Handout1: Types and variables
We can use the Python interpreter to check our work
pimento[w02]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> type("0.0")
<class 'str'>
>>> type(0.0)
<class 'float'>
>>> a = 0.0
>>> type(a)
<class 'float'>
>>> 4
4
>>> type(4)
<class 'int'>
>>> type(-4.5)
<class 'float'>
>>> -4.5
-4.5
>>> "6.6"
'6.6'
>>> 1 + 3.0
4.0
>>> type(1 + 3.0)
<class 'float'>
>>> "cup" + "cake"
'cupcake'
>>> type("cup" + "cake")
<class 'str'>
To test whether a variable name is valid, try assigning it a value in the Python interpreter!
>>> def = 1
File "<stdin>", line 1
def = 1
^
SyntaxError: invalid syntax
>>> h = 1
>>> h
1
>>> Amount# = 1
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'Amount' is not defined
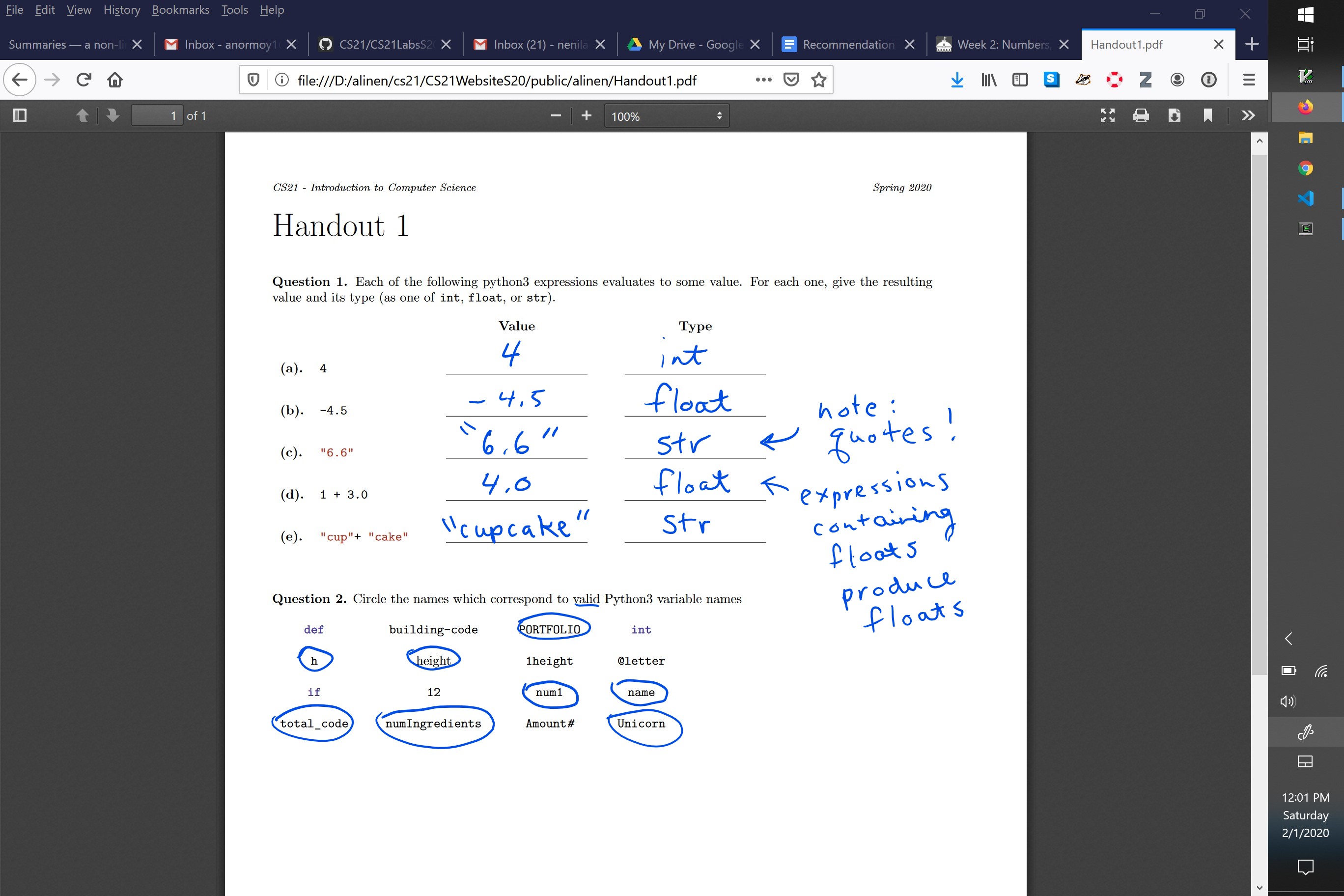
Operator precedence
Terms to know (see reading):
-
operator
-
operand
-
statement
-
expression
Rules
-
operands with higher precedence are evaluated first
-
operands with same precedence are evaluated left to right
-
if you’re not sure, use ()
highest |
() |
** |
|
/,//,*,% |
|
lowest |
+,- |
Examples:
-
4 * 2 + 3 = 11
-
4 * (2 + 3) = 20
-
"c" + "b" * 3 = "cbbb"
-
("c" + "b") * 3 = "cbcbcb"
Exercise:
-
1 + 1 ** 5 - 2 = 0
-
(1 + 1) ** (5 - 2) = 8
The data type of a variable is determined by the value stored in the variable. When evaluating expressions involving variables, we first substitute the variable with its value and then compute the operations. |
Exercise: inchesToFeet.py
What is the output of the following program?
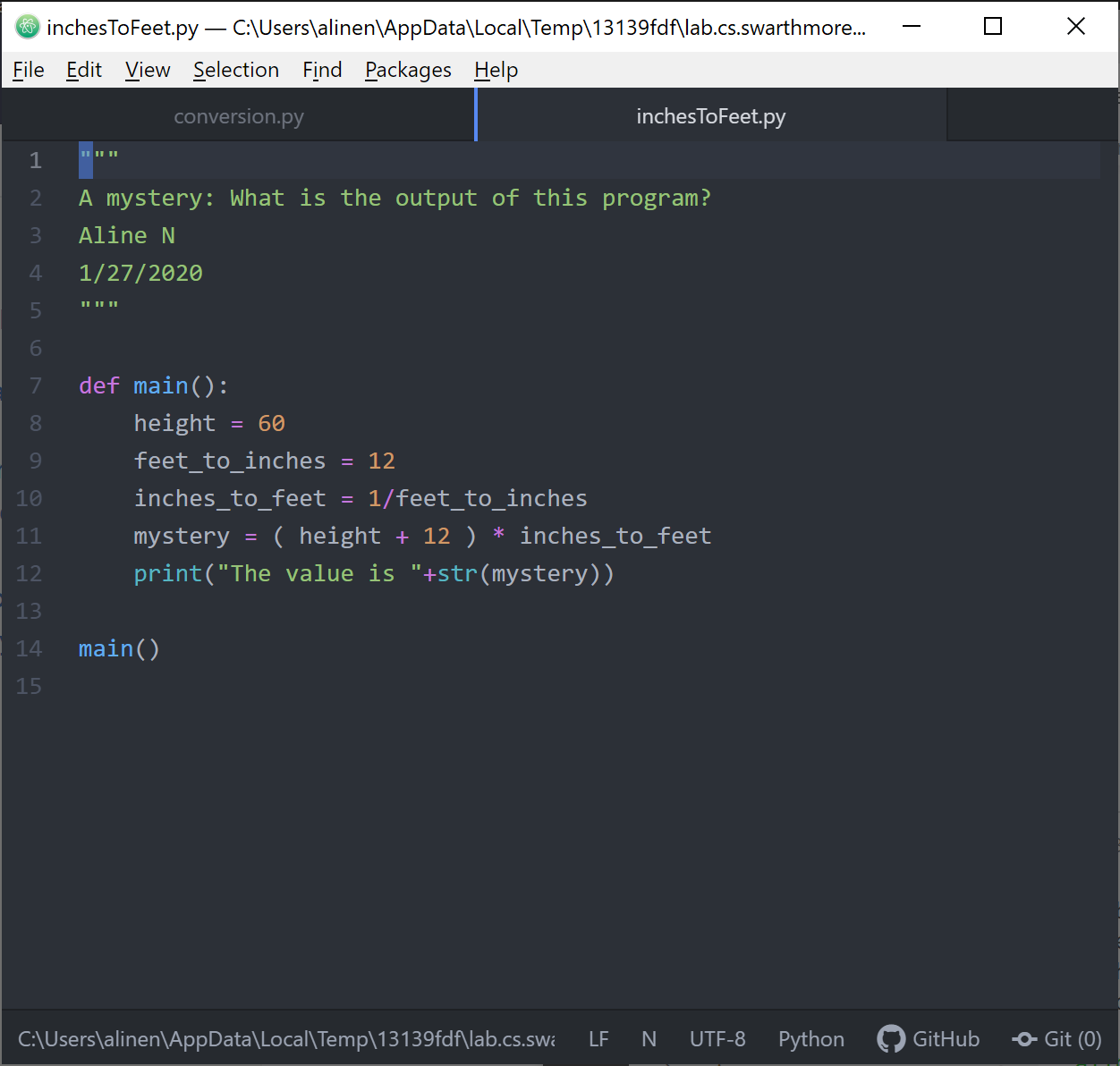
The program defines 4 variables
variable |
type |
value |
height |
int |
60 |
feet_to_inches |
int |
12 |
inches_to_feet |
float |
1/12 (e.g. 0.08333) |
mystery |
float |
(60 + 12) * (1/12) = 72/12 = 6.0 |
$ python3 inchesToFeet.py
The value is 6.0
String indexing and len()
-
indexing allows us to get a single character
-
valid indexes for a string range from 0 to len-1
-
indexes represent the offset from the start of the string. The first character is 0 offset from the start.
