CS21 Lab 6: Spelling Bee (TDD)
Design is due Saturday, October 24, before midnight
Full Implementation is due Saturday, October 31, before midnight
Goals
-
practice using Top-Down Design
-
write a complex program, with multiple functions, from scratch
Notes
Please read through the entire lab before starting!
This is a two-part lab, split over two weeks. For the first week you will focus on using top-down design to create the overall structure for the program. Once your proposed structure has been reviewed and approved by your professor or lab instructor, you will use bottom-up implementation to complete the full program.
You have two weeks to complete the full program. The first week is for the design, and the second for the full program. Your initial top-down design is due this Saturday (October 24) and the full implementation the following week (October 31). It is highly recommended that you submit your top-down design as soon as is possible so that we have time to give you feedback before you begin your full implementation. If you submit your design on the last day at midnight, it might take us a few days to get to it and send you comments.
Spelling Bee
In this lab, we will reimplementing a word puzzle game found on the New York Times website: Spelling Bee. You need a subscription to play the full game, but you can click the link and take one or two turns if you’d like.
To explain the game, let’s start with an example puzzle from September 30, 2020:
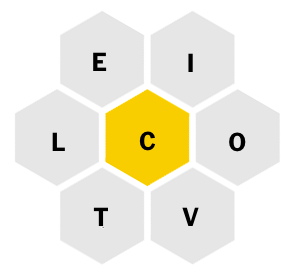
The puzzle consists of a center letter with six letters surrounding it. In the puzzle above, the letter "C" is in the center, with the letters "E", "I", "L", "O", "T", and "V" surrounding it.
Your goal is to make words using those 7 letters, earning points for each word you make. There are four basic rules you should remember when making words:
-
All words must contain the center letter
-
You can reuse a letter in the puzzle as many times as you want
-
All words must be at least 4 letters long
-
All words must be found in a dictionary that we will provide you
-
You can’t make the same word more than once
Using the example above:
-
We could make the word "LICE" since it contains the center letter and is at least 4 letters long. But we could not make the word "ICE" since it is only 3 letters long. And we could not make the word "LIVE" since it doesn’t contain the center letter "C".
-
We could make the word "COOL" since it contains the center letter and is at least 4 letters long and we are allowed to reuse a letter as many times as we want. But we could not make the word "COAL" since there is no "A" in the puzzle. And we could not make "CELTIC" since that isn’t a word in the dictionary — it is a proper noun.
Each word you make will earn you points. Try to get the most possible points!
-
Each 4 letter word is worth 1 point. For example, the word "COOL" would be worth 1 point.
-
Each word longer than 4 letters is worth 1 point per letter. For example, the word "VOICE" would be worth 5 points.
Examples
Here are a few examples of the running program, to help you see how things should work. Pay attention to how the game proceeds, and how input from the player is handled. As you can see from these examples, we have modified the game a bit, since we’re using a terminal screen to display the game and not a graphical web page.
The examples above include these features:
-
Before guessing each time, the player is presented with the words they’ve already guessed. The player is not allowed to guess the same word twice.
-
If the player enters a valid word, they are told how many points they got for the turn and their current score is displayed. If the word is not valid, an informative message is displayed.
Program Resources
To help you test your program, we have provided you with two files:
-
/data/cs21/bee/miniboards.txt
contains 3 spelling bee boards. In your program, you will read in the list of boards and choose one randomly. The first letter of each line represents the center tile. The remaining tiles are the outer letters. To represent the puzzle above, theminiboards.txt
contains the string"CEILOTV"
: "C" is the center tile and remaining letters are around the outside. You can open this file in Python usingopen('/data/cs21/bee/miniboards.txt', 'r')
. -
/data/cs21/bee/miniwords.txt
contains a list of legal words that can be made from the 3 spelling bee boards inminiboards.txt
. This is a small subset of words from a scrabble dictionary. Each word is one a line by itself. You can open this file in Python usingopen('/data/cs21/bee/miniwords.txt', 'r')
.
Once you are sure things are working well, you can switch to the full set of boards and words. Do not switch to the full set of boards and words until you are sure your program works completely on the smaller files above.
-
/data/cs21/bee/boards.txt
contains a list of 62,354 spelling bee boards in the same format asminiboards.txt
. You can open this file usingopen('/data/cs21/bee/boards.txt', 'r')
. -
/data/cs21/bee/words.txt
contains a list of all of the legal words that can be made in your game. Each word is one a line by itself. You can open this file usingopen('/data/cs21/bee/words.txt', 'r')
.
Requirements
You have some freedom in how you want your game to look. Here are our requirements for the full program:
-
As part of your top-down design, your main function should have a loop that plays the game. The body of this loop should contain more than just a call to one or two functions. Instead, it should contain multiple function calls and other code that implements various parts of a single round.
