctly. Why?
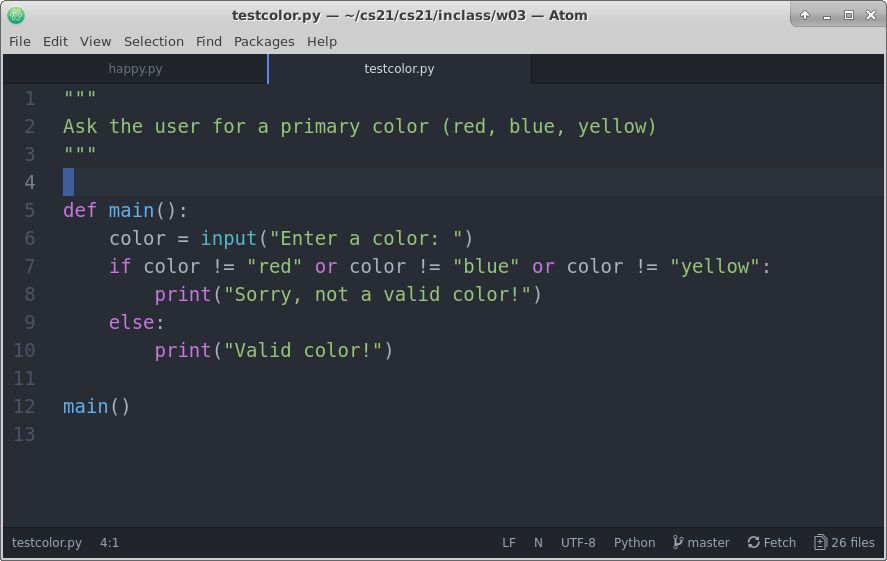
Importing modules
Modules extend the built-in functionality in Python. Without modules, we’d need to implement a lot of useful functionalities ourselves. Modules allow us to leverage code built by other people. When we learn about functions, we will take a step towards making our own re-useable code! Two useful modules are
-
math
-
random
link::https://docs.python.org/3/py-modindex.html[Index of all Python modules]
math
To use a module, we must import it into our program. How we import the module affects how we reference the module’s symbols in our code.
Syntax:
import <module_name> # method 1
from <module_name> import <symbols> # method 2
Demo:
If we say import math
, we must prepend the module name to use symbols (e.g.
functions and variables) defined in it.
pimento[~]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> import math
>>> r = 2
>>> print(math.pi * r * r)
12.566370614359172
If we say from math import pi
, we can use the variable pi
directly.
pimento[~]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from math import pi
>>> print(pi * r * r)
12.566370614359172
We can import all symbols from a module with *
, e.g. from math import *
pimento[~]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from math import *
>>> sin(pi)
1.2246467991473532e-16
random
We will use three useful functions from the random module
-
randrange(<start>, <end>)
which generates random integers between <start> and <end>-1 (likerange
) -
choice(<sequence>)
which generate a random member from <sequence> -
random()
which generates a random float between 0.0 and 1.0
pimento[~]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> import random
>>> random.random()
0.9016097746776163
>>> random.random()
0.0003006488342669478
>>> random.random()
0.8610908502580705
>>> random.random()
0.060160433647061695
>>> random.random()
0.9268107119044854
>>> random.random()
0.2297457902726423
>>> random.randrange(0, 7)
1
>>> random.randrange(0, 7)
2
>>> random.randrange(0, 7)
1
>>> random.randrange(0, 7)
3
>>> random.randrange(0, 7)
1
>>> random.randrange(0, 7)
3
>>> random.randrange(0, 7)
2
>>> random.randrange(0, 7)
6
>>> random.choice("hello friends")
' '
>>> random.choice("hello friends")
'e'
>>> random.choice("hello friends")
'e'
>>> random.choice("hello friends")
'd'
>>> random.choice("ABCDEF")
'A'
>>> random.choice("ABCDEF")
'C'
>>> random.choice("ABCDEF")
'C'
>>> random.choice("ABCDEF")
'D'
>>> random.choice([0,3,5,-6])
-6
>>> random.choice([0,3,5,-6])
3
>>> random.choice([0,3,5,-6])
3
>>> random.choice([0,3,5,-6])
5
>>> random.choice(range(4))
1
>>> random.choice(range(4))
0
>>> random.choice(range(4))
3
>>> random.choice(range(4))
1
>>> random.choice([0.4, 5.6])
0.4
>>> random.choice([0.4, 5.6])
5.6
>>> random.choice([0.4, 5.6])
0.4
>>> random.choice([0.4, 5.6])
0.4
Exercise: guess.py
We can nest if statements into for loops (and vice versa).
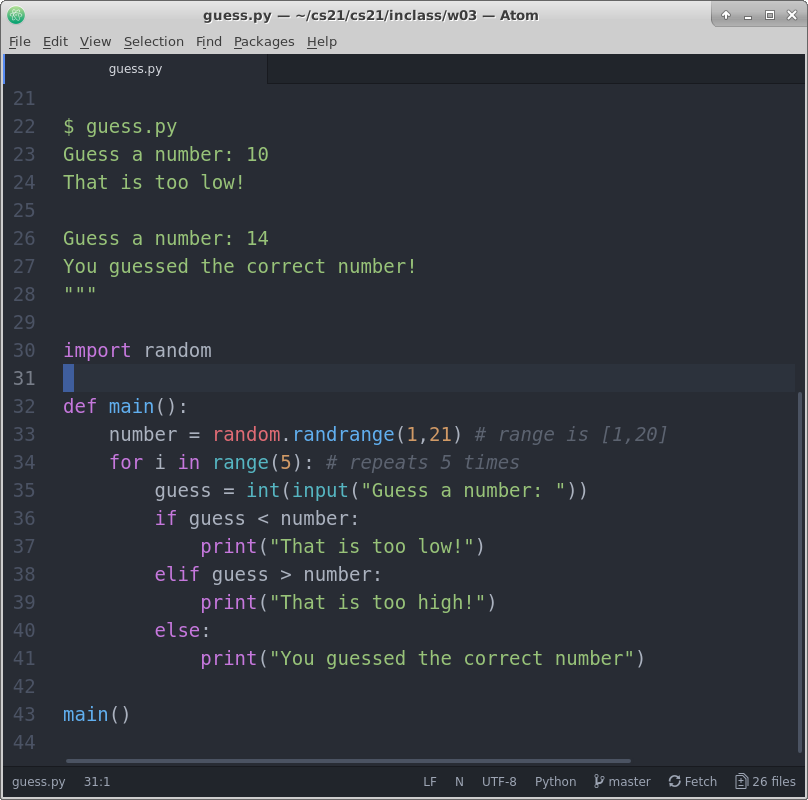