-
indexes out of the range are invalid and result in an error:
IndexError: string index out of range
-
indexes must be integers!
-
-
the len() function tells us the length of a string
pimento[w02]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> name = "Mr. Potato"
>>> name
'Mr. Potato'
>>> name[0]
'M'
>>> name[1]
'r'
>>> name[2]
'.'
>>> name[3]
' '
>>> name[4]
'P'
>>> len(name)
10
>>> name[10]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: string index out of range
>>> name[0]
'M'
>>>
Exercise: requestChar.py
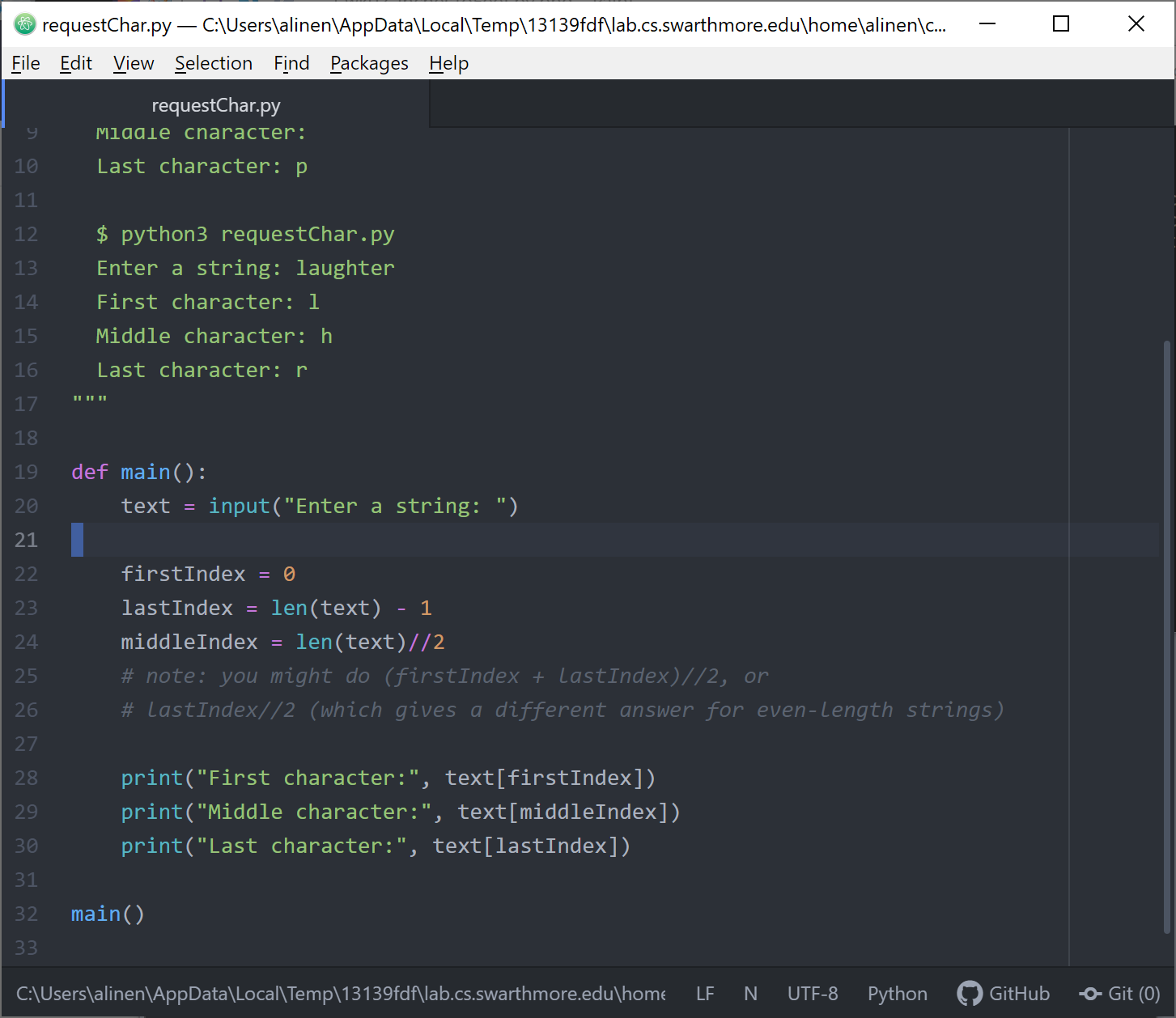
Lists
Lists are a data type for storing multiple values. Indexing for lists
work similary to strings. Lists also support the +
and *
operators
for concatenation and repetition.
pimento[w02]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> values = [0, 1, 5, -4]
>>> values
[0, 1, 5, -4]
>>> values[0]
0
>>> num_values = len(values)
>>> num_values
4
>>> values[num_values-1]
-4
>>> values[10]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: list index out of range
>>> values = [9.0, "cat", 3, 5]
>>> values
[9.0, 'cat', 3, 5]
>>> values[0]
9.0
>>> values[1]
'cat'
>>> values = []
>>> len(values)
0
>>> values = [] + [4,5]
>>> values
[4, 5]
>>>
for loops
Our programs will often need to repeat instructions. For example, suppose we would like to print every character from a word on its own line.
for <var> in <sequence>:
<body>
-
<var> variable which stored the current value of the iteration
-
<sequence> the set of values to iterate over
-
<body> one or more statements to be repeated
def main()
for i in range(5):
print(i) # this line is repeated 5 times!
main()
In the above example, range(5)
gives us the sequence of values
0,1,2,3,4.
iteration |
i |
1 |
0 |
2 |
1 |
3 |
3 |
4 |
3 |
5 |
4 |
range()
The range function generates sequences of integers. Range can be called with 1, 2, or 3 arguments
range(<end>) # one argument
range(<start>, <end>) # two arguments
range(<start>, <end>, <step>) # three arguments
-
<end> the last integer in the sequence will be <end>-1
-
<start> the first integer in the sequence
-
<step> the increment between values
Demo:
In the Python interpreter, we can convert range to a list to display the sequence of values. |
pimento[w02]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> range(5)
range(0, 5)
>>> list(range(5))
[0, 1, 2, 3, 4]
>>> list(range(0, 5))
[0, 1, 2, 3, 4]
>>> list(range(1, 5))
[1, 2, 3, 4]
>>> list(range(2, 10))
[2, 3, 4, 5, 6, 7, 8, 9]
>>> list(range(2, 10, 2))
[2, 4, 6, 8]
>>> list(range(10, 2))
[]
>>> list(range(10, 2, -1))
[10, 9, 8, 7, 6, 5, 4, 3]
>>>
Example: letters.py
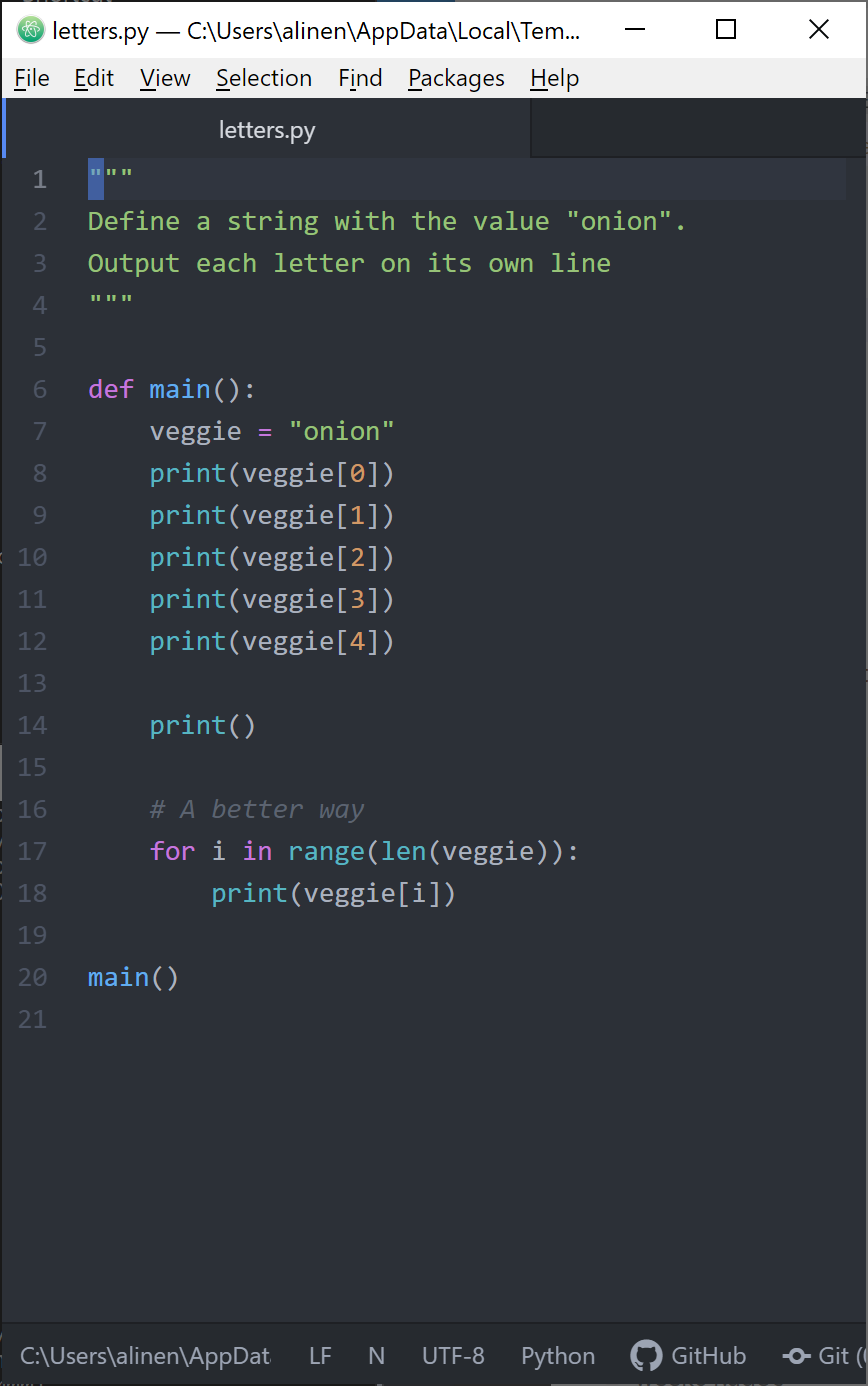
Handout2: loops
We can write Python code to check out work!