-
The program should use the files above to choose a random board and get the list of legal words.
-
The player should be prompted to take their turn with an informative prompt.
-
Legal words entered by the player should be scored properly and the score should be displayed to the player.
-
The player’s updated score should be displayed regularly.
-
If a player enters an illegal word, they should be given an informative message. (Recall that illegal words are: words that don’t contain the center tile, words that contain letters that aren’t in the puzzle, words that are less than 4 letters long, and words that aren’t in the dictionary.)
-
When the game ends, display a status message saying how many points the player got.
-
All output should be clean and easy to read.
Top-Down Design Requirements
You should complete your top-down design (TDD), submit it (run handin21), and obtain feedback on it before beginning the full game implementation. Special procedures for this two-week lab:
-
create your design in
design-spellingbee.py
first -
after you have a working design (see example below), run
handin21
to turn it in! Then send a short email to tdd@cs.swarthmore.edu, letting us know your design is done. We will take a look at each design and send you comments (usually within a day or two). If the design is incomplete or insufficient, you may be asked to submit a second design. -
after you have the design done and have heard back from us, please copy the design file to
spellingbee.py
and implement the full game inspellingbee.py
(i.e., leavedesign-spellingbee.py
as is!)cp design-spellingbee.py spellingbee.py
-
please ensure your design meets the following requirements before submitting:
-
main()
should be completely written, and should perform high-level steps without focusing on details -
main()
should call the functions you create in your design, in the appropriate order, and with arguments that match parameters. Return values should be saved in main(), even if you don’t do anything with them yet. -
all functions should be stubbed out with parameters, a block comment, and a return statement. They don’t need to actually do anything yet, except possibly call other functions.
-
if your function is meant to return something, you should return a reasonable value of the appropriate type (e.g., return 0 for an int, or [1,2,3] for a list).
-
your design should have several functions. Each function should be function worthy (i.e., not a trivial task) and also demonstrate encapsulation (one clearly defined purpose).
-
the design should run without syntax errors (although it doesn’t play the full game yet)
-
Your goal for the design is to completely write main()
, such that it
won’t need to change much as you implement and test all of the other functions.
If you are confused about how the top-down design should look, please see the following examples of a design and full program for a simple guessing game program.
Tips
Note: some of these tips may not be needed until you implement the full program in the second week of this lab, but are included here to help you with the design.
Random library: choice(<sequence>)
The random library includes a choice
function that, given a sequence,
will randomly choose one of the items in the sequence. You should use the
choice
function to choose a random board at the beginning of the game.
Testing the game
Once you start working on the game implementation, being able to easily test it makes debugging easier and faster. Be sure you test your program after you do the bottom-up implementation of each function!
While you are testing your program, it is useful to have the same puzzle shown to you on each test. When selecting a board from those read in the file, instead of using choice
to choose a random board, always select the first board.
When you think you have things running, follow each example run shown above and be sure that you can get the same behavior from your program.
Extra Challenges
These will not affect your grade or gain you extra points. They are included just as extra challenges, if you are interested (and have already finished the entire game).
-
Each puzzle has at least one "pangram", which is a word that uses every tile at least once. In the real game, pangrams are worth 1 point per letter PLUS a bonus of 7 points. For example, given the board shown at the top of the lab writeup, the word "COLLECTIVE" would be worth 17 points: 1 point for each letter plus 7 bonus points for using each letter at least once. Notice than some words might be more than seven letters but not be pangrams. For example, "ELECTIVE" contains 8 letters but since it doesn’t use the letter "O", it isn’t a pangram.
-
When the game ends, show the player all of the legal words that they could have made and what their maximum score would have been.
-
Customize the print message at the end depending on their score. You can tie this into the previous challenge, too! You can even update this message as they play. For example, the real game has levels that are set based on the percentage of the maximum score you’ve earned: "Beginner", "Good Start", "Moving Up", "Good", "Solid", "Nice", "Great", "Amazing" and "Genius"
-
The already-guessed words (and the list of words printed out when the game ends) are not printed in a very pretty way. Try to make the output of these words show up nicely.
-
The real game allows players to randomize the order of the outer tiles. The center tile must stay fixed in place. Allow this option if the player types "MIX". You can use the
random.shuffle
function to help you shuffle the tiles. -
Allow the player to enter "TIP" to get a hint. You could choose a random word that the player hasn’t guessed yet and tell them the first letter or some other useful hint.
Answer the Questionnaire
Please edit
the Questions-06.txt
file in your cs21/labs/06
directory
and answer the questions in that file.
Once you’re done with that, run handin21
again.
Turning in your labs….
Remember to run handin21
to turn in your lab files!
You may run handin21
as many times as you want. Each time it will
turn in any new work. We recommend running handin21
after
you complete each program or after you complete significant
work on any one program.