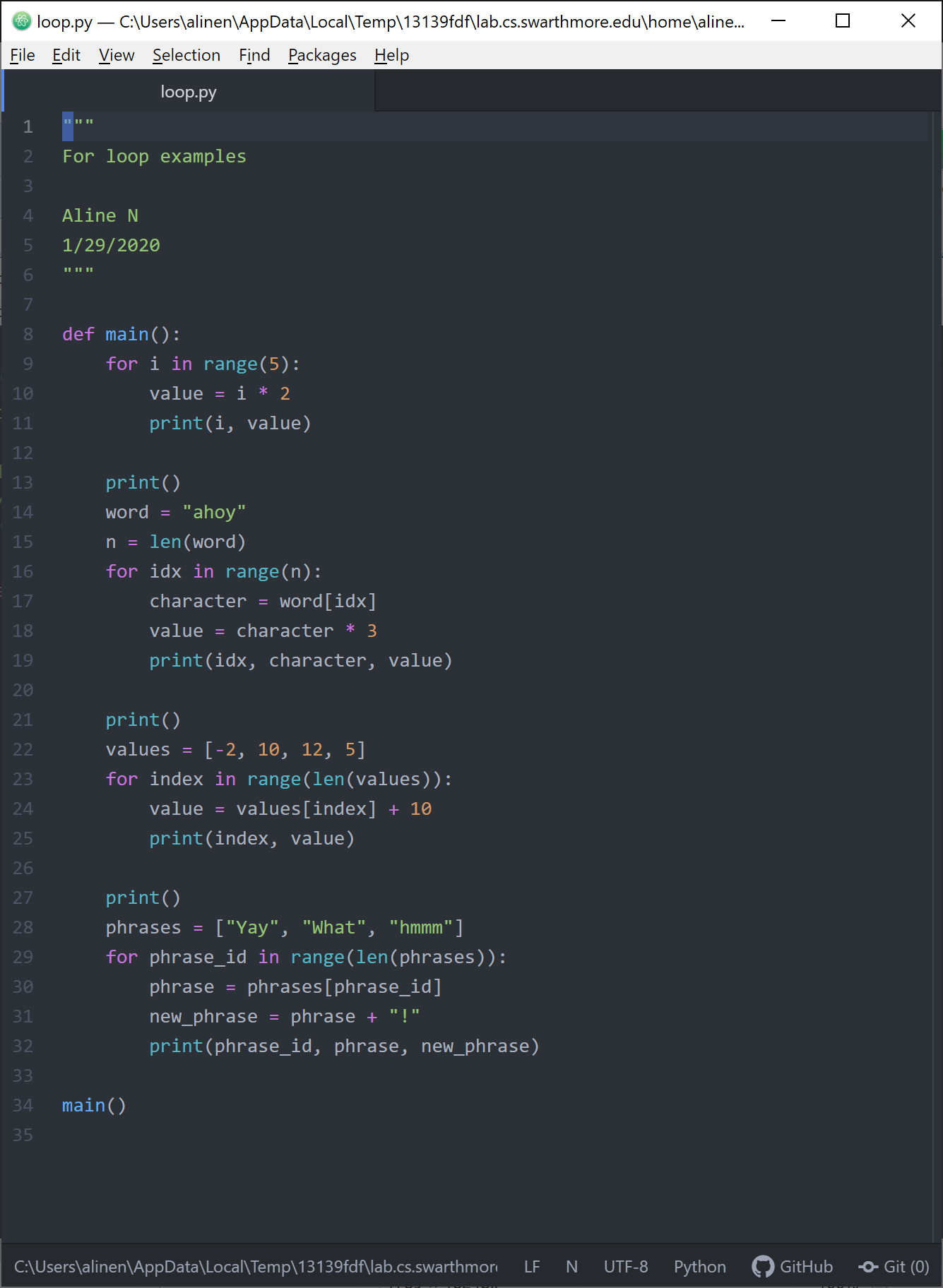
cheese[w02]$ python3 loop.py
0 0
1 2
2 4
3 6
4 8
0 a aaa
1 h hhh
2 o ooo
3 y yyy
0 8
1 20
2 22
3 15
0 Yay Yay!
1 What What!
2 hmmm hmmm!
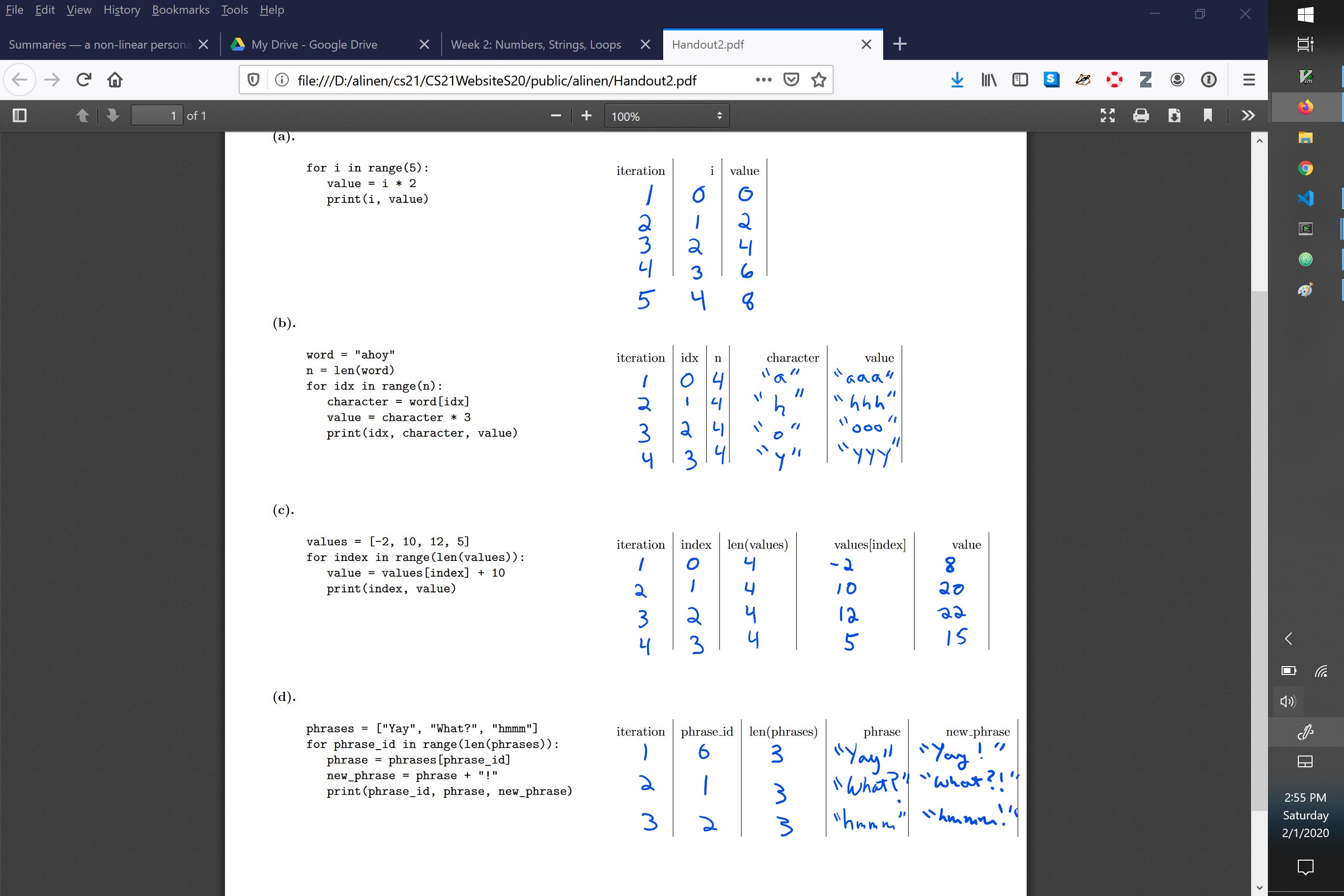
Example: countdown.py
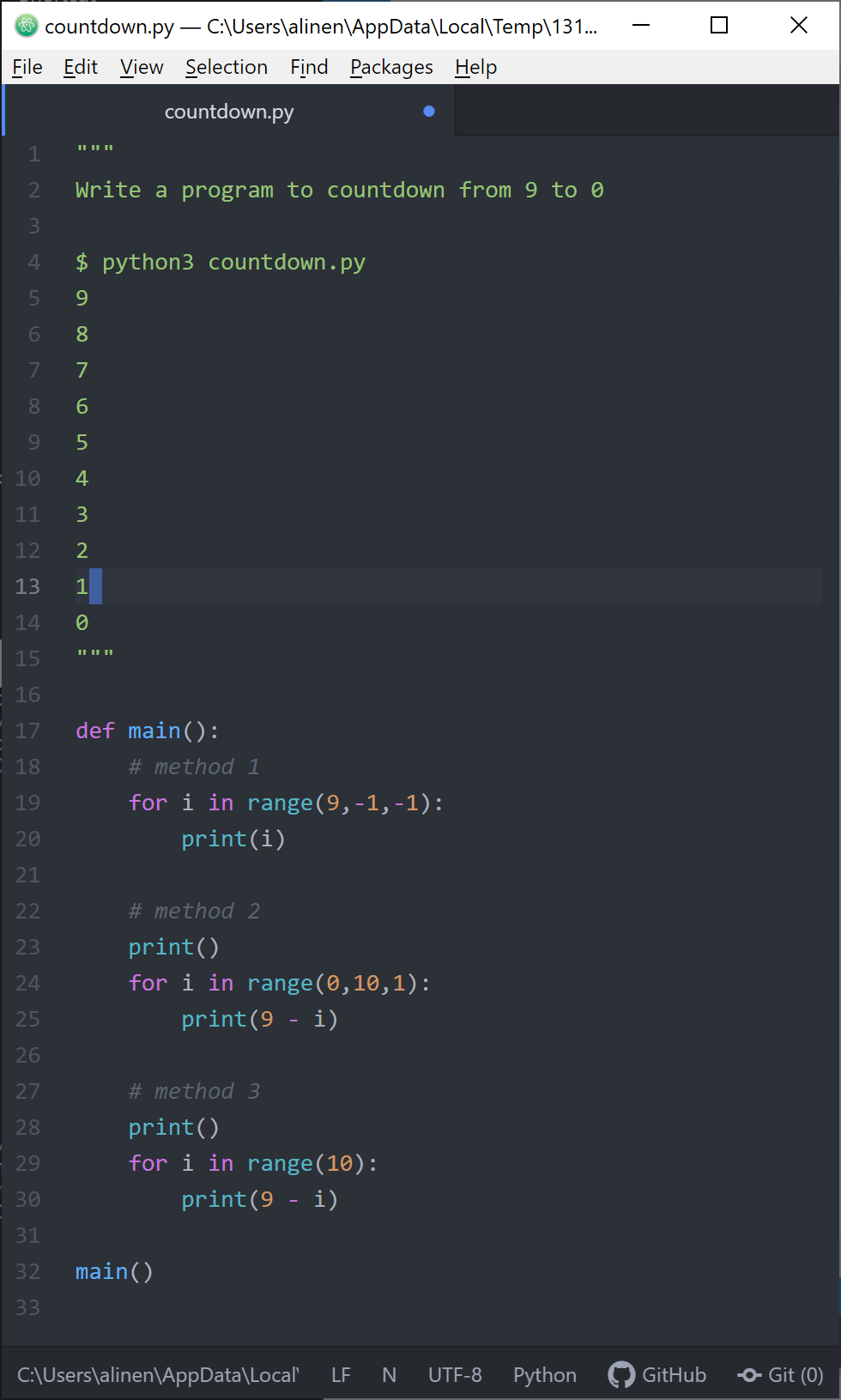
Example: square.py
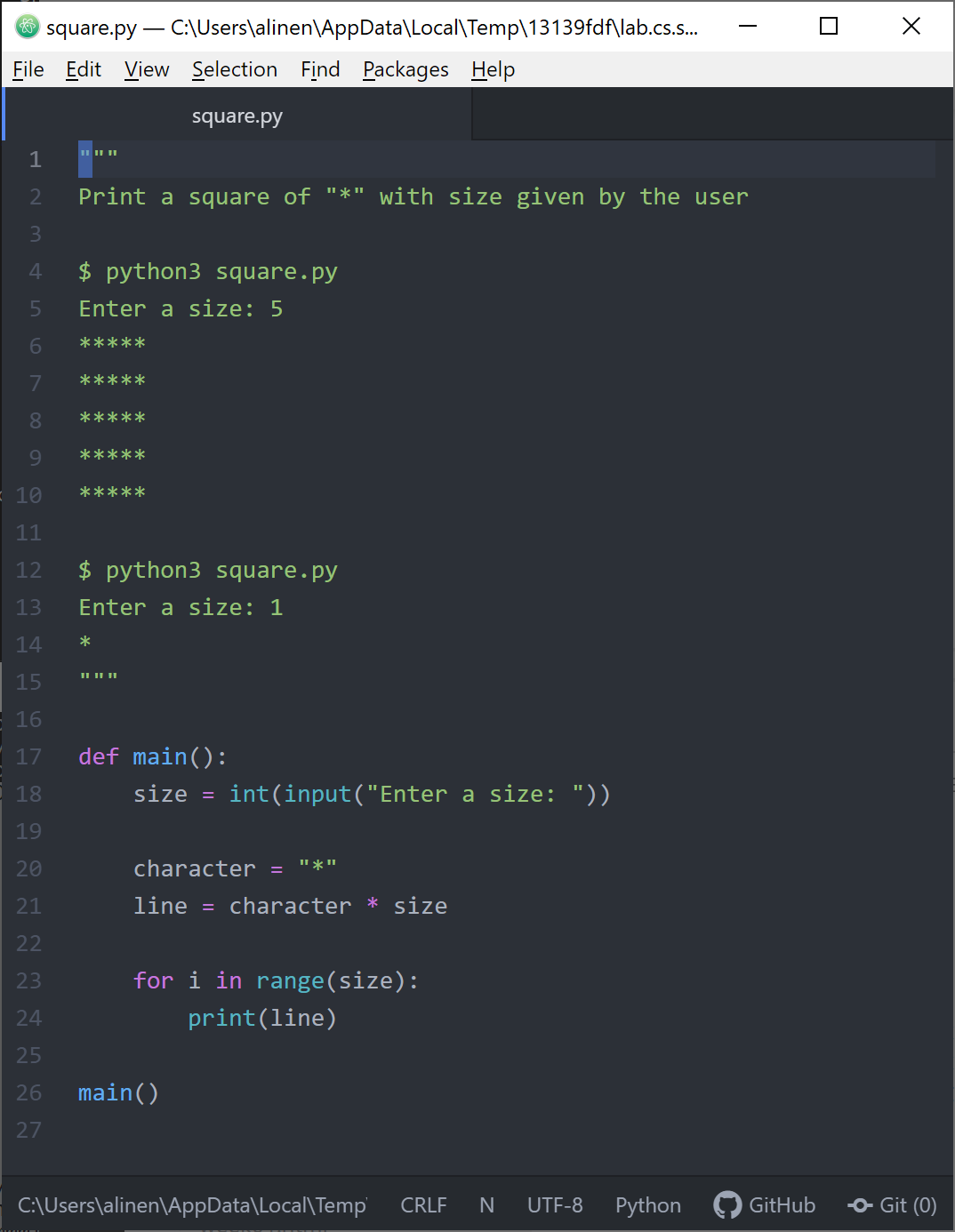
Accumulator pattern
The accumulator pattern allows us to update a variable using a loop. For example, imagine you are computing a total for a sequence of values without writing down values. A good way to do it is to remember the total so far and then update it with each new value. This is how accumulators work in code.
initialize the accumulator value
loop until done
update accumulator
Example: total.py
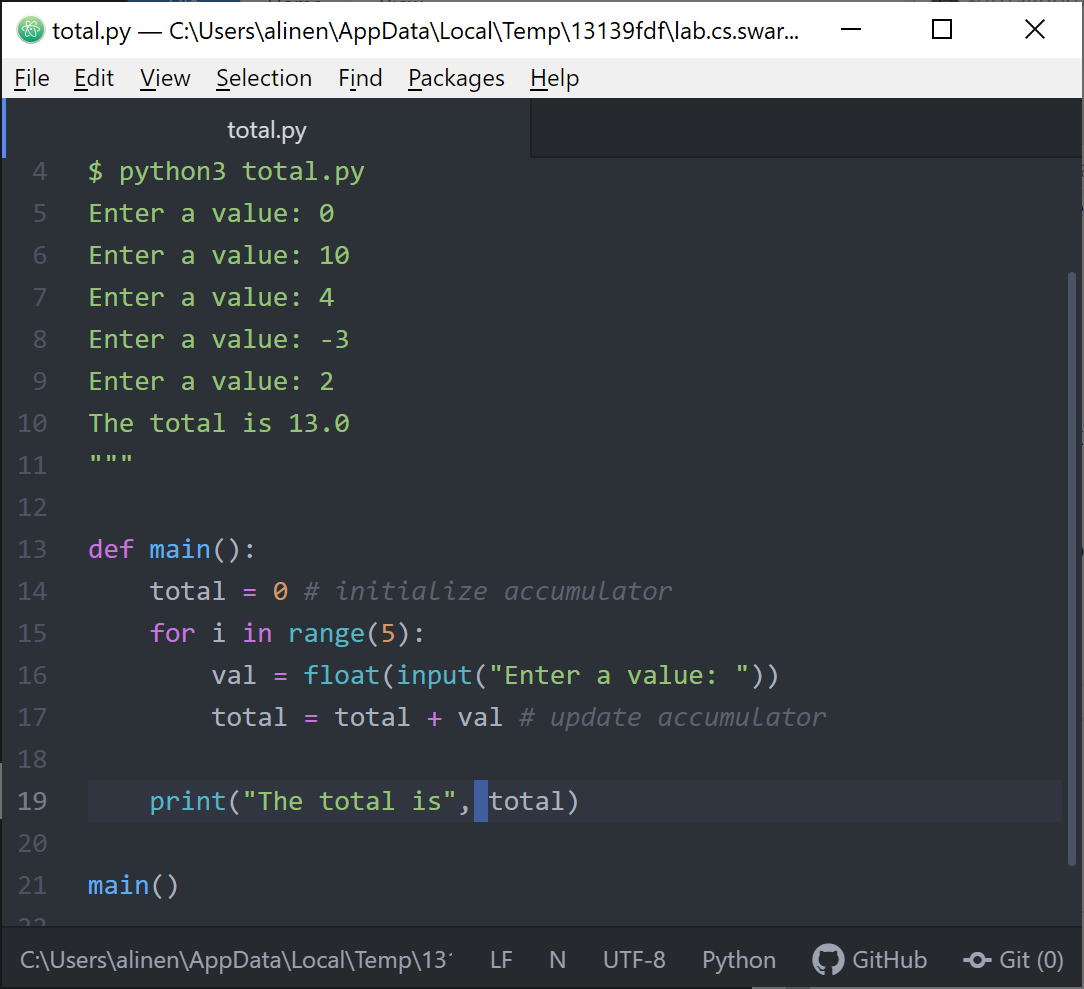
In the table below, assume the user gives the values 0, 10, 4, -3, and 2. Note that total starts with value 0.
iteration |
i |
val |
total |
1 |
0 |
0 |
0 |
2 |
1 |
10 |
10 |
3 |
3 |
4 |
14 |
4 |
3 |
-3 |
11 |
5 |
4 |
2 |
13 |
Example: countup-string.py
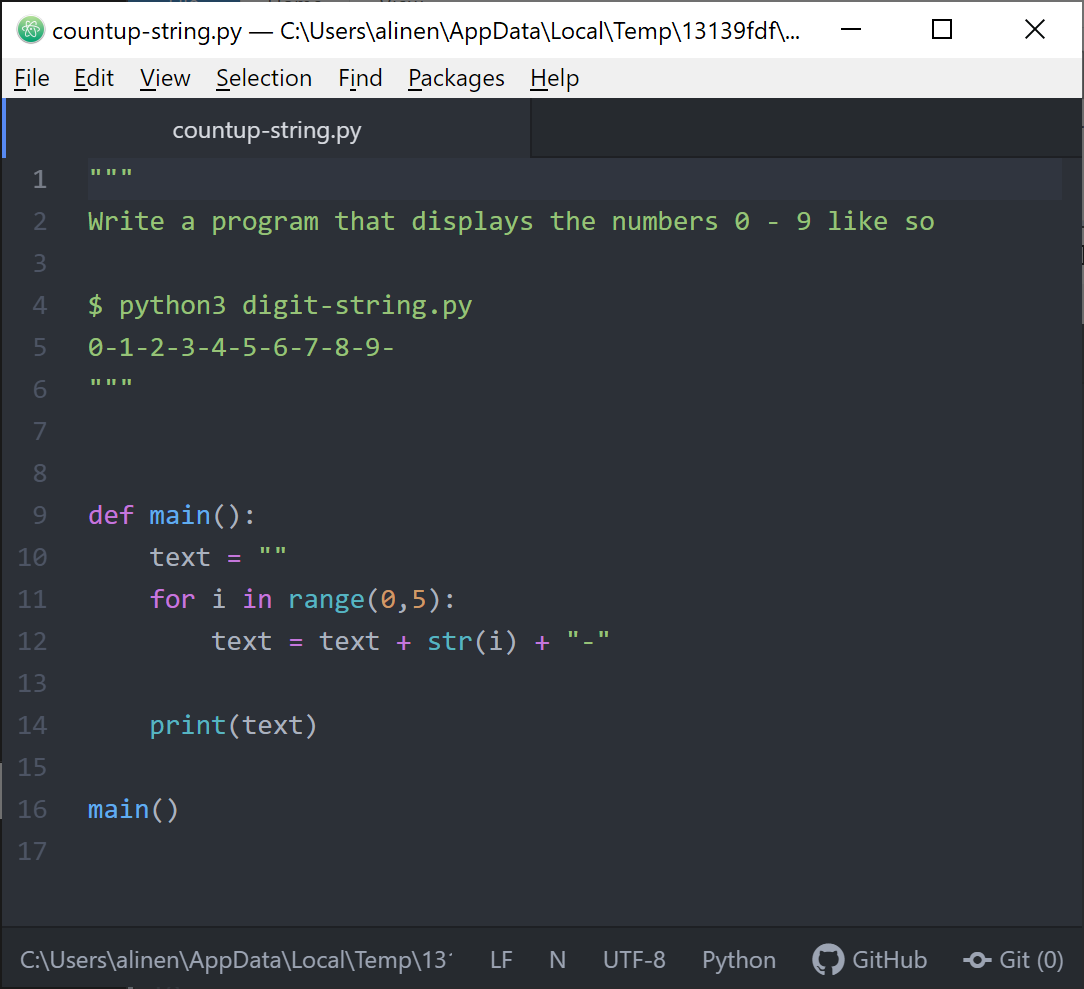
iteration |
i |
text |
1 |
0 |
"0-" |
2 |
1 |
"0-1-" |
3 |
3 |
"0-1-2-" |
4 |
3 |
"0-1-2-3-" |
5 |
4 |
"0-1-2-3-4-" |
Example: pies.py
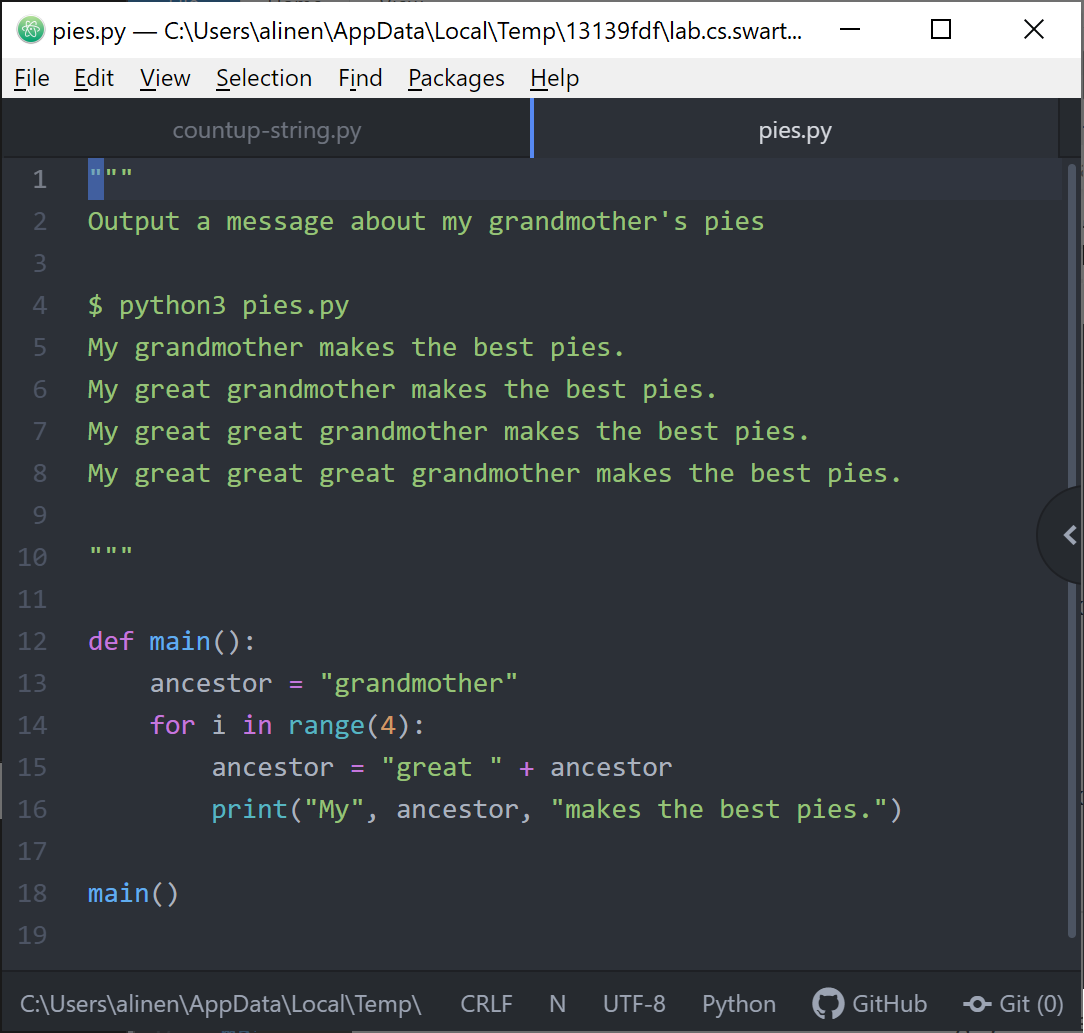
iteration |
i |
printed phrase |
ancestor |
1 |
0 |
"My grandmother makes the best pies." |
"great grandmother" |
2 |
1 |
"My great grandmother makes the best pies." |
"great great grandmother" |
3 |
2 |
"My great great grandmother makes the best pies." |
"great great great grandmother" |
4 |
3 |
"My great great great grandmother makes the best pies." |
"great great great great grandmother" |
Exercise: average.py
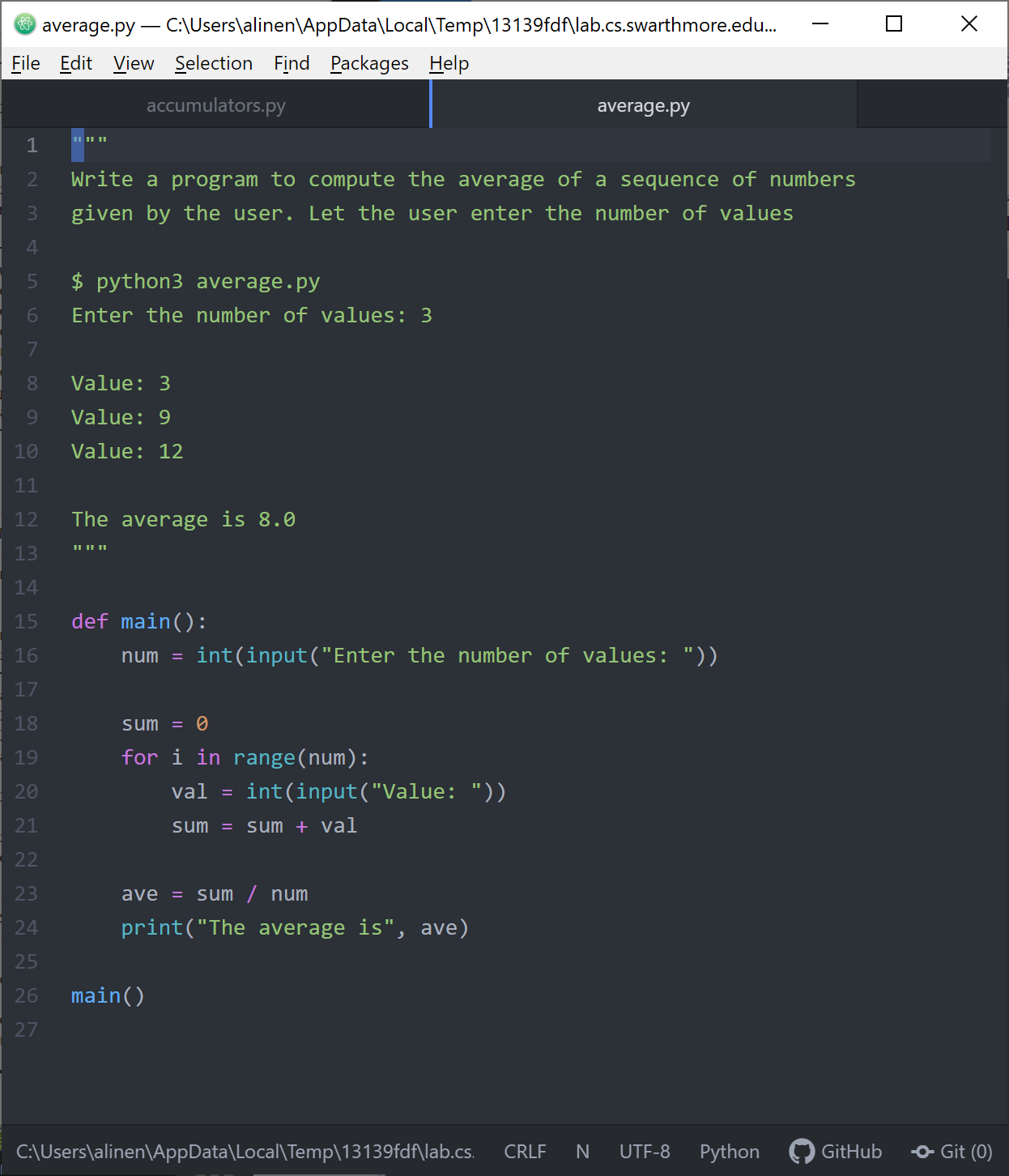
Exercise: banner.py
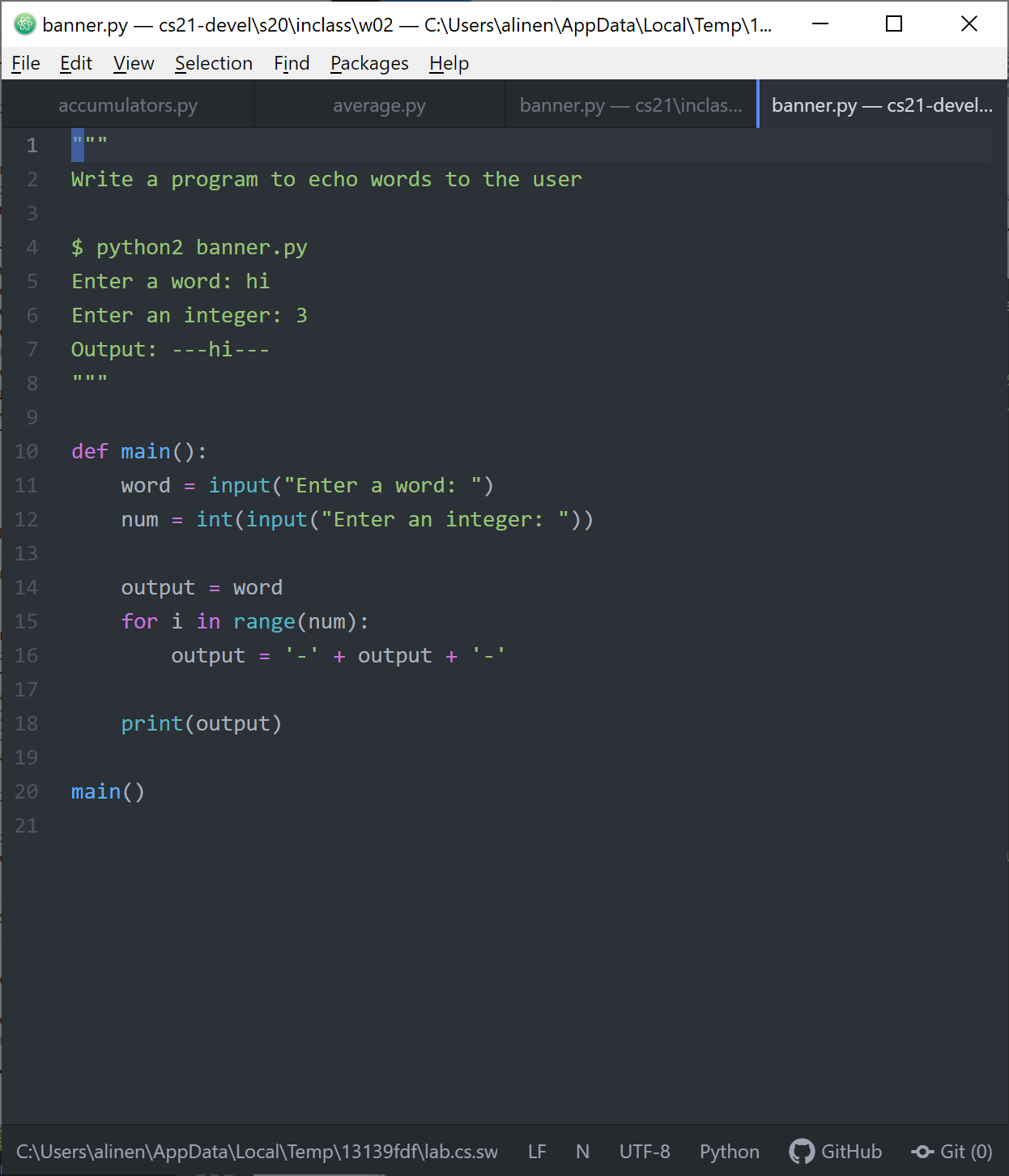
Exercise: factorial.py
There are many ways to implement factorial!
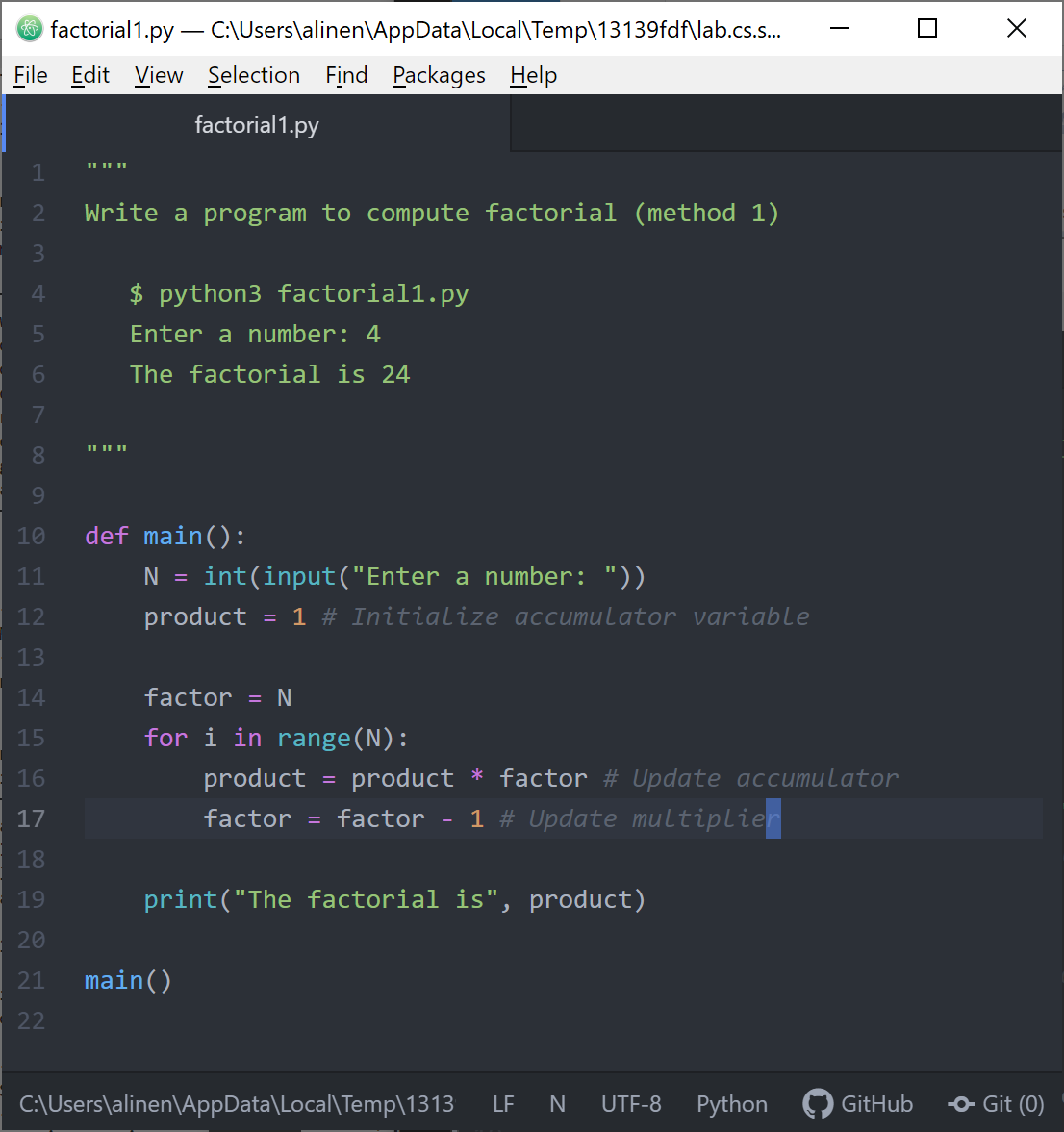
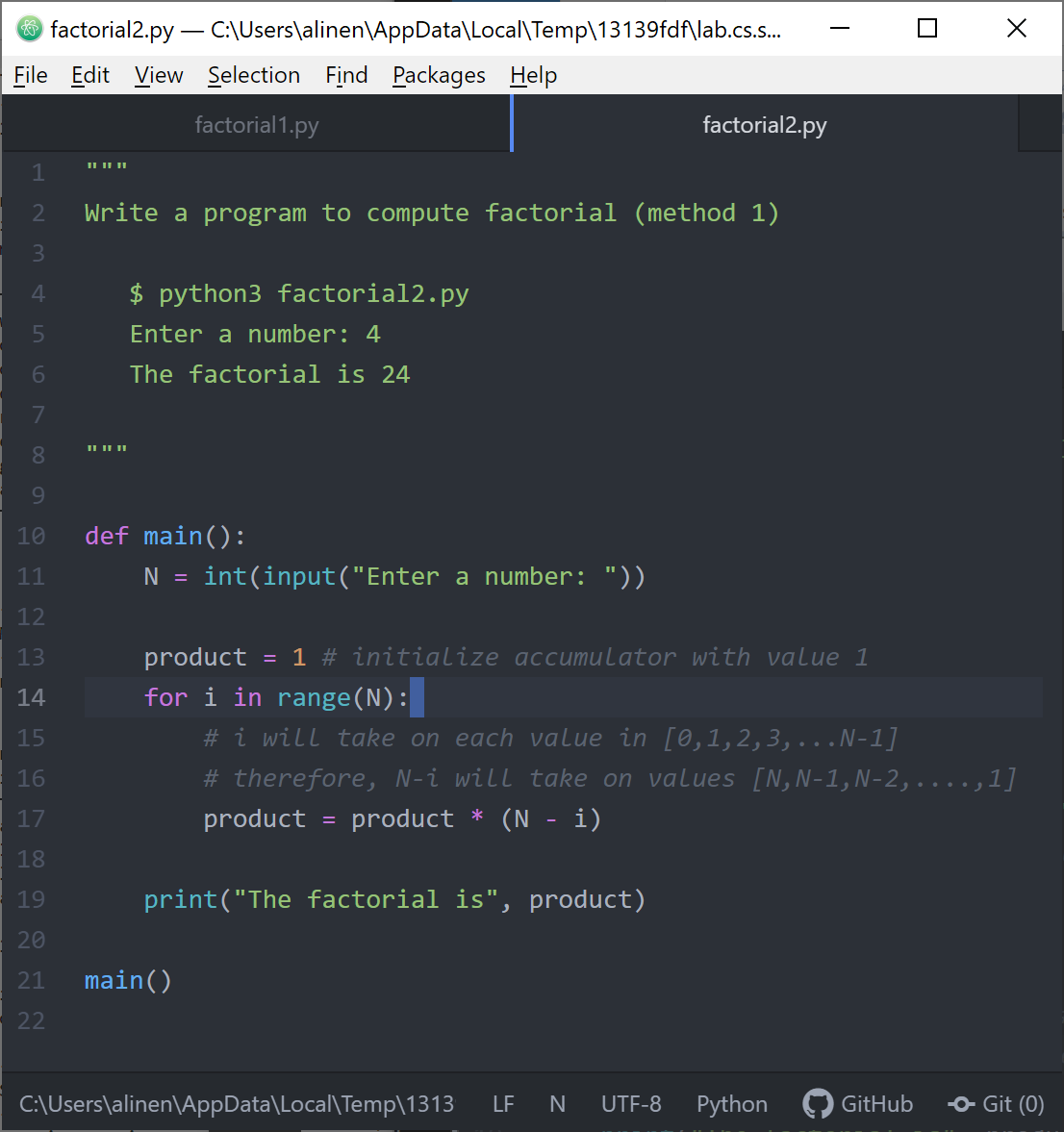
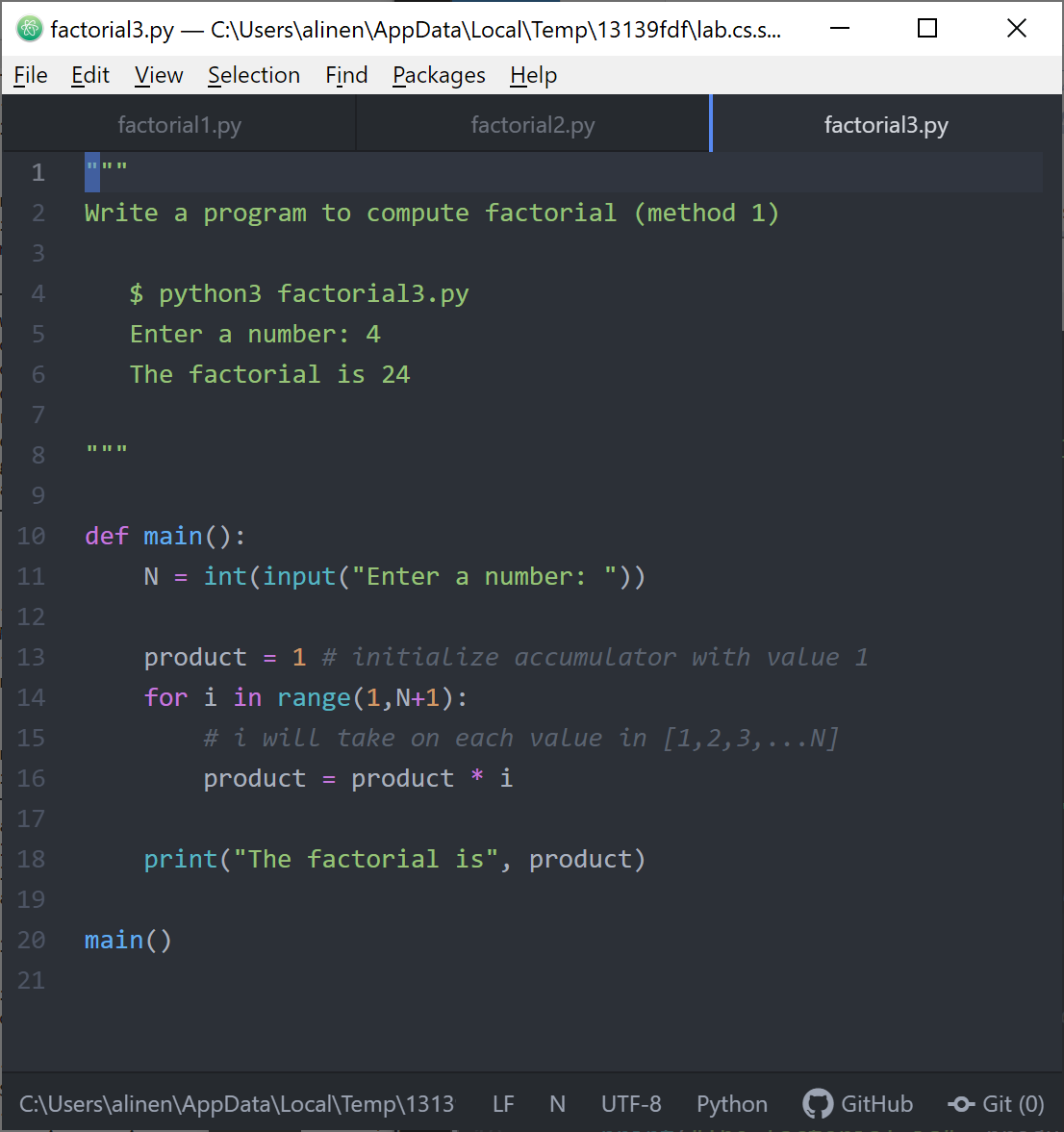
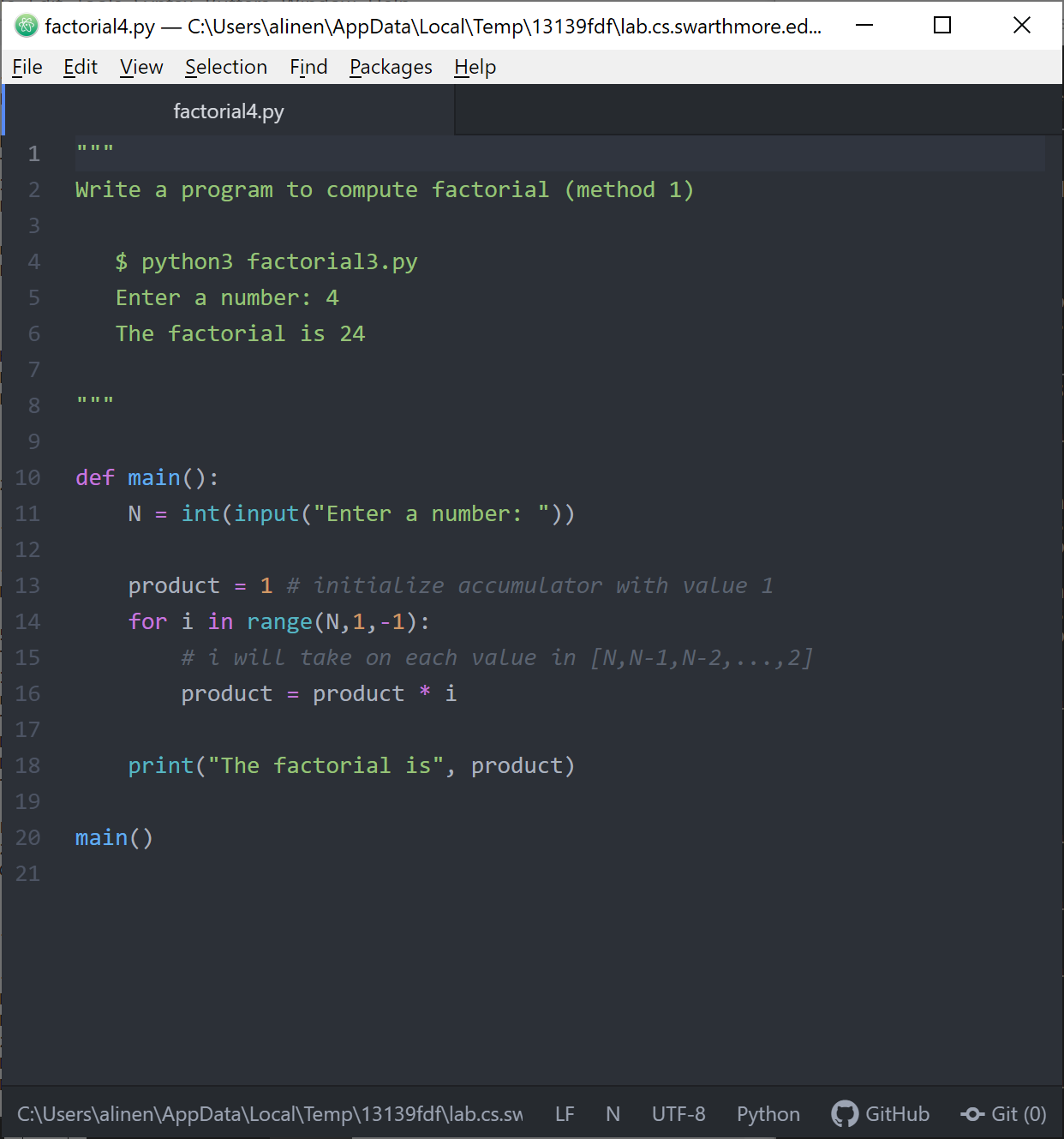
Handout3: accumulator
Again, we can check our answers using code and the Python interpreter!
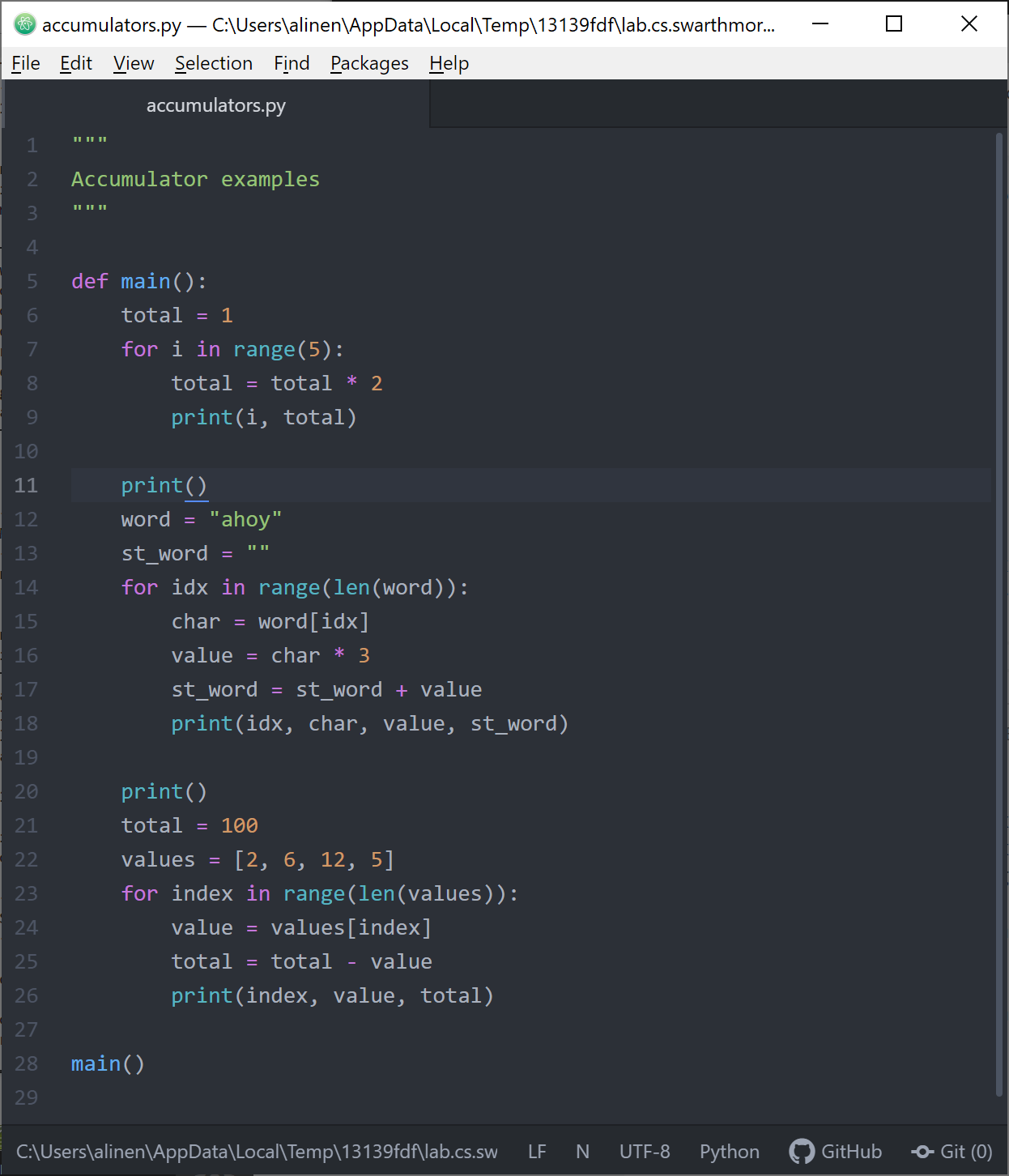
pimento[w02]$ python3
Python 3.6.9 (default, Nov 7 2019, 10:44:02)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> list(range(5,10))
[5, 6, 7, 8, 9]
>>> list(range(5,1,-1))
[5, 4, 3, 2]
>>> list(range(0, 15,3))
[0, 3, 6, 9, 12]
>>> list(range(5,5))
[]
>>> list(range(5,2))
[]
>>> list(range(5,2, -1))
[5, 4, 3]
>>>
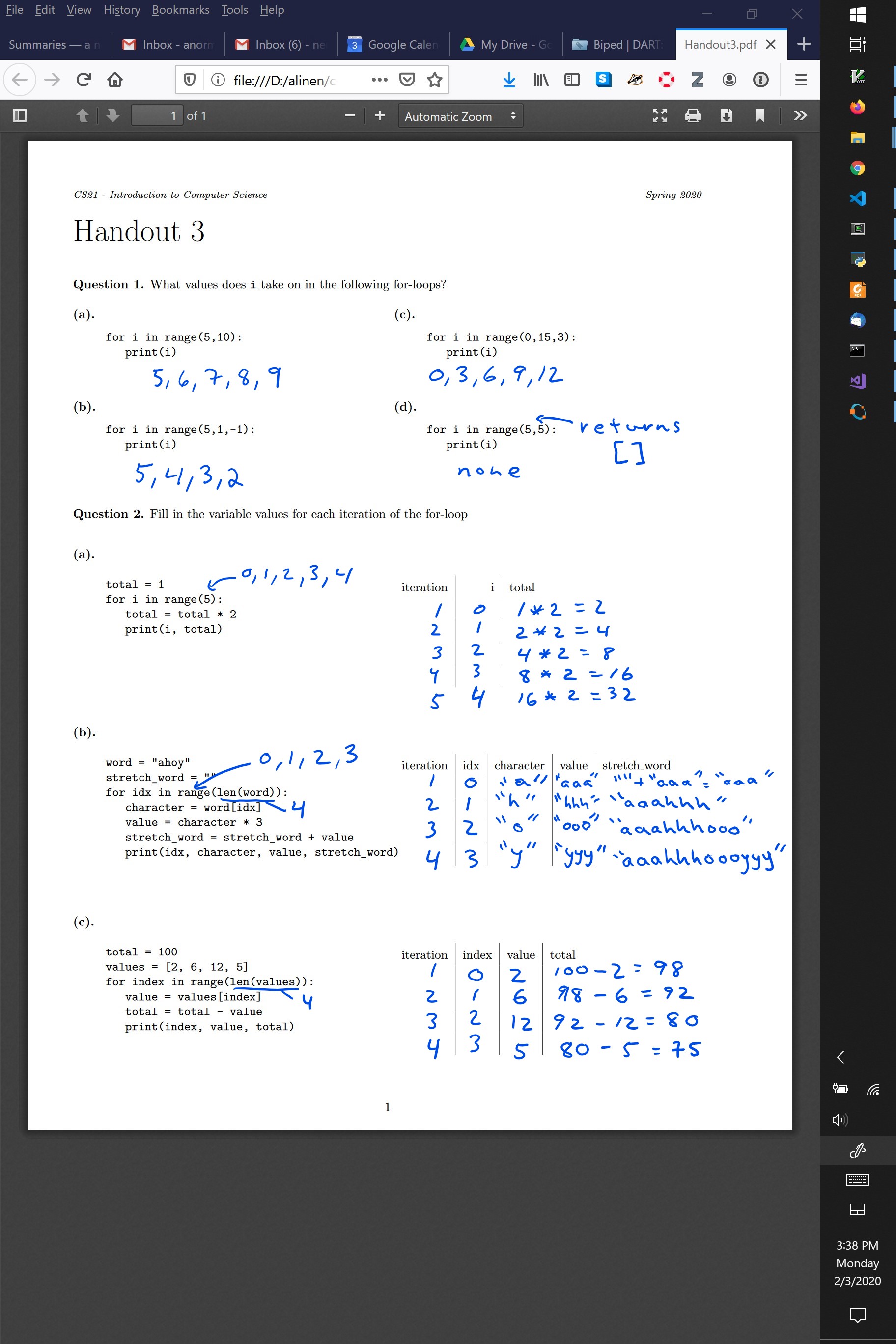